The Essentials of Secure Distributed Systems Implementation
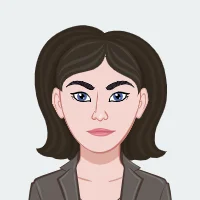
Distributed systems are essential for creating robust, scalable applications that can handle various tasks across multiple nodes. When building these systems, security is a critical consideration to protect data and ensure proper access control. This guide provides a comprehensive approach to implementing secure distributed systems, using the example of a Secure Shared Store (3S) service to illustrate the principles and best practices. For those seeking cryptography assignment help, this guide also highlights how to leverage cryptographic techniques to enhance security in distributed systems.
Understanding Distributed Systems Security Assignments
Distributed systems security assignments, such as the Secure Shared Store (3S) service described in the provided project outline, require a blend of theoretical knowledge and practical skills. The goal is to develop secure services that allow multiple users to store and retrieve documents securely. Key components include mutual authentication, secure communication, user authentication, session management, and access control. This section will delve into these fundamental concepts, setting the stage for a structured approach to solving similar assignments.
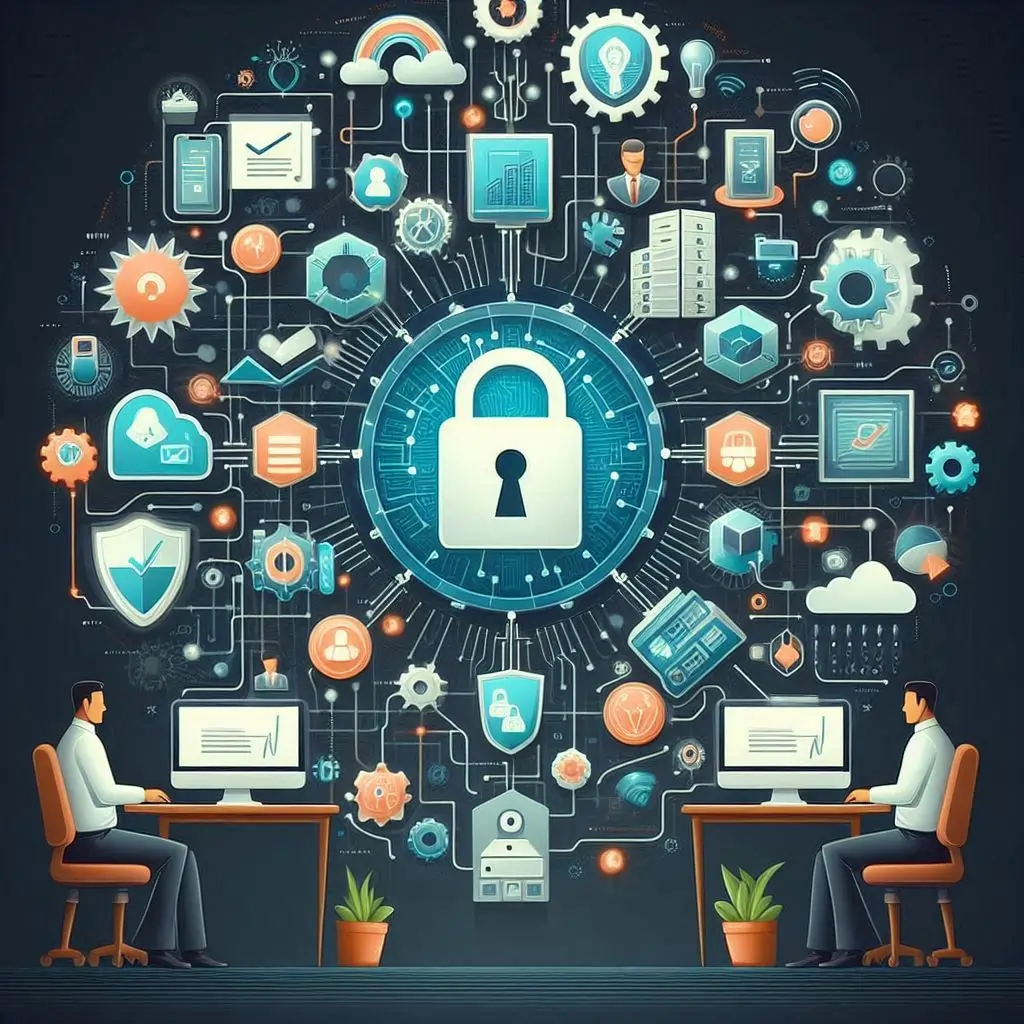
1. Certificate Authority (CA) Setup
A Certificate Authority (CA) is a trusted entity that issues digital certificates, which are essential for establishing secure communications in a distributed system. The CA acts as a root of trust, ensuring that all certificates used within the system are reliable and authentic.
Setting Up the CA: To begin, set up a CA using a tool like OpenSSL. This involves generating a root certificate and private key, which will be used to sign the certificates for the server and client nodes. The steps typically include:
1. Generate the CA’s private key:
openssl genrsa -out ca.key 2048
2. Create the root certificate:
openssl req -x509 -new -nodes -key ca.key -sha256 -days 1024 -out ca.crt
3. Generate the server and client certificates: Using the CA, generate certificates for the server and client nodes. Place these certificates in the appropriate directories within the project structure.
2. Secure Communication
Secure communication ensures that data transmitted between the server and client nodes is encrypted and protected from eavesdropping or tampering. This is achieved through mutual authentication and secure protocols.
Implementing Mutual Authentication: Mutual authentication (MTLS) requires both the server and client to present and verify each other’s certificates before establishing a secure connection. This can be configured using Nginx or directly within the server code. The typical process involves:
1. Configure the server to require client certificates: Modify the server configuration to enforce mutual authentication. For instance, in an Nginx configuration file:
server {
listen 443 ssl;
ssl_certificate /path/to/server.crt;
ssl_certificate_key /path/to/server.key;
ssl_client_certificate /path/to/ca.crt;
ssl_verify_client on;
}
2. Update the client to present its certificate: Ensure the client includes its certificate in requests to the server. For example, in a Python client:
import requests
response = requests.get('https://secure-shared-store', cert=('client.crt', 'client.key'))
Using Secure Protocols: Adopt secure protocols such as HTTPS to encrypt data in transit. Ensure all endpoints are accessed securely, and configure your server to redirect HTTP requests to HTTPS.
3. User Authentication and Session Management
Effective user authentication and session management are crucial for maintaining security in distributed systems. Users must authenticate themselves using private keys, and sessions should be managed to prevent unauthorized access.
User Authentication: Authentication involves verifying the identity of users before granting access to the system. In this project, users authenticate using their private keys. The server validates these keys and issues session tokens upon successful authentication.
1. User login process:
- The user provides their user ID (UID) and the filename of their private key.
- The client signs a statement using the private key and sends it to the server for verification.
- Upon successful verification, the server generates a unique session token.
2. Session management:
- The session token is included in all subsequent requests to the server.
- Ensure that each user has a unique UID and that only one active session per user is allowed.
Implementing Document Management Features
The core functionalities of the 3S service revolve around document management, including check-in, check-out, and deletion. Each operation must be secure and respect the access control policies defined by document owners.
1. Document Check-in
Checking in a document involves uploading it to the server, where it is stored securely. The server must handle both confidentiality and integrity, depending on the security flag specified.
Confidentiality: When a document is checked in with a confidentiality flag, the server encrypts the document using a randomly generated AES key. This key is then encrypted with the server's public key and stored with the document's metadata.
1. Encrypt the document:
from Crypto.Cipher import AES
key = os.urandom(32)
cipher = AES.new(key, AES.MODE_EAX)
ciphertext, tag = cipher.encrypt_and_digest(data)
2. Store the encrypted document and key: Save the ciphertext and the encrypted AES key on the server.
Integrity: For documents requiring integrity, the server signs the document to ensure it has not been tampered with.
1. Sign the document:
from Crypto.Signature import pss
from Crypto.Hash import SHA256
from Crypto.PublicKey import RSA
key = RSA.import_key(open('private.pem').read())
h = SHA256.new(data)
signature = pss.new(key).sign(h)
2. Store the signed document: Save the document and its signature on the server.
2. Document Check-out
Checking out a document involves retrieving it from the server. The server must verify the requestor's permissions and handle the document appropriately based on its security flag.
Handling Confidentiality: For documents stored with confidentiality, the server decrypts the document before sending it to the client.
1. Decrypt the document:
cipher = AES.new(key, AES.MODE_EAX, nonce)
data = cipher.decrypt_and_verify(ciphertext, tag)
Handling Integrity: For documents stored with integrity, the server verifies the document's signature before sending it.
2. Verify the signature:
verifier = pss.new(key)
verifier.verify(h, signature)
3. Send the document: If verification succeeds, send the document to the client.
3. Document Deletion
Deleting a document involves permanently removing it from the server, ensuring it cannot be recovered even if the server is compromised.
Secure Deletion: For confidential documents, this also means securely deleting the encryption key.
1. Delete the document and key: Ensure the document and its associated key (if applicable) are removed from the server's storage.
Implementing Access Control
Access control is essential for managing who can perform actions on documents. The owner of a document defines the access control policies, specifying who can check in, check out, or delete documents.
1. Granting Access
The grant function allows document owners to authorize other users to perform specific actions on their documents for a specified period.
- Define access rights: Access rights can include check-in, check-out, or both.
- Update the access control policy: Store the updated policy in the server’s metadata for the document.
- Handle grant scenarios: Ensure the latest grant for a specific document and user is always the effective rule.
2. Implementing Access Control Policies
Access control policies must be enforced consistently across all document operations.
- Check permissions before actions: Verify the user’s permissions before allowing them to check in, check out, or delete documents.
- Track document ownership: Maintain metadata to track the owner of each document and any granted access rights.
Best Practices for Secure Coding and Testing
Secure coding practices and thorough testing are critical to ensure the robustness of your distributed system. Here are some best practices to follow:
1. Secure Coding Practices
- Use well-maintained libraries: Adopt libraries and frameworks that are widely used and regularly updated.
- Avoid common vulnerabilities: Protect against SQL injection, cross-site scripting (XSS), and buffer overflows.
- Encrypt sensitive data: Ensure sensitive data is encrypted both in transit and at rest.
2. Testing and Code Analysis
- Static code analysis: Use tools like Pylint and Pyflakes to analyze your code for potential vulnerabilities.
- Automated testing: Implement automated tests to validate the functionality and security of your system.
- Document threat models: Identify potential threats and document how your implementation mitigates them.
Conclusion
Distributed systems security is a challenging but essential field, particularly in scenarios involving secure document storage and retrieval. By understanding the problem, setting up a robust development environment, implementing secure communication, managing user authentication and sessions, and adhering to best practices in secure coding, you can effectively tackle complex assignments like the Secure Shared Store (3S) service. Remember to continuously test your system and stay updated with the latest security practices to ensure your implementation is resilient against emerging threats.
By following the comprehensive guide outlined in this blog, you will be well-prepared to handle distributed systems security assignments and develop secure, reliable services.