Developing Dice Roll and Point System Games using C++
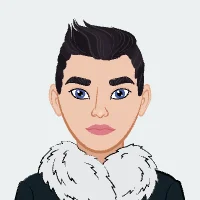
Programming assignments that involve game logic can be an exciting way to apply and enhance your coding skills. They typically require a combination of understanding control flow, implementing logic based on user input, and managing conditions and loops effectively. This blog post will guide you through a systematic approach to tackling such assignments, specifically focusing on solving C++ assignments, using the example of a dice game with a point system.
Understanding the Assignment
The first step in successfully completing any programming assignment is to thoroughly understand the requirements. Let’s break down the provided example of a dice game assignment:
1. Initial Setup:
- The game starts with a user score of 500 points.
- The user inputs the values of two dice.
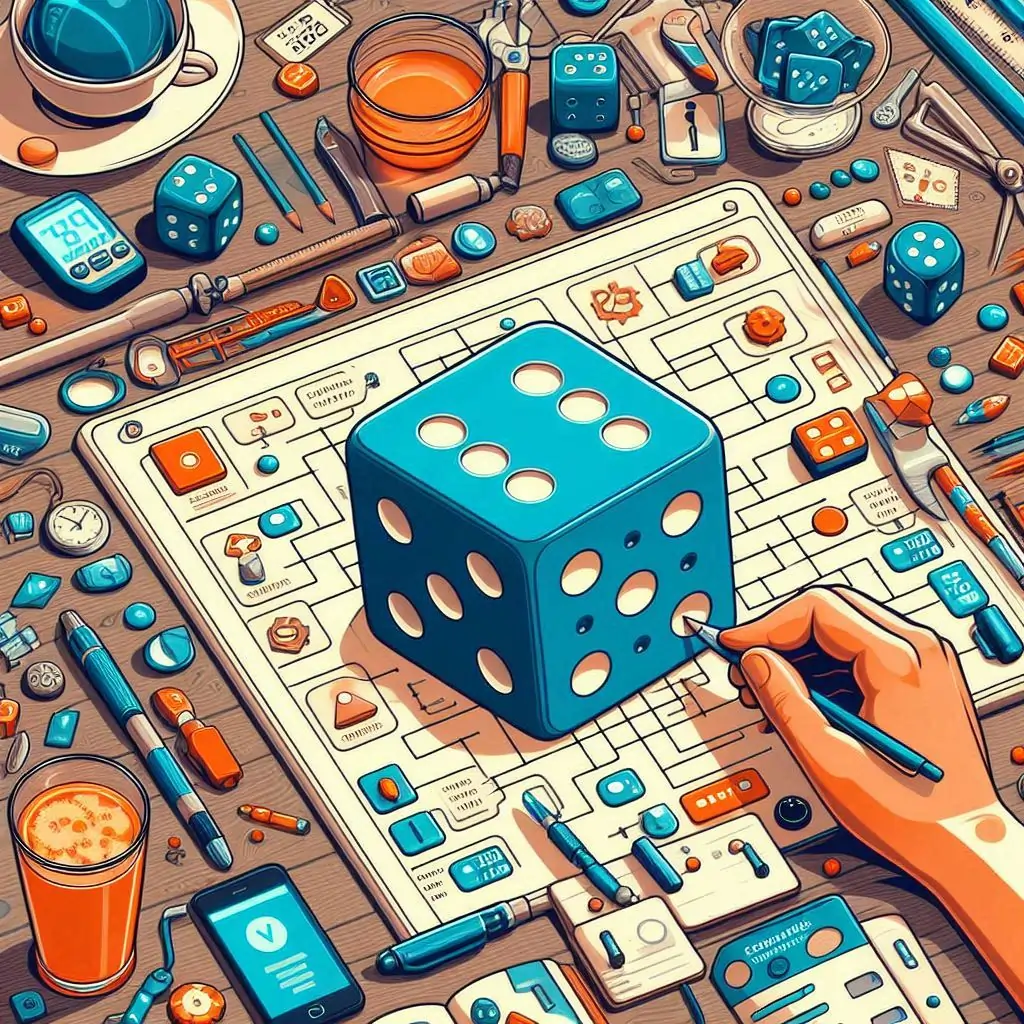
2. Scoring Criteria:
- If the dice roll is a double, the user scores 200 points.
- If the dice total equals X (9) or Y (11), the user scores 100 points.
- Otherwise, the user loses 50 points.
- The game ends if the score exceeds 1000 or drops below zero.
3. Game End Conditions:
- Output messages indicating win/lose status and points gained/lost.
- Allow the user to restart the game or exit.
4. User Instructions and Exit Option:
- Provide clear instructions at the beginning.
- Include an option for the user to exit the game, displaying their final score.
Initial Setup
Understanding the Game Rules
Before diving into coding, take time to clearly understand the game rules. The user starts with 500 points, and the game involves rolling two dice. Depending on the outcome of the dice rolls, the user gains or loses points based on specific criteria. The game continues until the score exceeds 1000 or drops below zero.
Designing the User Input Flow
Designing how the user interacts with the game is crucial. The user must input the values of the two dice manually. Ensure that your program prompts the user clearly and handles the input correctly, including any necessary validation to prevent invalid inputs.
Setting Up the Initial Score
Initialize the user’s score to 500 points at the start of the game. This setup will serve as the basis for the score calculations that will follow each dice roll.
Scoring Criteria
Implementing the Doubles Condition
One of the main scoring criteria is rolling doubles. If the two dice show the same value, the user gains 200 points. This condition can be checked by comparing the values of the two dice.
Handling Specific Totals
If the sum of the two dice equals 9 or 11, the user gains 100 points. This condition requires adding the values of the two dice and checking if the sum matches either of these totals.
Managing Points Deduction
If neither of the above conditions is met, the user loses 50 points. This ensures that there is always a consequence for each roll, keeping the game engaging and challenging.
Game End Conditions
Checking for Score Limits
After each roll, check if the score has exceeded 1000 or dropped below zero. If either condition is met, the game ends. This check ensures that the game has a clear end point based on the user’s performance.
Outputting Results
Provide feedback to the user after each roll. This feedback should include whether they won or lost points and their new total score. Clear and immediate feedback enhances the user experience.
Restarting or Exiting the Game
At the end of the game, give the user the option to restart or exit. If they choose to exit, display their final score and thank them for playing. This choice allows users to play multiple rounds without restarting the program.
Implementing the Solution
Now that we have a clear understanding of the assignment, let’s walk through the implementation step-by-step. We’ll use C++ for this example, but the principles apply to any programming language.
Initial Setup in Code
Setting Up the Environment
First, include the necessary headers and set up the main function. Initialize the user’s score and create a loop to keep the game running until a termination condition is met.
#include
using namespace std;
void displayInstructions() {
cout << "Welcome to the Dice Game!\n";
cout << "Rules:\n";
cout << "1. You start with 500 points.\n";
cout << "2. Roll two dice by entering their values.\n";
cout << "3. If you roll doubles, you score 200 points.\n";
cout << "4. If the total of the dice is 9 or 11, you score 100 points.\n";
cout << "5. Otherwise, you lose 50 points.\n";
cout << "6. The game ends if your score goes above 1000 or below 0.\n";
cout << "7. You can exit the game at any time by entering '0' for both dice.\n";
cout << "Let's start the game!\n\n";
}
Handling User Input
Prompt the user to input values for the two dice and handle their input. Ensure input validation to handle incorrect inputs gracefully.
bool playGame() {
int score = 500;
int dice1, dice2;
while (score > 0 && score <= 1000) {
cout << "Enter the value for dice 1: ";
cin >> dice1;
cout << "Enter the value for dice 2: ";
cin >> dice2;
if (dice1 == 0 && dice2 == 0) {
cout << "You chose to exit the game. You finished with " << score << " points.\n";
return false;
}
cout << "Dice 1: " << dice1 << ", Dice 2: " << dice2 << endl;
// Game logic goes here
cout << "Your new total score is: " << score << " points.\n";
}
return true;
}
Displaying Instructions
At the beginning of the game, display clear instructions to the user. This helps ensure they understand the rules and how to play.
int main() {
displayInstructions();
bool playAgain = true;
while (playAgain) {
playAgain = playGame();
if (playAgain) {
char choice;
cout << "Would you like to play again? (y/n): ";
cin >> choice;
playAgain = (choice == 'y' || choice == 'Y');
}
}
cout << "Good bye!\n";
return 0;
}
Implementing Game Logic
Checking for Doubles
Within the game loop, check if the values of the two dice are equal. If they are, add 200 points to the user’s score and display a winning message.
if (dice1 == dice2) {
score += 200;
cout << "YOU ARE A WINNER! You scored 200 points.\n";
}
Checking for Specific Totals
Next, check if the sum of the two dice equals 9 or 11. If so, add 100 points to the user’s score and display a winning message.
else if ((dice1 + dice2) == 9 || (dice1 + dice2) == 11) {
score += 100;
cout << "YOU ARE A WINNER! You scored 100 points.\n";
}
Handling Points Deduction
If neither of the above conditions is met, subtract 50 points from the user’s score and display a losing message.
else {
score -= 50;
cout << "SORRY, YOU LOSE. You lost 50 points.\n";
}
Managing Game End Conditions
Checking Score Limits
After updating the score, check if it has exceeded 1000 or dropped below zero. If so, end the game and display a message indicating that the game is over.
if (score > 1000) {
cout << "Congratulations! Your score exceeded 1000. Game Over.\n";
return false;
} else if (score < 0) {
cout << "Your score fell below 0. Game Over.\n";
return false;
}
Outputting Results
After each roll, display the outcome and the new total score to the user. This provides immediate feedback and keeps the user engaged.
cout << "Your new total score is: " << score << " points.\n";
Restarting or Exiting the Game
At the end of the game, prompt the user to decide if they want to play again or exit. If they choose to exit, display their final score and a farewell message.
char choice;
cout << "Would you like to play again? (y/n): ";
cin >> choice;
playAgain = (choice == 'y' || choice == 'Y');
Tips for Success
Successfully completing programming assignments, especially those involving game logic, requires a combination of good planning, clear coding practices, and thorough testing. Here are some tips to help you succeed:
Break Down the Problem
Divide and Conquer
Divide the assignment into manageable parts: initialization, user input, game logic, and termination conditions. This approach, often referred to as "divide and conquer," makes the task less overwhelming and helps you focus on one aspect at a time.
Step-by-Step Implementation
Implement your solution step-by-step. Start with the initial setup, then handle user input, followed by implementing game logic and finally managing the end conditions. This step-by-step approach ensures that each part of your program works correctly before moving on to the next.
Testing Each Component
Test each part of your program as you implement it. This helps identify and fix issues early, making the debugging process easier.
Clear User Instructions
Providing Detailed Instructions
Always provide clear and detailed instructions to the user. This improves the user experience and helps them understand how to interact with your program. Instructions should be displayed at the beginning of the game and should cover all the rules and possible inputs.
User Feedback
Provide immediate feedback after each user action. Whether they win points, lose points, or make an invalid input, clear feedback helps keep the user engaged and informed.
Error Handling
Implement error handling to manage invalid inputs gracefully. For example, if the user enters a non-numeric value or a value outside the expected range, display an appropriate error message and prompt them to try again.
Input Validation
Ensuring Correct Inputs
Ensure that user inputs are validated to avoid crashes and unexpected behavior. Check that the input values are within the expected range (e.g., dice values between 1 and 6) and handle invalid inputs appropriately.
Prompting for Re-entry
If the user enters an invalid value, prompt them to re-enter the correct value. This helps prevent errors and keeps the game running smoothly.
Using Loops for Validation
Use loops to continuously prompt the user until a valid input is provided. This ensures that the program only proceeds with valid data.
Modular Code
Using Functions
Use functions to modularize your code. This makes your program easier to understand and maintain. Each function should have a single responsibility, such as handling user input, checking game conditions, or updating the score.
Function Naming
Use descriptive names for your functions to indicate their purpose. This improves code readability and helps others understand your code more easily.
Reusability
Design your functions to be reusable. For example, a function that handles input validation can be used in multiple places in your program.
Test Thoroughly
Testing with Different Inputs
Test your game with different inputs to ensure all conditions are handled correctly. Try different combinations of dice values to check if the scoring logic works as expected.
Boundary Testing
Test the boundaries of your game logic. For example, check what happens when the score is exactly 1000 or when the user is about to lose all their points. This helps ensure that your program handles edge cases correctly.
User Testing
Have others test your game. They might use it in ways you didn’t anticipate and help you find bugs or areas for improvement.
Conclusion
Programming assignments involving game logic can be a rewarding way to apply your coding skills and learn new concepts. By understanding the requirements, breaking down the problem, implementing the solution step-by-step, and thoroughly testing your program, you can successfully complete such assignments and enhance your programming abilities. Remember, practice and patience are key to mastering programming skills. Each assignment is an opportunity to learn and grow. Good luck with your programming journey, and have fun creating engaging games and applications!