Simulating x86-45c Architecture for Programming Assignments
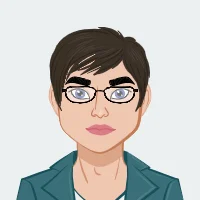
Programming assignments can sometimes feel overwhelming, especially when they involve complex topics like assembly language or custom instruction sets. However, with the right approach, you can tackle these assignments confidently and efficiently. Here’s a comprehensive guide to help you navigate through such tasks, ensuring you not only complete them but also understand the underlying concepts. This blog introduces the key components and characteristics of the architecture, providing a foundational understanding essential for completing your computer architecture assignment.
Identify Key Components
Once you have read the instructions, the next step is to identify the key components of the problem. This involves breaking down the problem into smaller parts and understanding how they fit together. Here’s how you can do this:
- List the Requirements: Write down the main requirements of the assignment. For example, in the provided assignment, the key requirements include handling various instructions (MOV, ADD, JMP, etc.) and managing the state of registers.
- Understand the Constraints: Identify any constraints or limitations specified in the problem statement. These could include restrictions on the libraries you can use or specific performance requirements.
- Visualize the Workflow: Try to visualize the workflow of the program. How will the instructions be processed? How will the state of the registers be updated? Visualizing the workflow can help you understand the problem better and plan your solution more effectively.
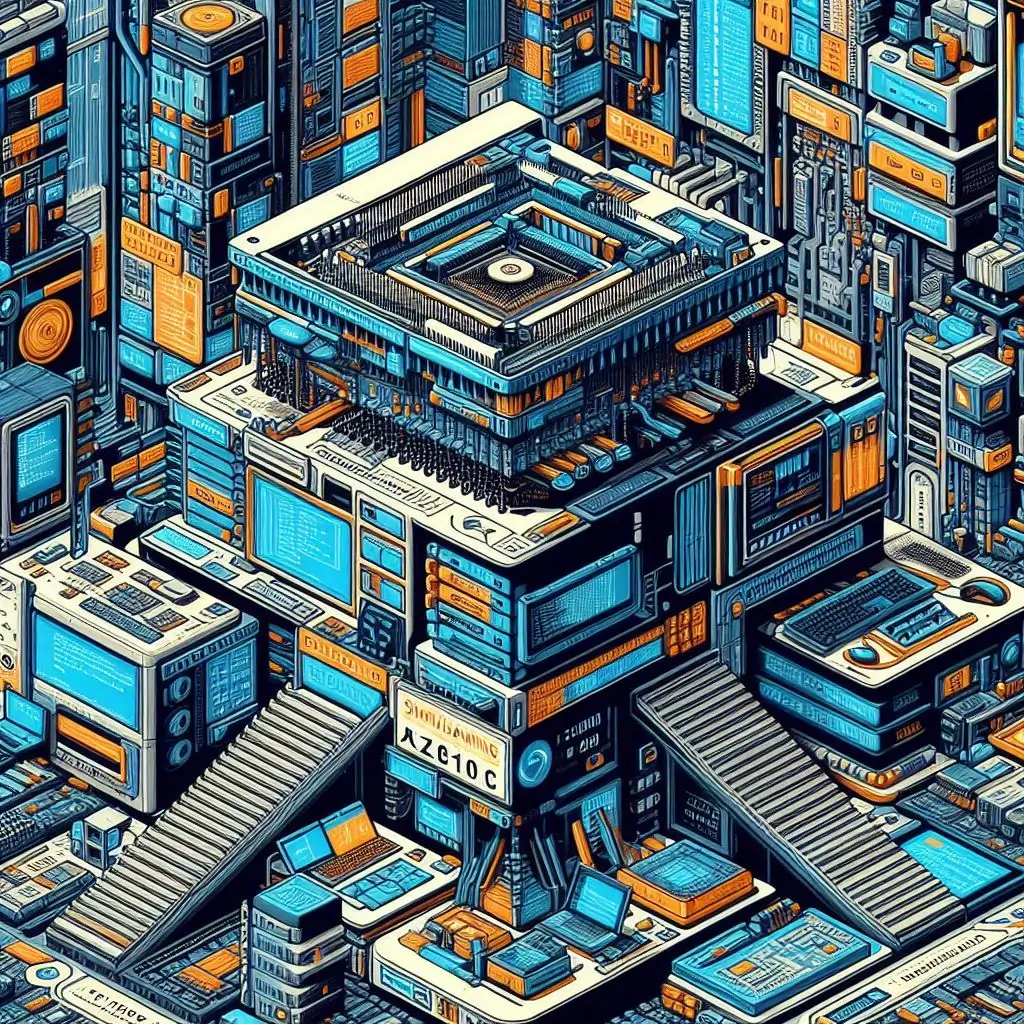
By identifying the key components, you can break down the problem into smaller, manageable parts, making it easier to tackle each part individually.
Plan Your Solution
With a clear understanding of the problem and its key components, you can now plan your solution. Planning is crucial to avoid getting stuck halfway through your coding. Here’s a step-by-step plan to help you get started:
- Outline the Classes and Functions: Identify the main classes and functions you’ll need. For instance, in the provided assignment, you’ll need a ProgramState class to manage the state of the registers and a function to execute each instruction.
- Define the Workflow: Determine the order in which tasks need to be executed. Typically, you would parse the input, execute the instructions, and then manage the program state.
- Create a Timeline: Break down the assignment into smaller tasks and create a timeline for completing each task. This will help you stay on track and ensure that you don’t leave everything until the last minute.
Planning your solution helps you organize your thoughts and approach the assignment methodically. It ensures that you don’t miss any important steps and makes the implementation process smoother.
Implementing Your Solution
When implementing your solution, break down the task into smaller, manageable parts. This not only makes the task less daunting but also helps you identify and fix issues more easily. Here’s how you can approach the implementation phase:
Setup the Environment
The first step in implementing your solution is to set up the environment. This involves initializing the necessary components and setting up the initial state of the program. Here’s how you can do this for the provided assignment:
- Initialize Registers: In the provided assignment, you need to initialize the registers to zero. This can be done in the constructor of the ProgramState class.
cpp
class ProgramState {
public:
ProgramState() {
// Initialize registers to zero
for(int i = 0; i < 16; i++) registers[i] = 0;
}
// More member functions and variables
private:
int registers[16];
};
- Set Up Input Handling: You will need to set up a mechanism to read and parse the input instructions. This can be done using standard input functions or by reading from a file.
- Define the Initial Program State: Set up any other initial state required for the program. This could include initializing variables, setting up data structures, or loading initial data.
Setting up the environment properly ensures that your program has a solid foundation to build upon. It helps you avoid issues related to uninitialized variables or incorrect initial states.
Implement Instruction Handling
Once the environment is set up, the next step is to implement the handling of instructions. This involves writing functions to handle each type of instruction (MOV, ADD, JMP, etc.). Here’s a possible approach:
- Define the Instruction Functions: Write functions for each type of instruction. These functions should take the necessary parameters (e.g., source and destination registers) and perform the required operations.
cpp
void handleMov(std::string dest, std::string src) {
// Convert dest and src to register indices or values
// Perform the MOV operation
}
void handleAdd(std::string src, std::string dest) {
// Perform the ADD operation
}
- Parse and Execute Instructions: Write a function to parse the input instructions and call the appropriate instruction functions. This function should loop through the instructions and update the program state accordingly.
cpp
void executeInstructions(std::vector
instructions) {
for (const std::string& instruction : instructions) {
// Parse the instruction and call the appropriate function
}
}
- Handle Edge Cases: Make sure to handle any edge cases or special scenarios. For example, you may need to check for invalid instructions or handle overflow/underflow situations.
Implementing instruction handling correctly is crucial to ensure that your program behaves as expected. It ensures that each instruction is executed properly and that the program state is updated accurately.
Testing Your Code
Testing is crucial to ensure your code works correctly. Here are some tips to help you test your code effectively:
- Unit Tests: Write unit tests for each function. This helps isolate and identify issues quickly. For example, you can write tests for each instruction function (MOV, ADD, JMP, etc.) to ensure they perform the expected operations.
- Edge Cases: Test your code with various edge cases, such as the minimum and maximum values for registers, and unusual sequences of instructions. This helps ensure that your code handles all possible scenarios.
- Comprehensive Tests: Create comprehensive test cases that simulate a full run of the program. This ensures that all parts of your code work together seamlessly. For example, you can write test cases that execute a series of instructions and verify the final state of the registers.
Testing your code thoroughly helps you identify and fix issues early on. It ensures that your code is robust and handles all possible scenarios correctly.
Debugging and Optimization
Even the best programmers need to debug their code. Debugging and optimizing your code is crucial to ensure it runs correctly and efficiently. Here’s how you can make the process easier:
Use Debugging Tools
Debugging tools are essential for identifying and fixing issues in your code. Here are some common debugging tools and techniques:
- Integrated Debuggers: Most modern IDEs come with integrated debugging tools. These tools allow you to set breakpoints, step through your code, and inspect variables. For example, Visual Studio and Eclipse both have powerful debugging tools that can help you identify issues quickly.
- Command Line Debuggers: If you prefer working from the command line, tools like gdb (GNU Debugger) can be very helpful. gdb allows you to set breakpoints, step through your code, and inspect variables just like an integrated debugger.
- Logging and Print Statements: Adding logging or print statements to your code can help you track the flow of execution and the state of variables. This can be particularly useful for identifying where things go wrong.
Using debugging tools effectively can save you a lot of time and frustration. It helps you pinpoint the exact location of issues and understand why they are occurring.
Optimize for Performance
Optimizing your code for performance is crucial, especially for assignments with time constraints. Here are some tips to help you optimize your code:
- Avoid Unnecessary Operations: Identify and remove any unnecessary operations in your code. For example, if you find that you are performing the same calculation multiple times, consider storing the result in a variable and reusing it.
- Use Efficient Data Structures: Choose the most efficient data structures for your needs. For example, if you need fast access to elements, consider using a hash table instead of a list.
- Profile Your Code: Use profiling tools to identify performance bottlenecks in your code. Profiling tools can help you see where your code is spending the most time and identify areas for improvement.
Optimizing your code ensures that it runs efficiently and meets any performance requirements specified in the assignment. It also helps improve the overall user experience by reducing the time taken to execute the program.
Refactor for Clarity
Refactoring your code for clarity is an important part of the debugging and optimization process. Clean, readable code is easier to debug and maintain. Here are some tips to help you refactor your code:
- Simplify Complex Logic: Break down complex logic into smaller, more manageable functions. This makes your code easier to understand and reduces the likelihood of errors.
- Use Meaningful Names: Use meaningful names for variables, functions, and classes. This makes your code more readable and easier to understand.
- Remove Redundant Code: Identify and remove any redundant code. This not only makes your code cleaner but also improves performance by reducing the number of operations.
Refactoring your code helps improve its readability and maintainability. It makes it easier for you and others to understand and work with your code in the future.
Final Touches
Before you submit your assignment, make sure to give it some final touches. This ensures that your code is polished and meets all the requirements. Here’s what you need to do:
Review the Requirements
Double-check that you’ve met all the requirements specified in the assignment. This includes both functional and non-functional requirements. Here are some tips:
- Go Through the Checklist: Create a checklist of all the requirements and go through it one by one. Make sure that your code meets each requirement.
- Verify Edge Cases: Ensure that your code handles all edge cases and special scenarios. This is particularly important for assignments with strict grading criteria.
- Test Again: Run your tests again to make sure that everything is working correctly. This includes unit tests, edge case tests, and comprehensive tests.
Reviewing the requirements ensures that you haven’t missed anything important. It helps you catch any last-minute issues and ensures that your code meets the expectations.
Optimize for Performance
Ensure your code runs efficiently, as some assignments have time constraints. Here are some additional tips for optimizing your code:
- Reduce Complexity: Simplify complex algorithms and reduce the overall complexity of your code. This can help improve performance and make your code easier to understand.
- Use Efficient Algorithms: Choose the most efficient algorithms for your needs. For example, if you need to sort a large dataset, consider using a quicksort or mergesort algorithm.
- Minimize Resource Usage: Optimize your code to minimize the usage of resources such as memory and CPU. This can help improve performance and reduce the likelihood of issues.
Optimizing your code ensures that it runs efficiently and meets any performance requirements specified in the assignment. It also helps improve the overall user experience by reducing the time taken to execute the program.
Document Your Code
Add comments to explain your logic. This is crucial for both your understanding and for anyone else who may read your code. Here are some tips for documenting your code effectively:
- Use Clear Comments: Write clear and concise comments that explain what each part of your code does. This helps others understand your logic and makes it easier to debug and maintain your code.
- Explain Complex Logic: Add comments to explain any complex logic or algorithms used in your code. This makes it easier for others to understand and follow your logic.
- Include Examples: Include examples in your comments to illustrate how your code works. This can be particularly helpful for complex functions or algorithms.
Documenting your code helps improve its readability and maintainability. It makes it easier for you and others to understand and work with your code in the future.
Submission Checklist
Before you submit your assignment, make sure to go through the following checklist:
- Code Review: Review your code to ensure it meets all the requirements and follows best practices.
- Testing: Ensure that all tests pass and that your code handles all edge cases and special scenarios.
- Documentation: Add comments to explain your logic and make your code easier to understand.
- Optimization: Optimize your code for performance and minimize resource usage.
- Submission Guidelines: Ensure that your submission meets any specific guidelines provided by your instructor.
Going through this checklist helps ensure that your code is polished and ready for submission. It helps you catch any last-minute issues and ensures that your code meets the expectations.
Conclusion
By following these steps, you can approach any programming assignment with confidence. Remember, the key is to break down the problem, plan your approach, and test thoroughly. With practice, you’ll become more adept at tackling even the most challenging assignments.