Conquer MIPS: Building Simple Games from Scratch
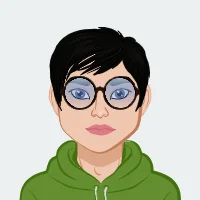
MIPS assembly language offers a unique challenge for students, particularly those tasked with creating interactive programs like games. While the intricacies of MIPS may initially seem overwhelming, mastering its fundamental concepts—such as memory management, control flow, input/output handling, and even understanding how it can empower you to tackle any MIPS assignment with confidence. This comprehensive guide is designed to help you understand and approach your programming assignments, using the development of a basic game as a framework. We will explore essential strategies, techniques, and best practices to ensure your success in any similar task
Understanding MIPS and Its Challenges
MIPS (Microprocessor without Interlocked Pipeline Stages) is a RISC (Reduced Instruction Set Computer) architecture that is commonly used in academic settings to teach assembly language programming. MIPS programming is known for its simplicity in instruction sets but also for its demands on the programmer to understand low-level operations. Successfully completing a MIPS programming assignment, particularly one that involves creating a game, requires a solid grasp of the architecture's constraints and capabilities.
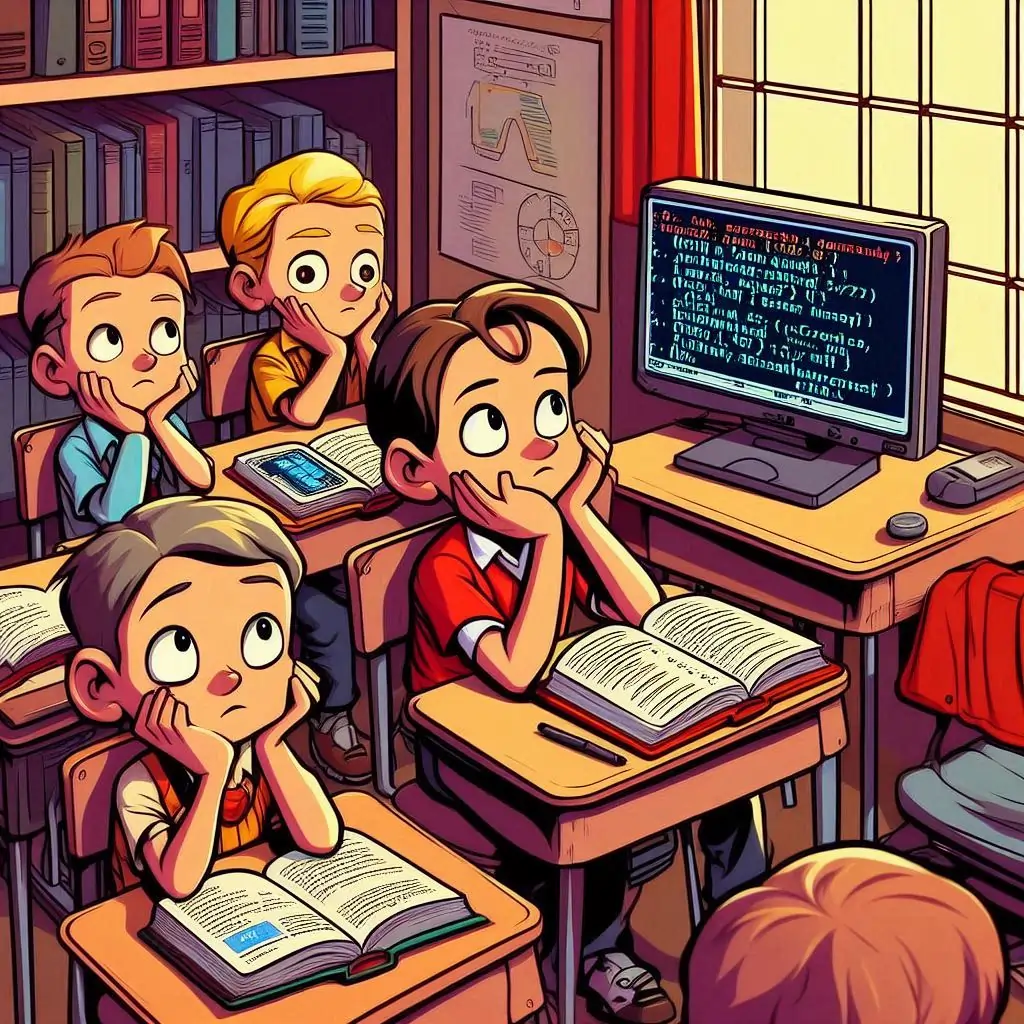
Decomposing the Assignment
The first step in tackling any MIPS assignment is to carefully read and deconstruct the assignment requirements. Breaking down the assignment into smaller tasks will help you manage the complexity and focus on one piece at a time.
Understanding the Requirements
Before you start writing your MIPS program, it's crucial to fully understand what is being asked. In the context of a game development assignment, you might be required to:
- Implement basic game mechanics such as movement, scoring, and collision detection.
- Use specific MIPS syscalls for memory allocation, random number generation, and output handling.
- Create an interactive environment where the player can control a character within a defined space.
- Manage user inputs from a keyboard and update the game state accordingly.
Taking the time to break down these requirements and envision how they translate into code is essential. This step will help you identify the core components of the game and how they interact with one another.
Identifying Key Components
In a MIPS game assignment, there are typically several key components you need to manage:
- Game Environment: This includes defining the layout of the game, such as the walls, the player’s starting position, and the rewards.
- Player Interaction: Handling user input to move the player within the game environment.
- Score Management: Keeping track of the player's score and determining when the game ends.
Understanding how these components fit together will help you create a clear plan of action. For instance, you need to decide how to store and update the player’s position in memory, how to detect and handle collisions with walls, and how to update the display.
Planning Your Approach
Once you have a clear understanding of the assignment and its requirements, the next step is to plan your approach. This involves writing pseudocode or creating a flowchart to map out the sequence of operations and control flow in your program. Pseudocode is particularly useful in MIPS programming because it allows you to focus on the logic of the program without getting bogged down in the syntax.
Implementing the Game in MIPS
After planning, it’s time to dive into the actual coding. Implementing a game in MIPS requires careful attention to detail, especially given the architecture's limitations. Below, we’ll walk through the critical steps involved in building a game in MIPS, from setting up the environment to handling user inputs and managing game states.
Setting Up the Game Environment
The first major task in your MIPS program is to set up the game environment. This involves defining the layout of the game, including the walls, the player’s starting position, and the rewards. In MIPS, this can be done using arrays to represent the grid of the game environment.
Defining the Grid
Start by defining a 2D array that represents the game grid. Each cell in the grid can be used to store information about what is located at that position—whether it's empty, contains a wall, the player, or a reward.
.data
grid: .space 49 # 7x7 grid (49 bytes)
Here, grid is an array that represents a 7x7 game environment. Each byte in this array corresponds to a cell in the grid, which can hold a specific value indicating what is in that cell.
Placing the Player and Rewards
Next, you need to initialize the player's starting position and place rewards randomly within the grid. Use the sbrk syscall to allocate memory dynamically if needed, and the random syscall to place rewards in random locations.
li $v0, 42 # Random integer in range syscall
li $a0, 49 # Range for random number (0-48)
syscall
move $t0, $v0 # Store random position in $t0
sb $t1, grid($t0) # Place reward in grid at random position
This code snippet generates a random position in the grid and places a reward at that location.
Handling Player Movement
With the environment set up, the next step is to allow the player to move within the game. This involves reading keyboard inputs and updating the player’s position accordingly.
Reading Keyboard Inputs
Use the MARS Keyboard and Display MMIO Simulator to read user inputs. The WASD keys can be used for movement, and you will need to translate these keypresses into changes in the player's position.
li $v0, 51 # Read character from keyboard
syscall
move $t1, $v0 # Store keypress in $t1
# Check for 'W' key press (ASCII 119)
li $t2, 119
beq $t1, $t2, move_up
Updating Player Position
After determining which key was pressed, update the player’s position in the grid. Be sure to check for collisions with walls and ensure the player doesn’t move out of bounds.
move_up:
sub $t3, $t0, 7 # Move up by decreasing row index
sb $zero, grid($t0) # Clear old position
sb $t4, grid($t3) # Place player at new position
This code moves the player up one row in the grid if the 'W' key is pressed.
Managing Game State
The final major component of your MIPS game is managing the game state, including score updates and determining when the game ends.
Updating the Score
Each time the player collects a reward, update the score by 5 points and display it at the top of the screen.
add $t5, $t5, 5 # Increment score by 5
li $v0, 1 # Print integer syscall
move $a0, $t5 # Print current score
syscall
Ending the Game
The game should end if the player collects 100 points or collides with a wall. When the game ends, clear the display and show a 'GAME OVER' message along with the final score.
li $v0, 10 # Exit syscall to end game
syscall
Extending the Game
After completing the basic game, you may be required to extend its functionality. This could involve adding features such as speed, additional obstacles, or more complex game mechanics. Let’s explore how to approach these extensions.
Adding Player Speed
One possible extension is to give the player a speed attribute, which causes the player to continuously move in one direction until they change direction.
Implementing Continuous Movement
To implement continuous movement, modify your code to keep the player moving after each keypress until another key is pressed.
li $t6, 1 # Speed variable
move $t7, $t6 # Set player speed
Handling Direction Changes
Allow the player to change direction by pressing a different key. Adjust the player's movement logic to update the direction instead of stopping the player.
move_right:
add $t0, $t0, 1 # Move right by increasing column index
Adding Obstacles
Another extension could involve adding obstacles that the player must avoid. This would require you to add more elements to the grid and modify the collision detection logic.
Placing Obstacles
Randomly place obstacles in the grid similar to how rewards are placed. Ensure that these obstacles block the player’s movement.
li $t8, 35 # ASCII for '#'
sb $t8, grid($t9) # Place obstacle in grid
Collision Detection
Update the movement logic to check for collisions with obstacles and end the game if the player hits one.
beq $t1, $t8, game_over # End game on collision with obstacle
Enhancing Game Complexity
Finally, you might consider adding more complex game mechanics, such as multiple levels, time limits, or power-ups.
Implementing Multiple Levels
Add functionality to load new game environments when the player reaches certain scores or completes a level.
load_level:
li $t9, 0 # Reset grid for new level
sb $zero, grid($t9) # Clear previous level
Common Pitfalls and Debugging Tips
MIPS programming can be challenging, especially for beginners. Below are some common pitfalls and tips to help you avoid them.
Memory Management Errors
One of the most common mistakes in MIPS programming is incorrect memory management. Always ensure that you allocate and deallocate memory correctly, especially when using dynamic memory for game elements.
Debugging Memory Issues
Use the MARS debugger to step through your code and inspect the contents of memory at each step. This can help you identify where your program is going wrong.
li $v0, 9 # Dynamic memory allocation
li $a0, 49 # Allocate 49 bytes
syscall
move $t0, $v0 # Store address of allocated memory
Incorrect Syscall Usage
Another common issue is the incorrect use of syscalls. Each syscall in MIPS serves a specific purpose, and using the wrong one can lead to unexpected behavior.
Verifying Syscalls
Always refer to the MIPS syscall reference to ensure you’re using the correct syscall for the task at hand. Test your syscalls in isolation to confirm they work as expected.
li $v0, 4 # Print string syscall
la $a0, string # Load address of string
syscall
Debugging Control Flow Issues
Control flow errors, such as infinite loops or incorrect branching, can be particularly difficult to debug in MIPS.
Using the MARS Simulator
Leverage the MARS simulator’s step-through feature to carefully trace the execution of your program and identify where control flow deviates from your plan.
beq $t0, $t1, loop # Branch if equal to create a loop
Conclusion:
Mastering MIPS programming, especially for complex tasks like game development, requires patience, practice, and a deep understanding of low-level programming concepts. By breaking down your assignment into manageable tasks, planning your approach, and carefully implementing and testing each part of your program, you can successfully complete any MIPS assignment. Always remember to manage your memory efficiently, use syscalls correctly, and take advantage of debugging tools like the MARS simulator to troubleshoot issues as they arise.
With these strategies and best practices, you'll not only be able to tackle your MIPS programming assignments but also develop a strong foundation in assembly language programming that will serve you well in more advanced computing courses.