How to Approach Complex Network Alias Listing Assignments in C Programming
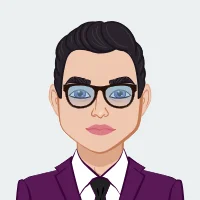
When it comes to network-related programming assignments, especially those involving the processing of IP addresses and aliases, students often encounter challenges in managing data, sorting it correctly, and outputting it in a specific format. One such task is creating a network alias listing assignment, which requires students to read, process, and sort a list of Internet addresses and their associated nicknames from a file, then output the sorted data to a new file. If you're looking for help with C assignment tasks like this, you've come to the right place. This guide will walk you through the essential steps and strategies to solve this type of assignment efficiently. In this blog, we will break down the problem, design an effective solution, and provide best practices that can help you approach and solve any similar assignment.
Understanding the Problem Statement
Before jumping into coding, it’s essential to first understand the problem you’re tackling. The key to solving any assignment lies in the details provided in the problem statement. For this network alias listing assignment, the challenge revolves around reading a file containing IPv4 addresses and their corresponding nicknames (aliases), sorting the data, and generating a well-formatted report.
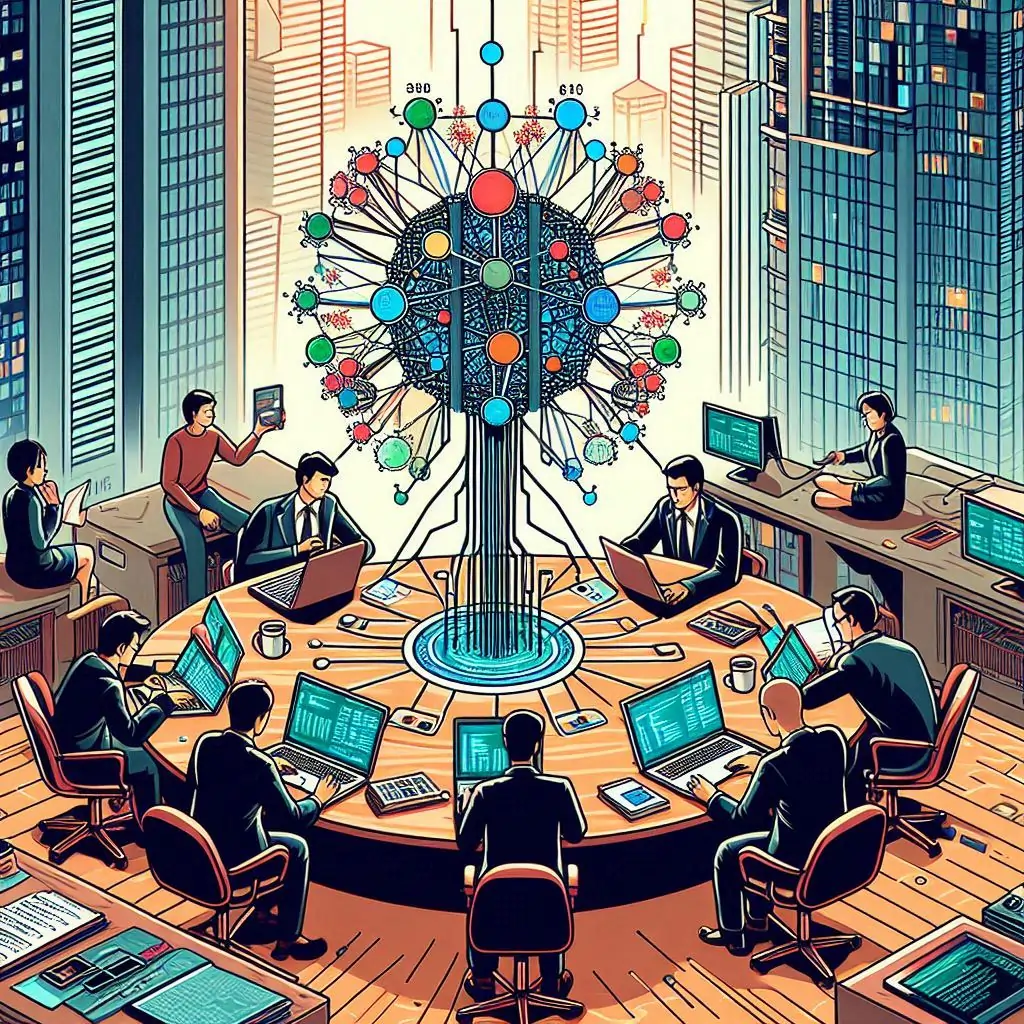
1. Grasping the Requirements
The problem involves several tasks that need to be understood individually. First, you're required to read a list of Internet addresses and aliases from a file named CS222_Inet.txt. The addresses are in dotted decimal notation (four octets), and the aliases are nicknames that may not be case-sensitive. The data in this file needs to be processed and stored in a way that can be sorted based on the aliases.
Next, you're required to prompt the user for their preferred sorting order (ascending or descending). After sorting the list, the program must generate a report, which is saved to another file, 222_Alias_List. This report will list the aliases and addresses in the specified order and must include the user's name and the current date.
2. Defining the Constraints and Edge Cases
When approaching this type of assignment, it’s vital to be aware of the constraints that may affect the functionality of your program. For example:
- Input Limit: The list should contain no more than 100 addresses and aliases.
- Termination: The file contains a sentinel value (an address of all zeros and the alias "NONE") that signals the end of the list. This must be handled correctly to prevent further data processing.
- Case Sensitivity: While reading the file, note that aliases are not case-sensitive, so your sorting algorithm should account for this.
- Formatting: The report must list all aliases in uppercase, even if they are stored in lowercase or mixed case in the input file.
3. Analyzing the Output Requirements
The output of the program will be saved in the file 222_Alias_List. This report should include:
- A heading with the user's name and the current date.
- The sorted list of aliases and their associated IP addresses.
- A clear format where the aliases are printed in uppercase, regardless of how they appeared in the input file.
It's essential to pay attention to details like formatting, as failing to meet the output requirements can affect the overall correctness of your solution.
Designing the Program Structure
Once you have a clear understanding of the problem statement, the next step is to design the program structure. This involves breaking down the problem into smaller, manageable components and planning the overall logic and flow of your program.
1. Creating the Address Structure
The first step in designing your program is defining a custom data structure to represent the IP addresses and their associated aliases. In C, this is typically done using a struct, which can store the four octets of the IP address and the alias as a string.
typedef struct {
int octet1;
int octet2;
int octet3;
int octet4;
char alias[11]; // Up to 10 characters plus null terminator
} address_t;
This structure, address_t, will store each IP address as four separate integers (one for each octet) and the alias as a string of up to 10 characters. The next step is to declare an array of 100 address_t structures to store the list of addresses and aliases read from the input file.
2. Planning the Functions
Your program will need several user-defined functions (UDFs) to handle different aspects of the problem. At a minimum, you will need functions to:
- Read the data from the input file.
- Sort the data based on aliases.
- Generate the sorted alias list and save it to a file.
- Get the current date and time for inclusion in the report.
Here’s a quick overview of the necessary functions:
void Read_Data_File(FILE *file, address_t addresses[], int *num_addresses);
void Bubble_sort(address_t addresses[], int num_addresses, bool ascending);
void Generate_Alias_List(FILE *output_file, address_t addresses[], int num_addresses, const char *username);
void getDateAndTime(char *date_str);
3. Defining the Main Program Flow
The main function of your program will control the overall flow and call the appropriate functions at the right times. Here's a general outline of the main function:
- Read Data: Call Read_Data_File to read the IP addresses and aliases from the file and store them in the array of structures.
- User Input: Prompt the user for their preferred sorting order (ascending or descending).
- Sort Data: Use the Bubble Sort algorithm to sort the data based on the aliases.
- Generate Report: Call Generate_Alias_List to create the report and save it to the file.
- Output Confirmation: Notify the user that the report has been successfully generated.
Implementing the Key Functions
With the structure and design in place, you can now focus on implementing the key functions of your program. Each function should be written to handle specific tasks efficiently and correctly.
1. Reading the Data from the File
The Read_Data_File function will read the data from CS222_Inet.txt, parse the IP addresses and aliases, and store them in the array of structures. This function should also keep track of the number of addresses read and handle the sentinel value to stop reading further input.
void Read_Data_File(FILE *file, address_t addresses[], int *num_addresses) {
*num_addresses = 0;
while (fscanf(file, "%d.%d.%d.%d %s",
&addresses[*num_addresses].octet1,
&addresses[*num_addresses].octet2,
&addresses[*num_addresses].octet3,
&addresses[*num_addresses].octet4,
addresses[*num_addresses].alias) == 5) {
if (addresses[*num_addresses].octet1 == 0 &&
addresses[*num_addresses].octet2 == 0 &&
addresses[*num_addresses].octet3 == 0 &&
addresses[*num_addresses].octet4 == 0 &&
strcmp(addresses[*num_addresses].alias, "NONE") == 0) {
break;
}
(*num_addresses)++;
}
}
2. Sorting the Data with Bubble Sort
Once the data is read, the next task is to sort the aliases alphabetically based on the user’s choice of ascending or descending order. The Bubble Sort algorithm can be used in conjunction with strcmp() to compare the aliases.
void Bubble_sort(address_t addresses[], int num_addresses, bool ascending) {
int i, j;
address_t temp;
for (i = 0; i < num_addresses - 1; i++) {
for (j = 0; j < num_addresses - i - 1; j++) {
if ((ascending && strcmp(addresses[j].alias, addresses[j + 1].alias) > 0) ||
(!ascending && strcmp(addresses[j].alias, addresses[j + 1].alias) < 0)) {
temp = addresses[j];
addresses[j] = addresses[j + 1];
addresses[j + 1] = temp;
}
}
}
}
3. Generating and Writing the Alias List
Once the data is sorted, the next step is to generate the alias list and save it to the output file 222_Alias_List. The report should include the user's name, the current date, and the list of aliases in uppercase.
void Generate_Alias_List(FILE *output_file, address_t addresses[], int num_addresses, const char *username) {
char date_str[20];
getDateAndTime(date_str);
fprintf(output_file, "%s %s\n", username, date_str);
fprintf(output_file, "CS222 Network Alias Listing\n\n");
for (int i = 0; i < num_addresses; i++) {
fprintf(output_file, "%s %d.%d.%d.%d\n",
strupr(addresses[i].alias),
addresses[i].octet1,
addresses[i].octet2,
addresses[i].octet3,
addresses[i].octet4);
}
}
4. Getting the Current Date and Time
To include the current date in the report, you can use the time.h library in C to get the system's date and time.
void getDateAndTime(char *date_str) {
time_t t = time(NULL);
struct tm tm = *localtime(&t);
sprintf(date_str, "%02d-%02d-%04d", tm.tm_mday, tm.tm_mon + 1, tm.tm_year + 1900);
}
5. Handling User Input
When prompting the user for input on sorting order, ensure you handle their input correctly and validate it to avoid invalid choices.
bool getUserSortPreference() {
char choice;
printf("Do you want to sort the aliases in ascending or descending order? (A/D): ");
scanf(" %c", &choice);
return toupper(choice) == 'A';
}
Testing and Debugging
Now that the program is designed and implemented, the final step is testing. Creating thorough test cases is essential to ensure your program works as expected under various conditions. This includes:
- Testing with different sets of data.
- Verifying that the sorting works correctly for both ascending and descending orders.
- Checking edge cases, such as when the input file contains only a few addresses or when the sentinel value is encountered early.
After testing your program thoroughly, make sure to debug any issues that arise. Common issues may include incorrect file handling, misformatted output, or faulty sorting logic. Use debugging tools and print statements to help isolate and fix these problems.
By following these guidelines and breaking down the problem step by step, you can confidently approach and solve your programming assignment. Remember that the key to success is understanding the problem thoroughly, designing your program structure carefully, and testing your code to ensure it meets all requirements.