Implementing Array Manager and Paged Arrays in Java
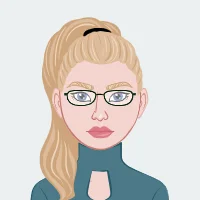
Programming assignments often involve working with arrays, one of the fundamental data structures in computer science. Understanding how to efficiently manage, manipulate, and optimize arrays is essential for any aspiring programmer. This guide aims to help you tackle Java assignments with confidence, using a sample problem to illustrate key concepts and techniques that can be applied to a wide range of similar tasks.
Understanding the Basics of Array Management
Array management involves several crucial operations such as creating, resizing, aliasing, and deleting arrays. These operations require a solid understanding of how memory works, especially when dealing with dynamic arrays that can change in size during runtime. In this section, we will break down the essential concepts and strategies for effective array management.
Creating and Deleting Arrays
Creating and deleting arrays are fundamental operations that form the basis of array management. Let's explore these processes in detail.
Creating Arrays
Creating an array involves allocating a contiguous block of memory to store elements. In Java, arrays are usually created with a fixed size, but dynamic resizing requires careful memory management to ensure efficiency and avoid errors.
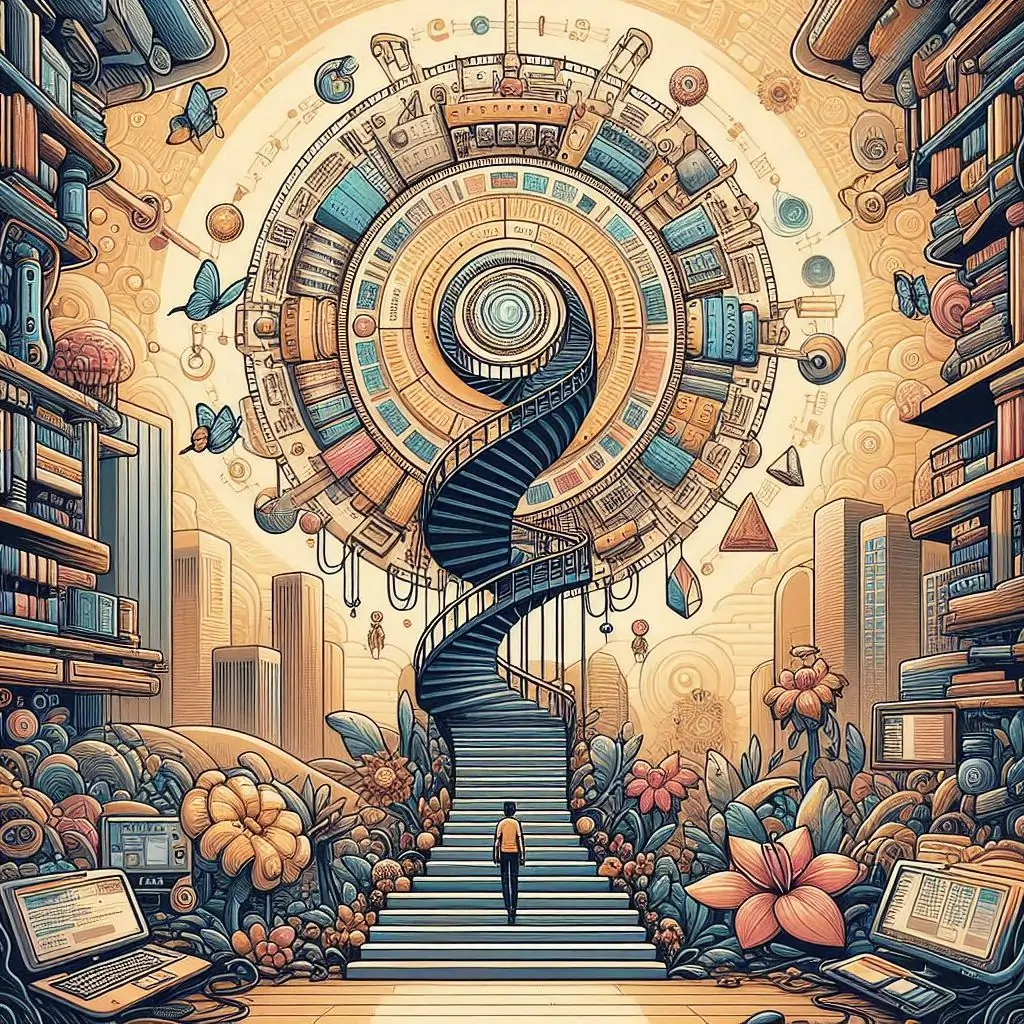
Deleting Arrays
Deleting an array involves deallocating the memory previously allocated to it, making it available for other operations. This is crucial for optimizing memory usage and preventing memory leaks.
Resizing Arrays
Resizing an array dynamically is a complex operation that requires understanding how to allocate and reallocate memory efficiently. When an array grows, new memory needs to be allocated, and existing elements must be copied to the new location. When an array shrinks, memory must be deallocated to free up space.
Aliasing Arrays
Aliasing allows multiple array references to point to the same memory location. This technique is useful for optimizing memory usage but requires careful handling to avoid issues like dangling references and unintended data modifications.
Implementing an Array Manager in Java
An Array Manager is a utility that provides a framework for creating, managing, and manipulating arrays dynamically. In this section, we'll walk through the process of implementing an Array Manager in Java, focusing on key components and functionalities.
Setting Up the Array Manager
The Array Manager is responsible for managing a large physical array and mapping virtual pages to physical frames. This setup allows for efficient memory management and supports dynamic resizing and aliasing.
Allocating Physical Memory
The first step in setting up the Array Manager is allocating a single, large array to serve as the physical memory. This array is divided into frames, which can be assigned to different arrays as needed.
Managing Free Frames
To keep track of available memory, the Array Manager maintains a list of free frames. When an array is created or resized, frames are allocated or deallocated from this list.
Creating and Manipulating Arrays
The Array Manager provides several methods for creating, resizing, aliasing, and deleting arrays. Each operation involves managing the mappings between virtual pages and physical frames.
Creating Arrays
When creating a new array, the Array Manager checks if there are enough free frames to accommodate the requested size. If not, an OutOfMemoryException is thrown. Otherwise, frames are allocated, and a new page table is created for the array.
Resizing Arrays
Resizing an array involves adjusting its page table and reallocating frames as needed. If the array grows, additional frames are allocated. If it shrinks, frames are deallocated and returned to the free frame list.
Aliasing Arrays
Aliasing is achieved by creating a new page table that shares frames with an existing array. This allows multiple arrays to reference the same memory, optimizing memory usage but requiring careful handling to avoid conflicts.
Advanced Techniques and Best Practices
Managing arrays effectively requires not only understanding basic operations but also implementing advanced techniques and following best practices. In this section, we'll explore some of these techniques and provide tips for writing efficient and reliable array management code.
Implementing Exception Handling
Proper exception handling is crucial for robust array management. Custom exceptions like OutOfMemoryException and SegmentationViolationException help manage errors and provide clear feedback when issues arise.
OutOfMemoryException
This exception is thrown when there are not enough free frames to create or resize an array. It helps prevent memory overflows and ensures that memory is allocated efficiently.
SegmentationViolationException
This exception is thrown when an array is accessed with an out-of-bounds index. It helps prevent segmentation faults and ensures that array access is safe and controlled.
Optimizing Memory Usage
Optimizing memory usage involves carefully managing the allocation and deallocation of frames, as well as implementing techniques like copy-on-write to minimize unnecessary memory usage.
Copy-On-Write Strategy
Copy-on-write (COW) is an optimization strategy that delays the copying of data until it is modified. This technique helps reduce memory usage and improve performance, especially when dealing with large arrays.
Efficient Memory Allocation
Efficient memory allocation involves minimizing fragmentation and ensuring that memory is used effectively. Techniques like maintaining a free frame list and using page tables help manage memory efficiently.
Testing and Debugging
Thorough testing and debugging are essential for ensuring that your array management code is reliable and performs as expected. This involves creating comprehensive test cases and using debugging tools to identify and fix issues.
Creating Test Cases
Test cases should cover all possible scenarios, including edge cases and error conditions. This helps ensure that your code handles all situations correctly and robustly.
Debugging Techniques
Debugging techniques like logging, breakpoints, and step-through debugging help identify and fix issues in your code. These tools provide insight into the internal state of your program and help track down bugs.
Conclusion
Array management is a critical skill for any programmer, and mastering it requires understanding both fundamental operations and advanced techniques. By following the guidelines and best practices outlined in this guide, you can confidently tackle array management assignments and build efficient, reliable code. Whether you're creating, resizing, aliasing, or deleting arrays, the principles discussed here will help you manage memory effectively and optimize your programs for performance and stability.