Solving Digital Design Assignments Effectively
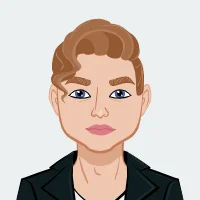
These assignments can be challenging, but they are crucial for developing a deep understanding of digital systems and microprocessor functionality. This guide will walk you through the process of solving such assignments, offering practical advice and insights that will help you excel. By the end, you'll be equipped with the knowledge and skills to approach similar tasks with confidence. The first step involves thoroughly understanding the assignment requirements and the expected outcomes. You need to grasp the initial instruction execution, memory address management, and instruction decoding and execution. Once you have a clear understanding, you can proceed to design the solution, starting with a high-level overview and then focusing on individual components like the control unit and arithmetic logic unit. Implementing the design in Verilog requires creating modules for each component and ensuring they work together seamlessly. Testing and simulation are vital to verify the design's functionality and to debug any issues. Finally, documenting your work comprehensively will help you review your approach and improve future projects. This approach not only aids in solving your Verilog assignment successfully but also builds a strong foundation for you so that you can complete your programming assignment in a better manner.
Understanding the Assignment Requirements
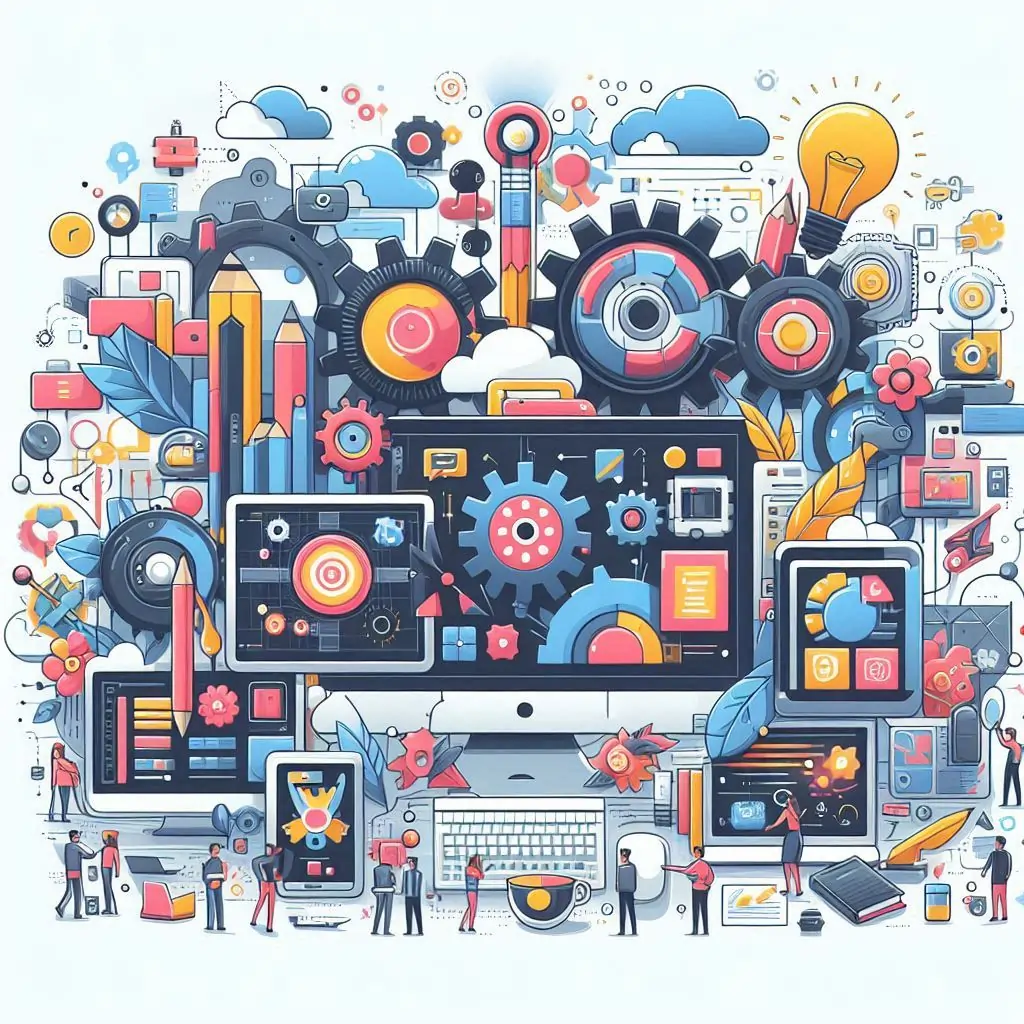
Before you start coding or designing, it's critical to thoroughly understand the assignment requirements. This step lays the foundation for your entire project, ensuring that you address all necessary components and meet the expectations set out by your instructor.
Initial Instruction Execution
The first step in any digital design assignment is to understand how the program starts and which instructions are executed initially. In many cases, the Program Counter (PC) starts at address 0x00. This is where the first instruction is located and from where the execution begins.
- Identify the First Instruction: In your assignment, the first instruction is often a branch instruction (e.g., BRA START_ADDRESS). This instruction tells the processor to jump to a specific memory address where the main code resides. Understanding how the PC is set up and how it fetches the first instruction is crucial for the successful execution of your program.
- Branching Mechanics: The branch instruction changes the flow of execution. Instead of continuing sequentially, the PC is updated to the specified address. This is essential for starting your main program logic from a defined point in memory.
- Importance of Initial Setup: Properly setting up the initial instruction ensures that your program starts correctly and that subsequent instructions are executed in the right order. This step often involves setting up memory and loading instructions into the correct locations.
Memory Address Management
Digital design assignments typically involve manipulating data and instructions stored in memory. Understanding how to manage memory addresses effectively is key to ensuring that your program functions correctly.
- Organizing Instructions and Data: Plan how your instructions and data will be laid out in memory. This organization affects how efficiently your program runs and how easily you can access and manipulate data. For instance, you might have instructions starting at one address range and data stored in another.
- Addressing Modes: Learn about different addressing modes such as immediate, direct, and indirect. Each mode offers a unique way to access memory, and understanding them will help you choose the most efficient way to handle data in your program.
- Example Layout: Suppose your main code starts at address 0x28, as specified by the branch instruction. From there, you might have data stored starting at address 0xA0. Clearly defining these regions helps avoid conflicts and ensures smooth program execution.
Instruction Decoding and Execution
The heart of any digital design project is the instruction set and how these instructions are decoded and executed by the processor. This step involves translating your high-level design into specific actions performed by the hardware.
- Define Your Instruction Set Architecture (ISA): Your ISA includes all the instructions that your processor can execute, such as MOV, ADD, LDR, and STR. Clearly define each instruction's operation, including what it does and how it interacts with registers and memory.
- Decoding Logic: Design logic to decode each instruction. This involves interpreting the binary code of each instruction to determine what action to take. For example, the instruction MOVH R1, 0x00 would set the high bits of register R1 to 0x00.
- Execution Flow: Implement the control logic that dictates the flow of execution. This involves managing the PC, handling jumps and branches, and ensuring that instructions are executed in the correct sequence.
Designing the Solution
Once you understand the requirements, the next step is to design a solution that meets these requirements effectively. This involves creating a high-level design, planning the memory map, and designing the control unit.
High-Level Design
Start by sketching out a high-level design of your program. This will help you visualize how the different components interact and how data flows through the system.
Flow Diagram
Create a flow diagram that outlines the sequence of operations in your program. This diagram should include:
- Initial Setup: The steps involved in setting up the initial conditions, such as loading data into registers and setting the PC to the starting address.
- Main Loop: The core loop of your program, including instruction fetch, decode, and execute cycles.
- End Conditions: The conditions under which the program will terminate or loop back to the start.
Component Interaction
Define how different components of your system, such as the ALU, registers, and memory, will interact with each other. This includes:
- Data Flow: How data moves between registers, memory, and the ALU.
- Control Signals: The signals that control the operation of each component, such as read/write signals for memory and control signals for the ALU.
Memory Map
Plan the memory layout, specifying where instructions and data will be stored. This helps in organizing your program and avoiding conflicts.
- Instruction Memory: Define the range of addresses that will store your instructions.
- Data Memory: Specify the range for data storage, ensuring that there is enough space for all the data your program will manipulate.
Designing the Control Unit
The control unit is responsible for managing the execution of instructions and coordinating the actions of the other components.
Instruction Fetch and Decode
Design logic to fetch instructions from memory and decode them to determine the appropriate action.
- Fetch Logic: The fetch logic reads the instruction from the address pointed to by the PC and increments the PC to point to the next instruction.
- Decode Logic: The decode logic interprets the fetched instruction and generates the control signals needed to execute it.
Execution Control
Implement the control logic that executes the instructions, managing data flow and coordinating the actions of the ALU, memory, and registers.
- ALU Operations: Define the operations that the ALU will perform, such as addition, subtraction, and logical operations.
- Memory Access: Design the logic for reading from and writing to memory, including handling different addressing modes.
Implementing in Verilog HDL
With your design in hand, it's time to implement it in Verilog HDL. This involves writing code for each component and ensuring that they work together as intended.
Module Creation
Break down your design into smaller modules, each responsible for a specific function. This modular approach makes your code easier to manage and debug.
Control Unit Module
Create a module for the control unit that handles instruction fetch, decode, and execution.
module control_unit(
input clk, reset,
output reg [15:0] pc, // Program Counter
output reg [15:0] instruction // Fetched Instruction
);
reg [15:0] memory [0:255]; // Memory Array
initial begin
// Initialize memory with instructions
memory[0] = 16'hXXXX; // Example: BRA 0x28
memory[40] = 16'hXXXX; // Example: MOVH R1, 0x00
// Load more instructions as needed
end
always @(posedge clk or posedge reset) begin
if (reset)
pc <= 0; // Reset PC
else
pc <= pc + 1; // Increment PC
end
always @(pc) begin
instruction = memory[pc];
end
endmodule
ALU Module
Design a module for the ALU that performs arithmetic and logical operations.
module alu(
input [15:0] operand1, operand2,
input [3:0] operation, // Define operations like ADD, SUB
output reg [15:0] result
);
always @(*) begin
case (operation)
4'b0000: result = operand1 + operand2; // ADD
4'b0001: result = operand1 - operand2; // SUB
// Define more operations as needed
endcase
end
endmodule
Memory Module
Create a module for memory that supports reading and writing data.
module memory(
input clk,
input [7:0] address, // Memory address
input [15:0] write_data,
input write_enable,
output reg [15:0] read_data
);
reg [15:0] mem_array [0:255]; // Memory array
always @(posedge clk) begin
if (write_enable)
mem_array[address] <= write_data; // Write data
end
always @(address) begin
read_data = mem_array[address]; // Read data
end
endmodule
Connecting the Modules
Integrate the modules into a cohesive system. Ensure that each module communicates correctly with the others and that the data flows smoothly through the system.
module system(
input clk, reset,
output [15:0] final_result // Example output
);
wire [15:0] pc, instruction;
wire [15:0] alu_result;
wire [15:0] read_data;
control_unit CU(.clk(clk), .reset(reset), .pc(pc), .instruction(instruction));
alu ALU(.operand1(16'h0000), .operand2(16'h0001), .operation(4'b0000), .result(alu_result));
memory MEM(.clk(clk), .address(pc[7:0]), .write_data(alu_result), .write_enable(1'b1), .read_data(read_data));
assign final_result = read_data;
endmodule
Testing and Simulation
Testing and simulation are crucial steps to ensure that your design works correctly. Use simulation tools to verify the functionality of each module and the entire system.
Simulation Tools
Choose simulation tools that allow you to test your Verilog code. Popular options include ModelSim, Xilinx Vivado, and Cadence.
- ModelSim: A widely used tool that offers powerful simulation capabilities and a user-friendly interface.
- Xilinx Vivado: Ideal for designs targeting Xilinx FPGAs, providing comprehensive simulation and debugging tools.
- Cadence: Offers advanced simulation and verification features, suitable for complex designs.
Creating Test Benches
Write test benches to simulate the behavior of your modules under various conditions. This helps identify bugs and verify that your design meets the requirements.
Example Test Bench for Control Unit
module test_bench;
reg clk, reset;
wire [15:0] pc, instruction;
control_unit CU(.clk(clk), .reset(reset), .pc(pc), .instruction(instruction));
initial begin
clk = 0;
reset = 1;
#10 reset = 0; // Release reset
#100 $stop; // Stop simulation after 100 time units
end
always #5 clk = ~clk; // Generate clock signal
initial begin
$monitor("Time: %0d, PC: %h, Instruction: %h", $time, pc, instruction);
end
endmodule
Analyzing Simulation Results
Analyze the simulation results to ensure that your design functions as expected. Look for discrepancies between expected and actual outputs, and debug any issues that arise.
- Expected Output: Define what you expect the output to be for each test case.
- Actual Output: Compare the actual output from the simulation to the expected output.
- Debugging: If there are discrepancies, debug your code by tracing the signals and understanding where the logic went wrong.
Documenting Your Work
Once your design is complete and tested, document your work thoroughly. This includes detailing your design decisions, simulation results, and any issues you encountered.
Design Documentation
Write a detailed report explaining your design choices and how you implemented each component.
- Component Descriptions: Describe each module and its functionality.
- Design Rationale: Explain why you chose specific design approaches and how they meet the assignment requirements.
Simulation Results
Include screenshots or logs of your simulation results, highlighting key tests and outcomes.
- Test Cases: List the test cases you used and their purposes.
- Results: Provide a summary of the results for each test case, noting any issues and how you resolved them.
Group Contributions
If you worked in a group, document the contributions of each member and how you collaborated on the project.
- Roles and Responsibilities: List each member and their role in the project.
- Collaboration Process: Describe how you worked together, including any challenges and how you overcame them.
Conclusion
By following these steps, you can effectively approach and solve digital design assignments involving Verilog HDL and assembly language. Remember, the key to success is a thorough understanding of the requirements, a well-structured design, meticulous implementation, and comprehensive testing. With practice, you'll develop the skills needed to tackle even the most complex digital design challenges.For more help with your programming assignments, visit Programming Assignment Helper. Our experts are here to guide you through your next project, ensuring you achieve your academic goals with confidence!