Conquer LZW Compression Assignments: Breakdown, Implementation, and Optimization
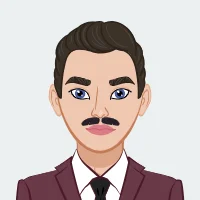
Compression algorithms are essential in data storage and transmission, as they significantly reduce the size of data files without compromising the content's quality. One such algorithm is the LZW (Lempel-Ziv-Welch) algorithm, which, despite its complexity, can be effectively managed with a structured approach. If you're seeking assistance with C++ assignments involving these algorithms, this guide is designed to help you through the process. By breaking down the LZW algorithm, planning your approach methodically, and offering practical implementation tips, this guide aims to simplify the challenges associated with compression algorithms. Whether you're tackling this as part of a coursework or a personal project, understanding the LZW algorithm's nuances and applying it correctly can greatly enhance your programming skills and problem-solving abilities.
Understanding the Compression Algorithm
Before diving into coding, it's essential to thoroughly understand the algorithm you're implementing. The LZW algorithm, proposed by Terry Welch in 1984, builds upon the LZ77 algorithm introduced by Abraham Lempel and Jacob Ziv in 1977. This dictionary-based compression algorithm is widely known for its application in GIFs and the V.42 communication standard.
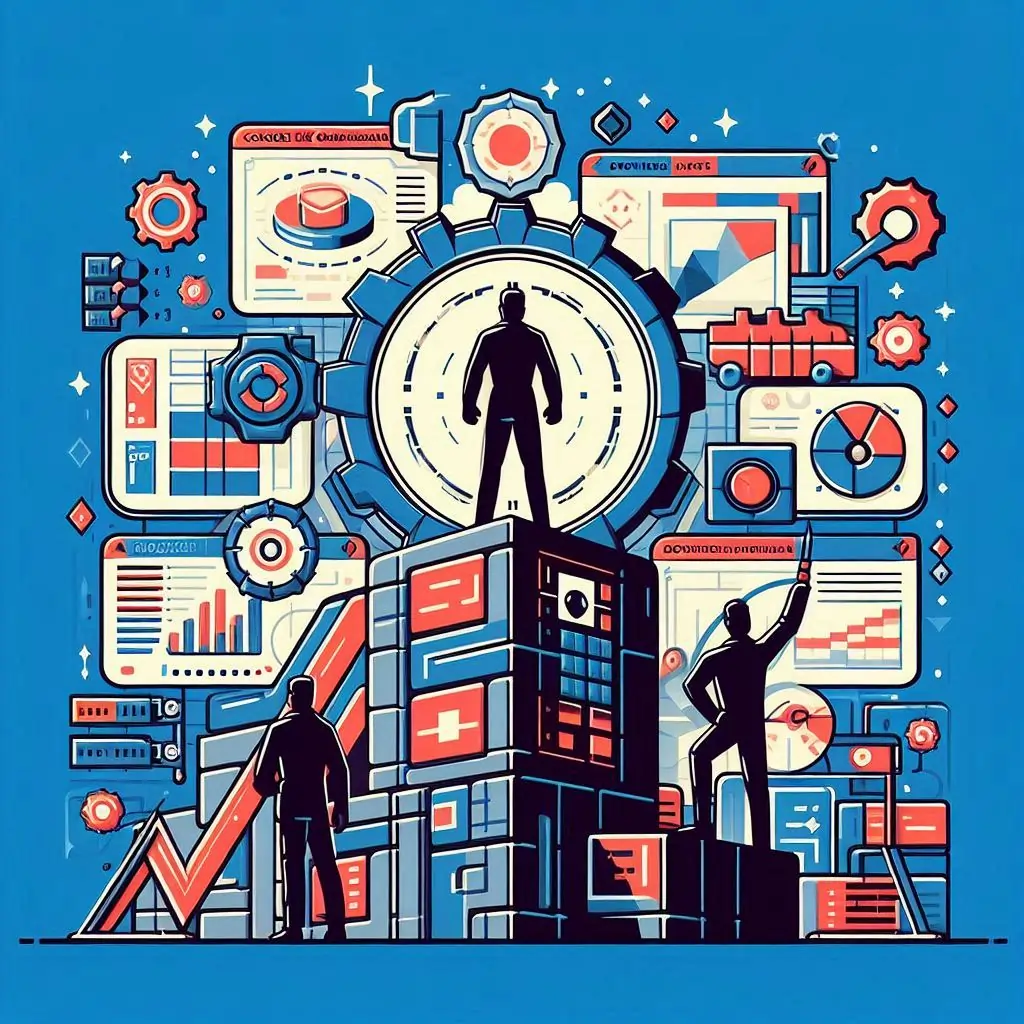
Algorithm Overview
The LZW algorithm operates by creating a dictionary of strings and their corresponding codes on the fly. It compresses data by finding the longest strings that match the dictionary entries and outputs the corresponding codes.
Compression Process
The compression process involves initializing a dictionary with the first 256 ASCII characters, reading input characters, and updating the dictionary as new strings are encountered.
Decompression Process
The decompression process reverses the compression steps, starting with a dictionary of ASCII characters and using codes from the compressed file to reconstruct the original strings.
Key Components of the LZW Algorithm
To implement the LZW algorithm effectively, you need to understand the key components involved in both compression and decompression.
Dictionary Initialization
Initialize the dictionary with the first 256 ASCII characters for both compression and decompression processes.
Handling Input and Output
Efficiently read from input files and write to output files using provided functions like readCode() and writeCode().
Managing the Dictionary
Maintain and update the dictionary dynamically during compression and decompression, ensuring efficient lookup and handling of dictionary overflow.
Planning Your Approach
Solving a programming assignment, such as implementing the LZW algorithm, requires careful planning and a structured approach. Here's a step-by-step guide to help you navigate the process.
Step 1: Read and Understand the Requirements
Before you start coding, thoroughly read the assignment requirements. Note key points such as the use of 12-bit codewords, dictionary handling, multiple file compression, and command-line interface specifications.
1: Identify Key Requirements
- Use ANSI C/C++ and standard libraries.
- Compress and decompress files of any length.
- Handle multiple files in a single archive.
- Implement efficient dictionary management.
2: Understand the Provided Skeleton Code
Familiarize yourself with the provided skeleton code and understand the existing functions and their roles. This will help you integrate your implementation seamlessly.
3: Outline the Algorithm Steps
Break down the algorithm into smaller tasks, such as dictionary initialization, reading input, dictionary lookup, outputting codes, and handling dictionary overflow.
Step 2: Set Up Your Development Environment
Ensure your development environment is ready for coding. For C/C++ assignments, you can use Visual Studio, GCC, or Clang. Verify that your compiler settings match the assignment requirements.
1: Install Necessary Tools
Install and configure your development tools, ensuring they are compatible with the assignment specifications.
2: Test the Provided Skeleton Code
Compile and run the provided skeleton code to ensure it works correctly. This will help you identify any issues early on.
Step 3: Implement the Compression Function
Start by implementing the compression function. This involves initializing the dictionary, reading the input file, building and searching the dictionary, and outputting codes.
1: Initialize the Dictionary
Begin with the first 256 ASCII characters, mapping each character to its corresponding code.
#include
#include
void initializeDictionary(std::unordered_map& dictionary) {
for (int i = 0; i < 256; ++i) {
dictionary[std::string(1, char(i))] = i;
}
}
int main() {
std::unordered_map dictionary;
initializeDictionary(dictionary);
// Rest of the compression code...
return 0;
}
2: Read Input and Update Dictionary
Use file I/O operations to read characters from the input file and update the dictionary as new strings are formed.
#include
#include
#include
void compress(const std::string& inputFile, const std::string& outputFile) {
std::unordered_map dictionary;
initializeDictionary(dictionary);
std::ifstream input(inputFile, std::ios::binary);
std::ofstream output(outputFile, std::ios::binary);
std::string prefix;
char character;
while (input.get(character)) {
std::string newPrefix = prefix + character;
if (dictionary.find(newPrefix) != dictionary.end()) {
prefix = newPrefix;
} else {
output << dictionary[prefix] << " ";
dictionary[newPrefix] = dictionary.size();
prefix = character;
}
}
if (!prefix.empty()) {
output << dictionary[prefix] << " ";
}
input.close();
output.close();
}
3: Handle Dictionary Overflow
Ensure the dictionary is reset when it becomes full, starting with the first 256 entries again.
Step 4: Implement the Decompression Function
Next, implement the decompression function, which involves initializing the dictionary, reading codes from the archive, looking up and outputting strings, and updating the dictionary.
1: Initialize the Dictionary
Start with the first 256 ASCII characters, mapping each code to its corresponding character or string.
2: Read Codes and Output Strings
Read codes from the archive, retrieve the corresponding strings, and output them to the decompressed file.
3: Update the Dictionary
Update the dictionary dynamically as new strings are formed during decompression.
Step 5: Handle Multiple Files
Modify your implementation to handle multiple files in a single compressed archive. Ensure the header and EOF codes are correctly managed, and reset the dictionary appropriately when needed.
1: Manage File Headers
Save a header in your compressed file with the list of filenames, followed by the compressed data for each file.
2: Insert EOF Codes
Insert the EOF code (4095) to indicate the end of each file within the archive.
Step 6: Testing and Debugging
Thoroughly test your implementation with various input files to ensure correctness. Compare your results with the provided example executable and debug any discrepancies.
1: Create Test Cases
Develop a set of test cases that cover different scenarios, including edge cases and large files.
2: Automate Testing
Automate the testing process using scripts to run your program with different inputs and compare the outputs.
Step 7: Optimize for Performance
Consider data structures like hash tables or trees for efficient dictionary lookup and ensure your implementation runs within acceptable time limits.
1: Use Efficient Data Structures
Choose appropriate data structures that offer fast lookup and insertion times.
2: Profile and Optimize Code
Profile your code to identify bottlenecks and optimize critical sections for better performance.
Step 8: Documentation and Submission
Comment your code to explain key parts and logic. Ensure your code adheres to submission guidelines, including the command line format and compilation instructions.
1: Write Clear Comments
Document your code with clear and concise comments to explain the purpose and functionality of each section.
2: Review Submission Requirements
Double-check the submission guidelines to ensure your code meets all requirements and compiles without errors.
Implementing the LZW Algorithm: Practical Tips
When implementing the LZW algorithm, keep these practical tips in mind to ensure a smooth and successful experience.
Understanding the Provided Skeleton Code
The skeleton code provides a foundation for your implementation, including functions for reading and writing codes. Familiarize yourself with these functions to integrate your compression and decompression logic seamlessly.
Skeleton Code Structure
The provided skeleton code typically includes the following structure:
- Initialization Functions: Functions to initialize the dictionary.
- File I/O Functions: Functions to read from and write to files.
- Compression and Decompression Stubs: Placeholders for your implementation.
Handling File I/O Efficiently
Efficient file I/O is crucial for the performance of your compression and decompression functions. Use the provided functions and standard libraries to handle file operations effectively.
Reading Input Files
Use standard file I/O functions to read characters from input files and store them in appropriate data structures.
Writing Output Files
Write the compressed codes and decompressed strings to output files using efficient file I/O operations.
Managing the Dictionary
The dictionary is a critical component of the LZW algorithm. Choose data structures that offer fast lookup and insertion times, such as hash tables or trees.
Dictionary Lookup
Implement efficient dictionary lookup mechanisms to quickly find strings and codes during compression and decompression.
Handling Dictionary Overflow
When the dictionary becomes full, reset it to its initial state to ensure continued operation without errors.
Sample Code Implementation
Below are sample code snippets to illustrate key parts of the LZW algorithm implementation in C++.
Dictionary Initialization for Compression
#include
< iostream >
#include
< unordered_map >
void initializeDictionary(std::unordered_map& dictionary) {
for (int i = 0; i < 256; ++i) {
dictionary[std::string(1, char(i))] = i;
}
}
int main() {
std::unordered_map dictionary;
initializeDictionary(dictionary);
// Rest of the compression code...
return 0;
}
Reading Input and Updating Dictionary
#include
< fstream >
#include
< string >
#include
void compress(const std::string& inputFile, const std::string& outputFile) {
std::unordered_map dictionary;
initializeDictionary(dictionary);
std::ifstream input(inputFile, std::ios::binary);
std::ofstream output(outputFile, std::ios::binary);
std::string prefix;
char character;
while (input.get(character)) {
std::string newPrefix = prefix + character;
if (dictionary.find(newPrefix) != dictionary.end()) {
prefix = newPrefix;
} else {
output << dictionary[prefix] << " ";
dictionary[newPrefix] = dictionary.size();
prefix = character;
}
}
if (!prefix.empty()) {
output << dictionary[prefix] << " ";
}
input.close();
output.close();
}
Handling Multiple Files
Modify your implementation to handle multiple files within a single compressed archive. Ensure the dictionary is reset appropriately when needed.
void compressMultipleFiles(const std::vector
< std::string >
& inputFiles, const std::string& outputFile) {
std::unordered_map dictionary;
initializeDictionary(dictionary);
std::ofstream output(outputFile, std::ios::binary);
for (const auto& inputFile : inputFiles) {
std::ifstream input(inputFile, std::ios::binary);
std::string prefix;
char character;
while (input.get(character)) {
std::string newPrefix = prefix + character;
if (dictionary.find(newPrefix) != dictionary.end()) {
prefix = newPrefix;
} else {
output << dictionary[prefix] << " ";
dictionary[newPrefix] = dictionary.size();
prefix = character;
}
}
if (!prefix.empty()) {
output << dictionary[prefix] << " ";
}
input.close();
// Insert EOF code (e.g., 4095)
output << 4095 << " ";
// Reset dictionary for the next file
dictionary.clear();
initializeDictionary(dictionary);
}
output.close();
}
Conclusion
Implementing compression algorithms like LZW requires a structured approach and a clear understanding of the underlying logic. By breaking down the task into manageable steps, planning your approach, and rigorously testing your implementation, you can successfully tackle similar assignments. Use the provided code snippets and tips as a starting point, and adapt them to fit the specific requirements of your assignment. Happy coding!
Through this guide, you now have a comprehensive understanding of how to approach programming assignments involving compression algorithms. By following the detailed steps, implementing the provided code snippets, and optimizing your solution, you can master the art of compression algorithms and excel in your programming assignments.