Word Frequency Analysis Assignments in C++
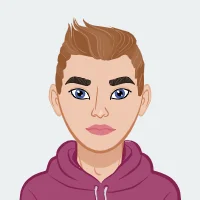
Word frequency analysis is a common task in text processing and analysis, often assigned to students to develop their understanding of fundamental computer science concepts. These assignments require you to read a text, count the occurrences of each word, and provide various statistics about the text, such as the most frequent word and the number of unique words. This type of assignment is essential in computer science education as it introduces key concepts like data structures, file handling, and basic algorithms. Implementing word frequency analysis in C++ involves using the Standard Template Library's unordered_map to efficiently store and count word occurrences, handling input and output streams to read text from files, and applying string manipulation techniques to sanitize and process words. In this blog, we'll guide you through the process of solving word frequency analysis assignments in C++, from understanding the problem requirements to planning your approach, choosing the right data structures, and implementing the necessary functions. If you need help with your C++ assignment, this blog will provide a comprehensive understanding that can be applied to similar assignments.
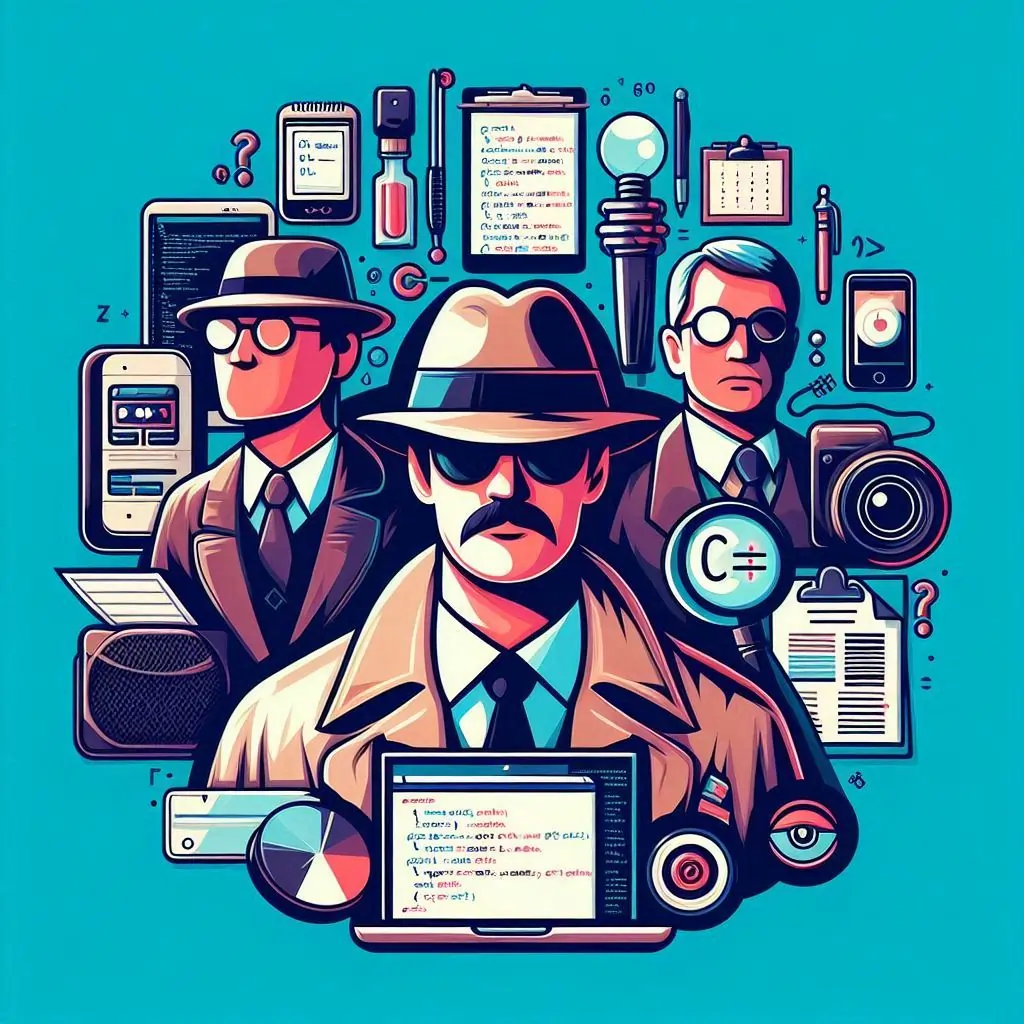
Understanding the Problem Requirements
Before diving into coding, it's crucial to thoroughly understand the assignment requirements. This initial step will save you time and prevent potential mistakes later on. Here's what you should focus on:
Identifying the Main Objectives
The primary goal of a word frequency analysis assignment is to analyze a text and count the occurrences of each word. Common tasks you might need to perform include:
- Reading the input text: This could be from a file or standard input.
- Sanitizing the input: Removing punctuation, converting words to lowercase, etc.
- Storing word frequencies: Using an appropriate data structure.
- Implementing specific functions: Such as finding the most frequent word or the number of unique words.
Recognizing Constraints and Rules
Every assignment will have specific rules and constraints that you need to follow. For example:
- Handling punctuation: Only remove leading and trailing punctuation but keep intra-word punctuation.
- Case sensitivity: Words should be case insensitive, meaning "Word" and "word" should be treated as the same word.
- Data structures: Often, you'll be required to use specific data structures like std::unordered_map in C++.
Reading the Provided Files
Assignments usually come with starter code and sample files. Make sure you understand what each file does. For example:
- main.cpp: Often orchestrates the flow of execution and tests intermediate results.
- WordFrequency.hpp/WordFrequency.cpp: These files will contain the core logic of your assignment, such as reading the text, sanitizing words, and storing word frequencies.
Planning Your Approach
Once you understand the problem requirements, the next step is to plan your approach. Having a clear plan will make the coding process smoother and more efficient. Here's how to structure your plan:
Breaking Down the Problem
Divide the problem into smaller, manageable tasks. For a word frequency analysis assignment, your plan might look like this:
Reading and Sanitizing Input
- Reading from a file or input stream: Use standard C++ input/output streams to read the text.
- Sanitizing words: Implement a function to remove punctuation and convert words to lowercase.
Storing and Counting Words
- Choosing the data structure: Use std::unordered_map to store words and their frequencies.
- Updating word counts: As you read and sanitize each word, update its count in the unordered_map.
Implementing Required Functions
- Counting unique words: Implement a function to return the number of unique words.
- Finding the most frequent word: Implement a function to find and return the word with the highest frequency.
- Other statistics: Implement additional functions as required, such as finding the size of the largest bucket in the hash table.
Choosing the Right Data Structure
For counting word frequencies, a hash table (implemented as std::unordered_map in C++) is ideal because it provides average-case O(1) time complexity for insert and lookup operations. The keys will be the words, and the values will be their respective counts.
Benefits of Using std::unordered_map
- Efficiency: Fast insertion and lookup operations.
- Ease of use: Simple interface for common operations like insertion, deletion, and lookup.
- Flexibility: Can handle a large number of entries efficiently.
Implementing the WordFrequency Class
With your plan in place, you can now start coding. In this section, we'll guide you through the implementation of the WordFrequency class, which will encapsulate all the logic for reading, sanitizing, and counting words.
Header File (WordFrequency.hpp)
First, define the class interface and declare necessary functions in the header file. This file will contain the class definition and function prototypes.
#ifndef WORDFREQUENCY_HPP
#define WORDFREQUENCY_HPP
#include
#include
#include
class WordFrequency {
public:
WordFrequency(std::istream& input = std::cin);
size_t numberOfWords() const;
size_t wordCount(const std::string& word) const;
std::string mostFrequentWord() const;
size_t maxBucketSize() const;
private:
std::unordered_map word_map;
static std::string sanitize(const std::string& word);
};
#endif // WORDFREQUENCY_HPP
Source File (WordFrequency.cpp)
Next, implement the member functions in the source file. This file will contain the actual code for reading input, sanitizing words, and counting frequencies.
#include "WordFrequency.hpp"
#include
#include
#include
WordFrequency::WordFrequency(std::istream& input) {
std::string word;
while (input >> word) {
word = sanitize(word);
if (!word.empty()) {
++word_map[word];
}
}
}
size_t WordFrequency::numberOfWords() const {
return word_map.size();
}
size_t WordFrequency::wordCount(const std::string& word) const {
auto it = word_map.find(sanitize(word));
return it != word_map.end() ? it->second : 0;
}
std::string WordFrequency::mostFrequentWord() const {
if (word_map.empty()) {
return "";
}
return std::max_element(word_map.begin(), word_map.end(),
[](const auto& a, const auto& b) {
return a.second < b.second;
})->first;
}
size_t WordFrequency::maxBucketSize() const {
size_t max_size = 0;
for (size_t i = 0; i < word_map.bucket_count(); ++i) {
max_size = std::max(max_size, word_map.bucket_size(i));
}
return max_size;
}
std::string WordFrequency::sanitize(const std::string& word) {
std::string result;
std::copy_if(word.begin(), word.end(), std::back_inserter(result),
[](char c) { return std::isalnum(c) || c == '-'; });
std::transform(result.begin(), result.end(), result.begin(), ::tolower);
return result;
}
Testing
To ensure your implementation is correct, create a main file to test your WordFrequency class. This file will set up the input and output, then call the class functions and verify the results.
#include "WordFrequency.hpp"
#include
int main() {
std::ifstream inputFile("The Legend of Sleepy Hollow by Washington Irving.txt");
WordFrequency wf(inputFile);
inputFile.close();
std::ofstream outputFile("output.txt");
outputFile << "Number of unique words: " << wf.numberOfWords() << std::endl;
outputFile << "Most frequent word: " << wf.mostFrequentWord() << std::endl;
outputFile << "Max bucket size: " << wf.maxBucketSize() << std::endl;
outputFile.close();
return 0;
}
Compiling and Running Your Program
With your code written and your tests in place, it's time to compile and run your program. This section will guide you through the compilation and execution process, ensuring that your program works correctly and efficiently.
Using a Build Script
To streamline the compilation process, you can use a build script. A build script can save you from typing long compile commands repeatedly. Here's an example of a simple build script using g++:
#!/bin/bash
g++ -o word_frequency main.cpp WordFrequency.cpp
./word_frequency
Command Line Compilation
Alternatively, you can compile your program directly from the command line. For example:
g++ -o word_frequency main.cpp WordFrequency.cpp
./word_frequency
Redirecting Input and Output
In many assignments, you will need to redirect input from a file and output to another file. This can be done using command line redirection:
./word_frequency < "The Legend of Sleepy Hollow by Washington Irving.txt" > output.txt
Conclusion
Solving word frequency analysis assignments in C++ involves understanding the problem requirements, planning your approach, choosing the right data structure, implementing the necessary functions, and thoroughly testing your program. By following these steps, you can effectively tackle a wide range of similar assignments. This structured approach not only helps in completing your tasks efficiently but also enhances your understanding of core programming concepts. Whether you're a student aiming to excel in your coursework or a professional looking to refine your skills, mastering these techniques will prove invaluable. Keep practicing and exploring different challenges to further solidify your knowledge and proficiency in C++ programming.