How to Successfully Solve Circuit Puzzle Assignments in Haskell
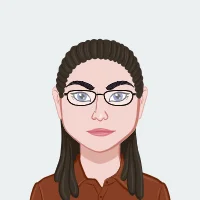
Circuit puzzles, such as the one described, require a systematic approach to ensure all the wires connect and form a complete circuit. If you're looking to do my Haskell assignment, this step-by-step guide will help you tackle similar programming assignments effectively. By breaking down the problem, implementing necessary functions, and thoroughly testing your solution, you can confidently complete any circuit puzzle. Whether you're working on a class project or just trying to improve your Haskell skills, this guide provides the tools and techniques needed to do your Haskell assignment successfully and efficiently.
Understanding the Problem Statement
Circuit puzzles, as introduced in the given programming assignment, are engaging challenges where the solver is presented with a grid of tiles, each having wires printed on them. The goal is to rotate each tile so that all the wires connect together, forming a complete circuit. These puzzles not only test your programming skills but also your problem-solving and logical thinking abilities.
1. Analyzing the Puzzle Structure
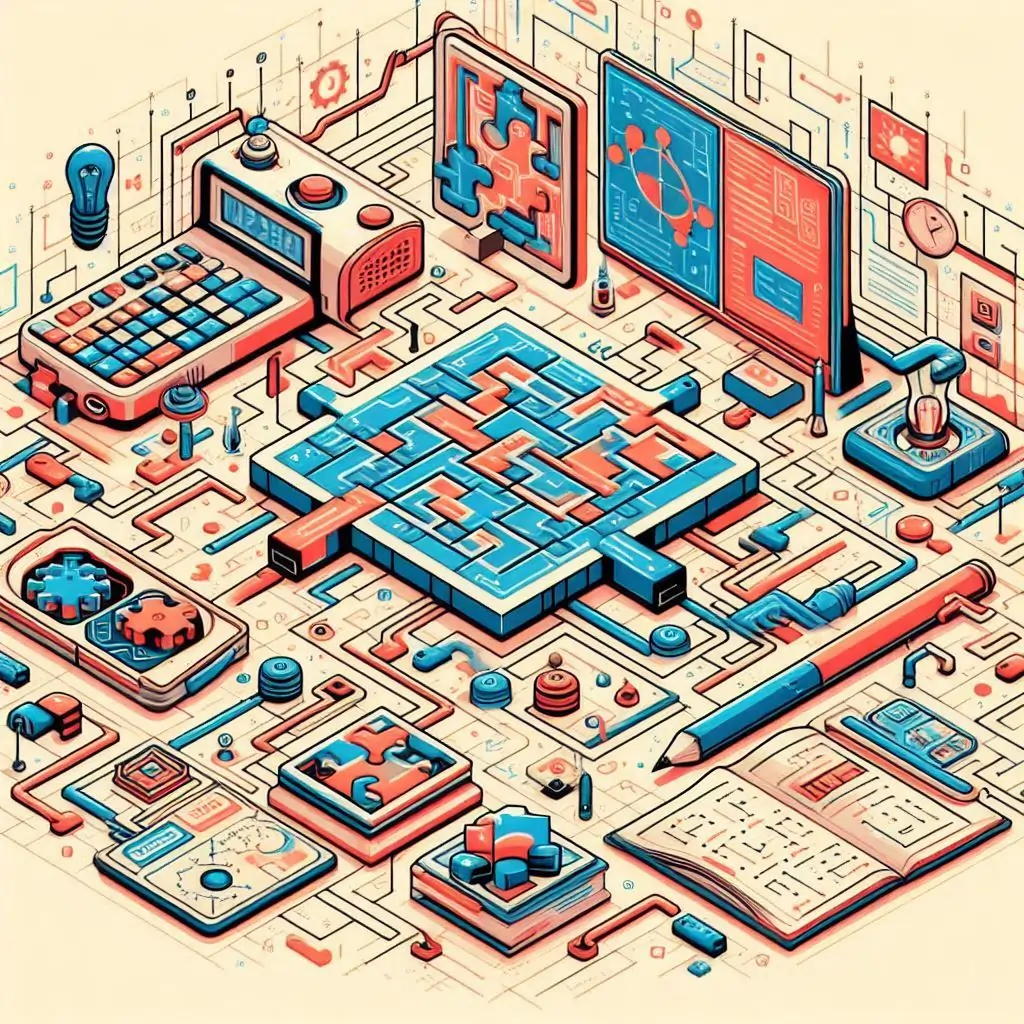
Circuit puzzles consist of various types of tiles: Source, Sink, and Wire. Each tile can have connectors on its edges, and these connectors determine how the tiles can be rotated and connected to form a complete circuit. Understanding the structure and behavior of these tiles is crucial to solving the puzzle.
2. Visualizing the Problem
Visualization is a powerful tool when tackling circuit puzzles. Drawing out the grid and the connections can help you better understand how the tiles should be rotated to form a complete circuit. By visualizing, you can see the paths from sources to sinks and ensure that all wires are properly connected.
3. Breaking Down the Problem
Before diving into the coding part, it's essential to break down the problem into smaller, manageable tasks. Identify the key functions needed to solve the puzzle, such as checking the completeness of the circuit and rotating the tiles. This step-by-step approach will help you create a structured solution.
Implementing Helper Functions
To solve circuit puzzles, you'll need several helper functions. These functions will handle tasks such as rotating tiles and checking connections between tiles. Implementing these helper functions will make it easier to solve the overall puzzle.
1. Rotating Tiles
One of the fundamental operations in solving circuit puzzles is rotating the tiles. Each tile can be rotated by 90, 180, or 270 degrees. Implementing a function to handle these rotations is the first step in creating a solution.
Function to Rotate a Tile
Here's an example of a Haskell function to rotate a tile:
rotateTile :: Tile -> Rotation -> Tile
rotateTile tile R0 = tile
rotateTile tile R90 = rotate90 tile
rotateTile tile R180 = rotate90 (rotate90 tile)
rotateTile tile R270 = rotate90 (rotate90 (rotate90 tile))
Function to Rotate Edges
The rotateTile function relies on a helper function to rotate the edges of a tile:
rotateEdge90 :: Edge -> Edge
rotateEdge90 North = East
rotateEdge90 East = South
rotateEdge90 South = West
rotateEdge90 West = North
2. Checking Connections
After rotating the tiles, you need to check if they are connected properly. This involves verifying if adjacent tiles have matching connectors. Implementing a function to check these connections is crucial for solving the puzzle.
Function to Check Connections
Here's an example function to check if two tiles are connected:
isConnected :: Tile -> Tile -> Bool
isConnected (Wire edges1) (Wire edges2) = any (`elem` edges2) edges1
isConnected _ _ = False
3. Creating a Grid
To solve the puzzle, you'll need to create a grid of tiles and apply rotations to them. Implementing a function to create and manipulate this grid will help you apply the rotations and check the connections.
Function to Create a Grid
Here's an example function to create a grid of tiles:
createGrid :: Int -> Int -> [[Tile]]
createGrid rows cols = replicate rows (replicate cols (Wire []))
Main Functions
With the helper functions in place, you can now focus on implementing the main functions to solve the circuit puzzle. These functions will check the completeness of the puzzle and find a solution by rotating the tiles.
1. Checking Puzzle Completeness
The first main function you need is one that checks if the puzzle is complete. This function will ensure that all wires are connected and that there is a path from each Source tile to at least one Sink tile, and vice-versa.
Function to Check Puzzle Completeness
Here's an example function to check if the puzzle is complete:
isPuzzleComplete :: Puzzle -> Bool
isPuzzleComplete puzzle = allTilesConnected && allSourcesReachable && allSinksReachable
where
allTilesConnected = all (uncurry isConnected) (adjacentTiles puzzle)
allSourcesReachable = all (reachableFromAny sourceTiles) sinkTiles
allSinksReachable = all (reachableFromAny sinkTiles) sourceTiles
sourceTiles = filter isSource (concat puzzle)
sinkTiles = filter isSink (concat puzzle)
2. Solving the Puzzle
The second main function you need is one that solves the puzzle. This function will try different rotations for each tile and check if the resulting puzzle is complete. If a solution is found, it returns the rotations; otherwise, it returns Nothing.
Function to Solve the Puzzle
Here's an example function to solve the puzzle:
solveCircuit :: Puzzle -> Maybe [[Rotation]]
solveCircuit puzzle = findSolution (allPossibleRotations puzzle)
where
findSolution = find (isPuzzleComplete . applyRotations puzzle)
allPossibleRotations = sequence (map (map allRotations))
allRotations tile = [R0, R90, R180, R270]
Testing and Validation
Testing your solution is crucial to ensure it works correctly. Use the provided examples to validate your functions, and create additional test cases to handle edge cases and ensure robustness.
1. Using Provided Examples
Start by testing your solution with the examples provided in the assignment. This will help you verify that your functions are working as expected.
Example Test Case
Here's an example test case:
main = do
let puzzle = [
[ Wire [North, West], Wire [North, South], Source [North] ],
[ Wire [North, West], Wire [East, West], Wire [North, East] ],
[ Sink [West], Wire [North, South], Wire [North, West] ]
]
print $ solveCircuit puzzle
2. Creating Additional Test Cases
To ensure your solution is robust, create additional test cases. Consider edge cases such as puzzles with no connections or puzzles with multiple sources and sinks.
Additional Test Case
Here's an example of an additional test case:
main = do
let puzzle = [
[ Source [North], Wire [North, South], Sink [South] ],
[ Wire [East, West], Wire [East, West], Wire [East, West] ],
[ Source [East], Wire [North, South], Sink [West] ]
]
print $ solveCircuit puzzle
Optimization
After implementing and testing your solution, consider optimizing it for better performance. Analyzing your solution for efficiency and scalability will ensure it handles larger puzzles effectively.
1. Improving Efficiency
Identify any redundant computations in your solution and optimize them. For instance, avoid recalculating connections and rotations multiple times.
Optimized Check Connections Function
Here's an optimized version of the isConnected function:
isConnected :: Tile -> Tile -> Bool
isConnected (Wire edges1) (Wire edges2) = not (null (edges1 `intersect` edges2))
isConnected _ _ = False
2. Handling Larger Puzzles
Ensure your solution scales well with larger grid sizes. Test your solution with larger puzzles to verify its performance and efficiency.
Testing with Larger Puzzles
Here's an example of testing with a larger puzzle:
main = do
let puzzle = replicate 10 (replicate 10 (Wire [North, East, South, West]))
print $ solveCircuit puzzle
Conclusion
Solving circuit puzzles involves a systematic approach, breaking down the problem, implementing helper functions, and creating the main functions to check the completeness and find a solution. By following these steps, you can effectively tackle any similar programming assignment. By approaching the problem methodically and testing your solution thoroughly, you can ensure your program works correctly and efficiently. If you need additional support, a programming assignment helper can provide expert guidance and assistance.