How to Approach and Solve Temperature Conversion Assignments in Python
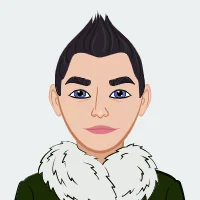
Taking on an existing codebase, especially one left behind without notice, can be a daunting task. However, it also presents a unique opportunity to develop your problem-solving skills and deepen your understanding of programming concepts. In this blog, we'll explore how to tackle a common assignment scenario: a temperature conversion program in Python. By the end of this guide, you'll be equipped with the knowledge and techniques to handle similar assignments with confidence and efficiency, ensuring you can complete your Python assignment effectively.
Understanding the Problem Statement
The first and most crucial step in solving your programming assignment is to thoroughly understand the problem statement. Let’s break down the given scenario:
Breaking Down the Requirements
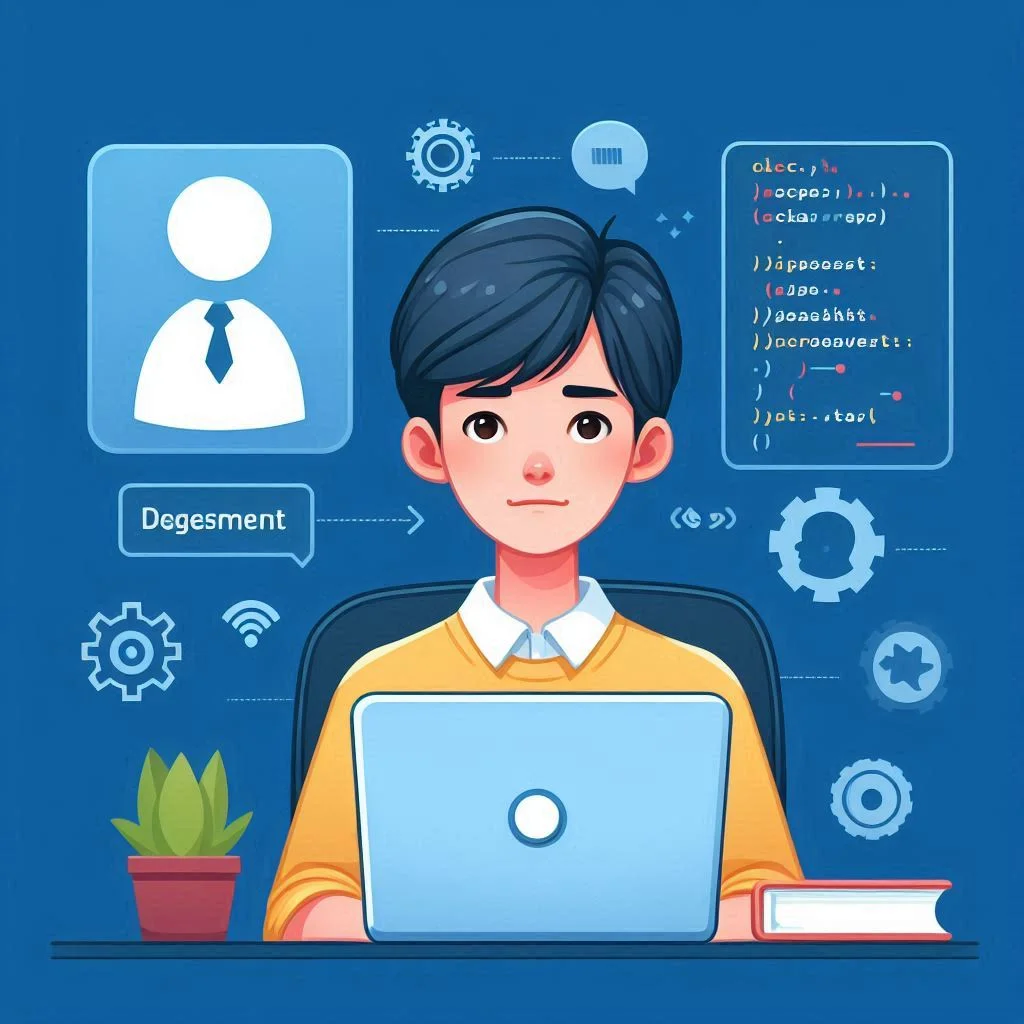
In the provided scenario, you are tasked with completing a nearly finished software that converts Fahrenheit to Celsius and provides clothing advice based on the temperature. Here are the detailed requirements:
- Ask the user for a temperature in Fahrenheit.
- Provide appropriate clothing advice:
- Hot (80°F or higher)
- Warm (60°F to 80°F)
- Cold (below 60°F)
- Display the equivalent temperature in Celsius.
- Ask if the user has another temperature to convert and repeat the process if they do.
Reviewing Existing Code and Pseudocode
If you have access to pseudocode or existing code, take the time to review it. Understanding the logic implemented by the previous developer can save you significant time and effort. Look for any patterns or structures that can be leveraged in your solution.
Identifying Key Components
To effectively tackle the problem, identify the key components that need to be developed or modified. In this case, the main components are user input, clothing advice logic, temperature conversion, result display, and loop control for repeating the process.
Structuring Your Approach
Once you have a clear understanding of the requirements and key components, the next step is to structure your approach. Breaking down the task into smaller, manageable parts makes the problem less intimidating and helps ensure you cover all aspects of the assignment.
User Input
Handling user input is a fundamental aspect of this assignment. You need to prompt the user for a temperature in Fahrenheit and ensure the input is valid.
Using the input() Function
In Python, the input() function is used to capture user input. For this assignment, you’ll use it to get the temperature in Fahrenheit from the user. Here’s an example:
fahrenheit = input("Enter temperature in Fahrenheit: ")
Validating Input
To prevent errors, it’s important to validate the user input. Ensure the input can be converted to a float and handle any exceptions that may occur:
try:
fahrenheit = float(input("Enter temperature in Fahrenheit: "))
except ValueError:
print("Please enter a valid number.")
continue
Providing Clothing Advice
Based on the temperature input, you need to provide appropriate clothing advice. This involves using conditional statements to categorize the temperature.
Using Conditional Statements
Conditional statements (if, elif, else) are used to determine the clothing advice based on the temperature ranges provided:
if fahrenheit >= 80:
advice = "It's hot! Wear light clothing."
elif 60 <= fahrenheit < 80:
advice = "It's warm. Wear comfortable clothing."
else:
advice = "It's cold! Wear warm clothing."
Converting Fahrenheit to Celsius
The core functionality of the assignment is converting the temperature from Fahrenheit to Celsius. This can be achieved using a simple mathematical formula.
The Conversion Formula
The formula to convert Fahrenheit to Celsius is:
Celsius=59×(Fahrenheit−32)\text{Celsius} = \frac{5}{9} \times (\text{Fahrenheit} - 32)Celsius=95×(Fahrenheit−32)
Implementing the Conversion Function
To keep your code organized, implement the conversion logic in a function:
def fahrenheit_to_celsius(fahrenheit):
return (5/9) * (fahrenheit - 32)
Displaying the Result
Once you have the converted temperature and clothing advice, the next step is to display the results to the user.
Formatting the Output
Use formatted strings to present the results clearly:
celsius = fahrenheit_to_celsius(fahrenheit)
print(f"The equivalent temperature in Celsius is {celsius:.2f} degrees.")
print(advice)
Repeating the Process
Finally, to meet the requirement of asking the user if they have another temperature to convert, use a loop that repeats the process based on user input.
Using a Loop
A while loop can be used to repeat the process until the user decides to stop:
while True:
# Code to get input, provide advice, convert temperature, and display result
another_conversion = input("Do you have another temperature to convert? (yes/no): ").lower()
if another_conversion != 'yes':
break
Testing and Debugging
Testing and debugging are critical steps in ensuring your program works correctly and handles all edge cases.
Writing Test Cases
Create test cases to cover a variety of scenarios, including edge cases. For example, test temperatures at the boundary of each category (cold, warm, hot) and ensure the program handles invalid inputs gracefully.
Testing Edge Cases
Some edge cases to consider:
- Extremely high or low temperatures
- Non-numeric input
- Boundary values (e.g., exactly 60°F or 80°F)
Using Print Statements and Debugging Tools
If your program isn’t working as expected, use print statements or debugging tools to trace the problem. Print statements can help you understand the flow of your program and identify where it’s going wrong.
Example Debugging
For instance, if the clothing advice isn’t displaying correctly, add print statements to check the value of fahrenheit and the branch of the conditional statement being executed:
print(f"Temperature entered: {fahrenheit}")
if fahrenheit >= 80:
advice = "It's hot! Wear light clothing."
print("Advice: Hot")
elif 60 <= fahrenheit < 80:
advice = "It's warm. Wear comfortable clothing."
print("Advice: Warm")
else:
advice = "It's cold! Wear warm clothing."
print("Advice: Cold")
Optimizing and Refining Your Code
After ensuring your program works correctly, look for opportunities to optimize and refine your code. This step involves improving efficiency, readability, and maintainability.
Improving Efficiency
Consider whether there are any parts of your code that can be made more efficient. For example, if you find yourself repeating code, look for ways to consolidate or refactor it.
Refactoring Repeated Code
In the example provided, the code for displaying results and prompting the user for another conversion is repeated. This can be moved into a function to reduce repetition:
def get_user_input():
while True:
try:
return float(input("Enter temperature in Fahrenheit: "))
except ValueError:
print("Please enter a valid number.")
def display_results(fahrenheit):
celsius = fahrenheit_to_celsius(fahrenheit)
if fahrenheit >= 80:
advice = "It's hot! Wear light clothing."
elif 60 <= fahrenheit < 80:
advice = "It's warm. Wear comfortable clothing."
else:
advice = "It's cold! Wear warm clothing."
print(f"The equivalent temperature in Celsius is {celsius:.2f} degrees.")
print(advice)
Enhancing Readability
Ensure your code is easy to read and understand. Use meaningful variable names, add comments to explain complex logic, and organize your code into functions.
Adding Comments
Comments help others (and your future self) understand your code. Add comments to explain the purpose of each section and any non-obvious logic:
def fahrenheit_to_celsius(fahrenheit):
# Convert Fahrenheit to Celsius using the formula
return (5/9) * (fahrenheit - 32)
while True:
# Get temperature input from user
fahrenheit = get_user_input()
# Display results and advice
display_results(fahrenheit)
# Ask user if they have another temperature to convert
another_conversion = input("Do you have another temperature to convert? (yes/no): ").lower()
if another_conversion != 'yes':
break
Organizing Code into Functions
Organize your code into functions to make it modular and easier to maintain. Each function should have a single responsibility, making your code more readable and easier to debug.
Example of Modular Code
Here’s an example of the complete program with functions:
def fahrenheit_to_celsius(fahrenheit):
return (5/9) * (fahrenheit - 32)
def get_user_input():
while True:
try:
return float(input("Enter temperature in Fahrenheit: "))
except ValueError:
print("Please enter a valid number.")
def display_results(fahrenheit):
celsius = fahrenheit_to_celsius(fahrenheit)
if fahrenheit >= 80:
advice = "It's hot! Wear light clothing."
elif 60 <= fahrenheit < 80:
advice = "It's warm. Wear comfortable clothing."
else:
advice = "It's cold! Wear warm clothing."
print(f"The equivalent temperature in Celsius is {celsius:.2f} degrees.")
print(advice)
def main():
while True:
fahrenheit = get_user_input()
display_results(fahrenheit)
another_conversion = input("Do you have another temperature to convert? (yes/no): ").lower()
if another_conversion != 'yes':
break
if __name__ == "__main__":
main()
Conclusion
By breaking down the problem and systematically addressing each requirement, you can tackle similar programming assignments with confidence. Remember to test thoroughly, refine your code for efficiency and readability, and make use of functions to keep your code organized and maintainable.