Simulating CPU Instruction Traces and Cache Behavior
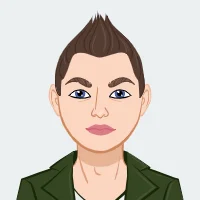
Programming assignments can be daunting, especially when they involve multiple components and advanced concepts. This comprehensive guide will provide you with a structured approach to solving such assignments efficiently. This comprehensive guide will provide you with a structured approach to solving such assignments efficiently, including help with C assignments. We will use an example of an assignment that involves generating trace files, manipulating binary data, and simulating cache behavior. However, the principles outlined here can be applied to a wide range of similar programming tasks.
Understanding the Assignment Requirements
Before diving into coding, it's crucial to thoroughly understand the assignment requirements. This section will guide you through the steps needed to break down the assignment into manageable tasks and identify the main objectives.
Breaking Down the Assignment
Understanding the assignment involves breaking it down into smaller, manageable parts. Let's consider the provided assignment as an example, which involves three main components:
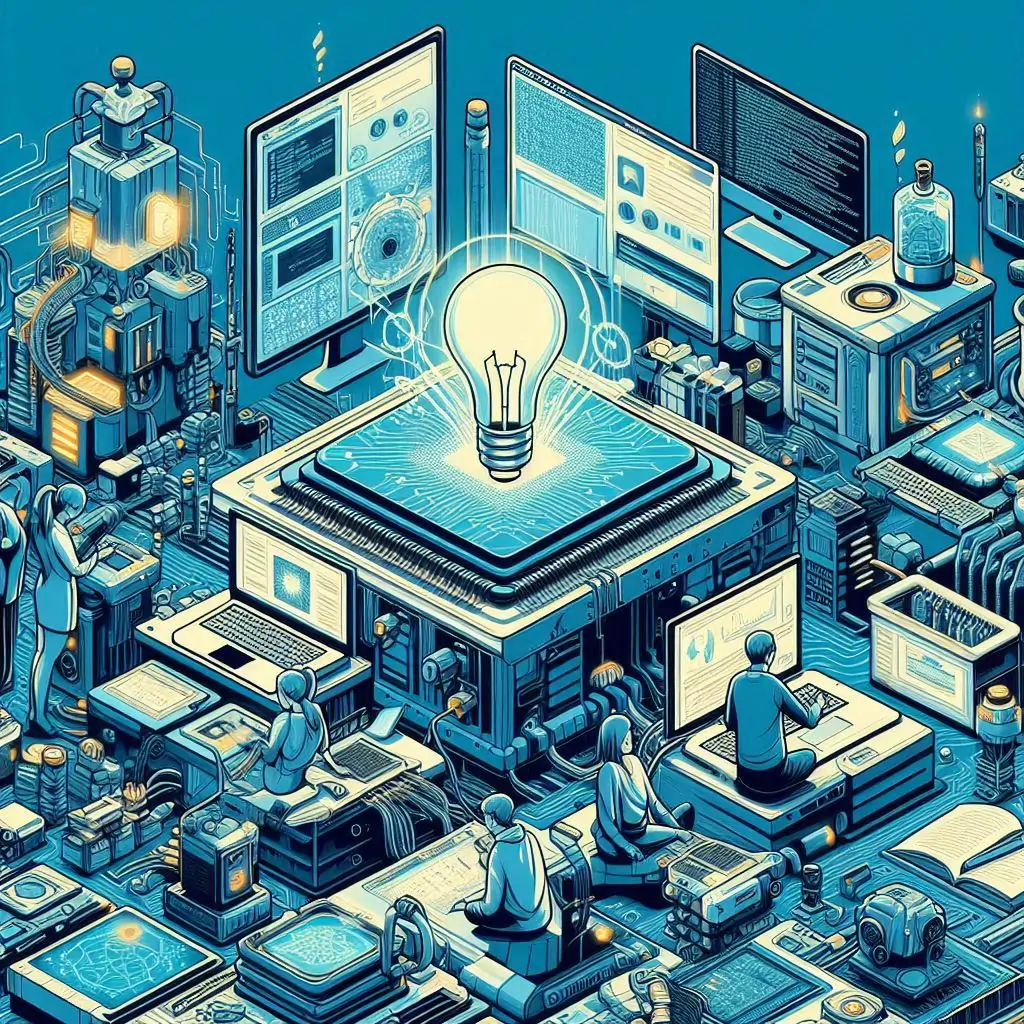
- Trace Generation (trace.c): Implement functions to generate trace files using different algorithms.
- Binary Manipulation (binary.h): Utilize the provided binary manipulation functions.
- Cache Simulation (simulator.c): Develop a cache simulator to measure cache performance.
Identifying the Objectives
Each part of the assignment has specific objectives:
- Trace Generation: You need to generate trace files using various algorithms such as realistic, random, single, and regular patterns.
- Binary Manipulation: Understand and use functions to pick out ranges of bits and convert them to integers.
- Cache Simulation: Create a simulator to evaluate cache behavior with different trace files.
Reviewing the Provided Code
Carefully review any provided code or libraries. In our example, binary.h contains binary manipulation functions that you will use. Understanding how these functions work is essential for completing the assignment successfully.
Planning Your Approach
A well-structured plan is key to tackling complex programming assignments. This section will help you organize your work and create a roadmap for implementation.
Setting Up Your Development Environment
Before you start coding, ensure that your development environment is properly set up:
- Install Necessary Tools: Make sure you have a C compiler installed, such as clang.
- Organize Your Files: Create a directory structure to keep your files organized. For example, you might have separate directories for trace.c, binary.h, and simulator.c.
Dividing the Assignment into Phases
Divide the assignment into phases, each focusing on a specific component:
- Phase 1: Implement and test the trace generation functions.
- Phase 2: Understand and integrate the binary manipulation functions.
- Phase 3: Develop and test the cache simulator.
Creating a Timeline
Set realistic deadlines for each phase. Allocate more time for components that you anticipate will be more challenging. For instance, the cache simulator might require more debugging and testing compared to the trace generation functions.
Implementing Trace Generation
The first major component of our assignment is trace generation. This section will guide you through the process of implementing different trace generation algorithms.
Realistic Trace Generation
The realistic trace generation function simulates the behavior of a typical program by randomly generating addresses and repeating them several times.
Understanding Realistic Traces
Realistic traces represent the control flow of a typical program, where certain instructions are executed multiple times due to loops and jumps. This pattern helps in understanding how a program might interact with the cache.
Implementing the Function
Here’s how you can implement the realistic trace generation function:
void generate_realistic_trace(FILE *file) {
srand(time(NULL));
for (int i = 0; i < 1000; i++) {
int address = rand() % 1025;
for (int j = 0; j < (rand() % 5 + 1); j++) {
fprintf(file, "%d\n", address);
}
}
}
Testing the Function
Compile your code using clang -lm trace.c -o trace and run it with ./trace. Check the generated realistic.txt file to ensure it contains the expected pattern.
Random Trace Generation
The random trace generation function generates addresses randomly without any specific pattern.
Understanding Random Traces
Random traces simulate a program that accesses memory locations in a completely random manner. This is useful for testing the worst-case scenario for cache performance.
Implementing the Function
Here’s how you can implement the random trace generation function:
void generate_random_trace(FILE *file) {
srand(time(NULL));
for (int i = 0; i < 1000; i++) {
int address = rand() % 1025;
fprintf(file, "%d\n", address);
}
}
Testing the Function
Compile and run your code as before. Check the generated random.txt file to ensure it contains a random sequence of addresses.
Single Trace Generation
The single trace generation function generates a single address repeatedly.
Understanding Single Traces
Single traces simulate a program that executes the same instruction repeatedly. This is useful for understanding the best-case scenario for cache performance.
Implementing the Function
Here’s how you can implement the single trace generation function:
void generate_single_trace(FILE *file) {
for (int i = 0; i < 1000; i++) {
fprintf(file, "0\n");
}
}
Testing the Function
Compile and run your code as before. Check the generated single.txt file to ensure it contains the same address repeated multiple times.
Regular Trace Generation
The regular trace generation function generates addresses in a regular, cyclic pattern.
Understanding Regular Traces
Regular traces simulate a program that accesses memory locations in a predictable, cyclic manner. This can help in understanding how well the cache handles regular access patterns.
Implementing the Function
Here’s how you can implement the regular trace generation function:
void generate_regular_trace(FILE *file) {
for (int i = 0; i < 1000; i++) {
fprintf(file, "%d\n", i % 1025);
}
}
Testing the Function
Compile and run your code as before. Check the generated regular.txt file to ensure it contains a regular sequence of addresses.
Utilizing Binary Manipulation Functions
The second major component involves binary manipulation functions. This section will guide you through understanding and utilizing these functions effectively.
Understanding Binary Manipulation
Binary manipulation involves working with binary representations of data. In our example, binary.h provides functions to pick out ranges of bits and convert them to integers.
Picking Out Bit Ranges
The binaryStringRangeToInt function extracts a range of bits from a binary string and converts it to an integer.
int binaryStringRangeToInt(char* bin, int start, int stop) {
int result = 0;
for (int i = start; i <= stop; i++) {
result = (result << 1) | (bin[i] - '0');
}
return result;
}
Converting Strings to Integers
Understanding how to convert binary strings to integers is crucial for indexing and other operations in the cache simulator.
Testing Binary Functions
Create a test program to validate the functionality of the binary manipulation functions. Ensure that the extracted bit ranges and conversions are accurate.
Integrating Binary Functions
Integrate the binary manipulation functions into your trace generation and cache simulation code where needed. For example, use these functions to parse addresses in the cache simulator.
Debugging Binary Functions
If you encounter any issues, use debugging techniques such as printing intermediate results and using a debugger to step through the code. Ensure that all binary manipulations are performed correctly.
Developing the Cache Simulator
The final major component is the cache simulator. This section will guide you through the process of developing and testing a cache simulator.
Understanding Cache Simulation
Cache simulation involves mimicking the behavior of a CPU cache to measure performance metrics such as hit and miss rates.
Direct Mapping Cache
Our example uses a direct mapping cache with 4 cache sets, each with 1 cache line and 1 block of information. Understanding this structure is crucial for implementing the simulator.
Eviction and Replacement Policy
Implement an accurate eviction and replacement policy to ensure the simulator works correctly. The provided code uses a replace-always policy by default.
Implementing the Cache Simulator
Here’s how you can implement the cache simulator:
void simulate_cache(const char *trace_file) {
// Initialize cache and other variables
Cache cache;
initialize_cache(&cache);
FILE *file = fopen(trace_file, "r");
char line[32];
while (fgets(line, sizeof(line), file)) {
int address = atoi(line);
access_cache(&cache, address);
}
fclose(file);
// Print cache statistics
print_cache_statistics(&cache);
}
Testing the Cache Simulator
Compile your code using clang -std=c11 -lm simulator.c -o simulator and run it with ./simulator realistic.txt. Test with different trace files to ensure the simulator works correctly under various scenarios.
Optimizing Cache Performance
To create cache-friendly and cache-unfriendly programs, generate trace files with different access patterns:
- Cache Unfriendly: Generate random addresses to cause more cache misses.
- Cache Friendly: Generate repetitive or regular addresses to cause more cache hits.
Conclusion
By following a structured approach, you can tackle complex programming assignments more effectively. Understand the requirements, plan your approach, implement each component step-by-step, and test thoroughly. With practice, you'll become more proficient at solving similar assignments.
For more help with programming assignments, visit ProgrammingAssignmentHelper.com, where we provide expert assistance at affordable prices.