How to Build and Test Responsive Web Pages
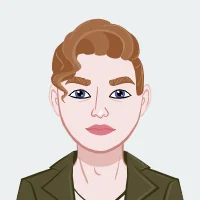
Creating a responsive website can seem daunting, but with a structured approach, you can build and test a site that works seamlessly across various devices. Here’s a guide to help you tackle assignments like the one outlined and complete your web development assignment, ensuring you develop the skills needed for similar projects. This guide will help you tackle programming assignments like the one outlined, ensuring you develop the skills needed for similar projects.
Implementation
Building a website involves a series of steps that must be carefully planned and executed. In this section, we'll discuss how to create responsive web pages using HTML5 and CSS, and how to implement the specific requirements of your assignment.
Structuring Your Web Pages with HTML5
The foundation of any website is its structure, which is built using HTML5. This modern markup language provides semantic elements that help organize your content in a meaningful way.
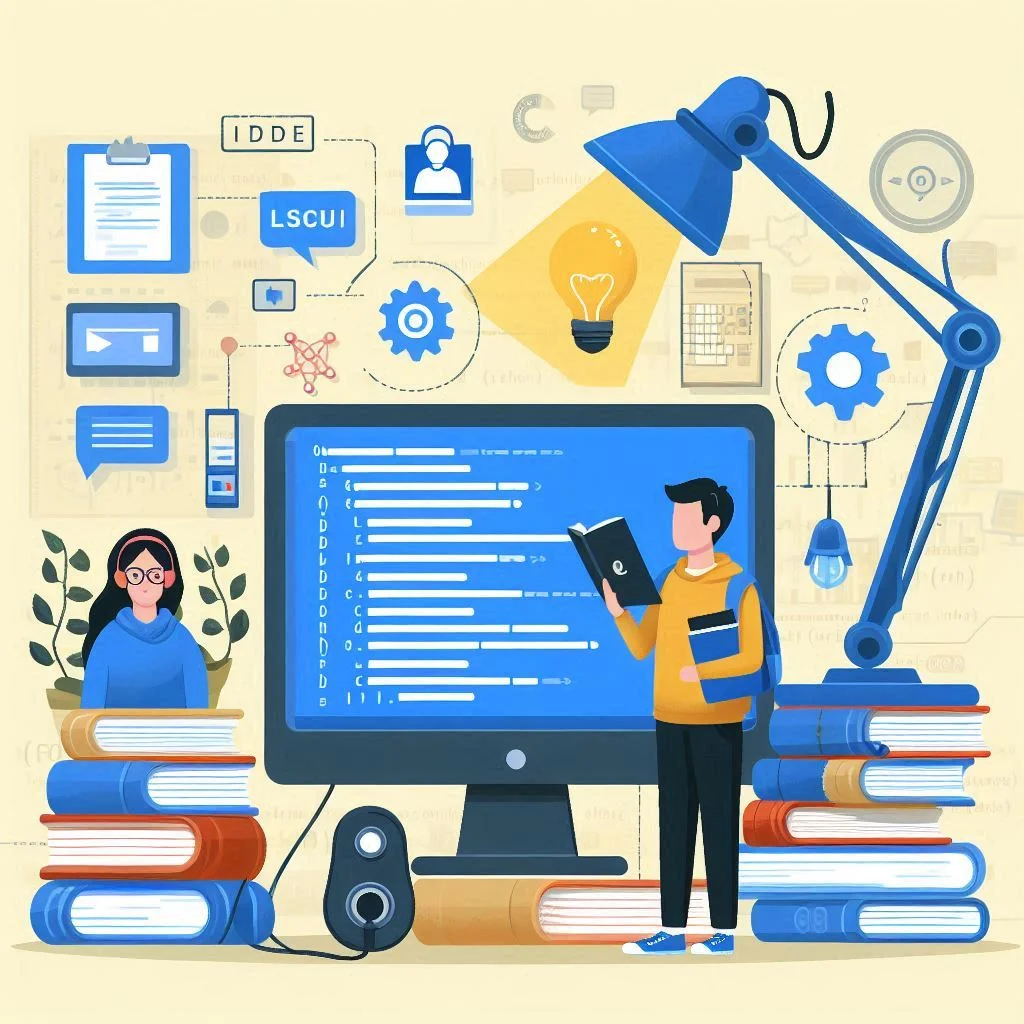
Using Semantic Elements
HTML5 introduces several semantic elements that replace generic < div > elements, making your HTML more readable and easier to maintain. These elements include < header >, < nav >, < section >, < article >, and < footer >.
Example:
< !DOCTYPE html >
< html lang="en" >
< head >
< meta charset="UTF-8" >
< meta name="viewport" content="width=device-width, initial-scale=1.0" >
< title >Website Title< /title >
< link rel="stylesheet" href="styles.css" >
< /head >
< body >
< header >
< nav >
< img src="logo.png" alt="Logo" >
< ul >
< li >< a href="index.html">Home< /a >< /li >
< li >< a href="tour.html" >Tour< /a >< /li >
< li >< a href="contact.html" >Contact< /a >< /li >
< /ul >
< /nav >
< /header >
< main >
< !-- Content goes here -- >
< /main >
< footer >
< p >Contact us: [email protected] | 123-456-7890< /p >
< /footer >
< /body >
< /html >
Organizing Your Content
Using semantic elements helps search engines understand your content better, improving your website's SEO. It also makes your code more accessible, as screen readers can better navigate the content.
Styling with CSS Flexbox and Grid
CSS is used to style your HTML content and make it visually appealing. Flexbox and Grid are powerful CSS modules that help create responsive layouts.
Flexbox for One-Dimensional Layouts
Flexbox is ideal for arranging elements in a single dimension, either horizontally or vertically. It's particularly useful for creating navigation bars, aligning items, and distributing space within a container.
Example:
nav {
display: flex;
justify-content: space-between;
align-items: center;
}
nav ul {
display: flex;
list-style-type: none;
}
nav li {
margin-right: 20px;
}
Grid for Two-Dimensional Layouts
CSS Grid is perfect for more complex, two-dimensional layouts. It allows you to create grids with rows and columns, making it easier to design web pages with varying content.
Example:
.grid-container {
display: grid;
grid-template-columns: 1fr 1fr 1fr;
gap: 10px;
}
Making Images Responsive
Responsive images adjust their size according to the screen they are being viewed on. Use the max-width property to ensure images do not overflow their containers.
Example:
img {
max-width: 100%;
height: auto;
}
Creating Forms
Forms are an essential part of any website, allowing users to interact and submit information. Here’s an example of a simple contact form:
Example:
< form action="submit_form.php" method="post" >
< label for="name" >Name:< /label >
< input type="text" id="name" name="name" required >
< label for="email" >Email:< /label >
< input type="email" id="email" name="email" required >
< label for="message">Message:< /label >
< textarea id="message" name="message" required >< /textarea >
< button type="submit" >Send< /button >
< /form >
Testing
Testing is a crucial phase in web development to ensure your website functions correctly across different devices and browsers. This section will guide you through the testing process.
Testing Responsiveness Across Major Browsers
Your website should load and behave correctly in the latest versions of major browsers like Chrome and Firefox. This ensures that users have a consistent experience regardless of their browser choice.
Using Developer Tools
Both Chrome and Firefox offer developer tools that allow you to test your website's responsiveness. These tools enable you to emulate different devices and screen sizes, helping you identify and fix layout issues.
Chrome Developer Tools:
- Open your website in Chrome.
- Right-click and select "Inspect" or press Ctrl+Shift+I.
- Click the "Toggle device toolbar" icon or press Ctrl+Shift+M.
- Choose different devices and screen sizes from the toolbar.
Firefox Developer Tools:
- Open your website in Firefox.
- Right-click and select "Inspect" or press Ctrl+Shift+I.
- Click the "Responsive Design Mode" icon or press Ctrl+Shift+M.
- Select different devices and screen sizes from the toolbar.
Real Devices
If possible, test your website on actual devices, including laptops, tablets, and mobile phones. This gives you a more accurate representation of how your site will perform for real users.
Documenting Screenshots
Documenting your testing process is important for showcasing your work and for future reference. Take screenshots of each page on different devices and compile them in a Word document.
Taking Screenshots
- Use the developer tools to switch between devices.
- Capture screenshots of each page (Home, Tour, Contact) on laptop, tablet, and mobile views.
- Save these screenshots with descriptive filenames (e.g., home-laptop.png, tour-tablet.png).
Compiling Screenshots
- Open a new Word document.
- Insert each screenshot with a brief description.
- 3rganize the document logically, grouping screenshots by device and browser.
Best Practices for Building Responsive Websites
In addition to the technical steps outlined above, following best practices will help you create high-quality, responsive websites that offer a great user experience.
Mobile-First Design
Start designing your website for mobile devices first, then scale up for larger screens. This ensures your site is optimized for the majority of users, as mobile browsing continues to grow.
Why Mobile-First?
- Performance: Mobile-first design often leads to faster loading times and better performance on all devices.
- User Experience: Prioritizing mobile users ensures your site is accessible and easy to navigate on small screens.
- SEO: Search engines favor mobile-friendly websites, improving your site's search rankings.
Use of Media Queries
Media queries in CSS allow you to apply different styles based on the screen size and other characteristics of the user's device. This is key to creating responsive designs.
Example:
/* Base styles */
body {
font-family: Arial, sans-serif;
margin: 0;
padding: 0;
}
/* Mobile styles */
@media (max-width: 600px) {
nav ul {
flex-direction: column;
}
.grid-container {
grid-template-columns: 1fr;
}
}
/* Tablet styles */
@media (min-width: 601px) and (max-width: 1200px) {
.grid-container {
grid-template-columns: 1fr 1fr;
}
}
/* Desktop styles */
@media (min-width: 1201px) {
.grid-container {
grid-template-columns: 1fr 1fr 1fr;
}
}
Testing Tools
Various tools are available to help you test and optimize your responsive design. These tools can automate parts of the testing process and provide insights into your site's performance.
Popular Testing Tools
- Google Mobile-Friendly Test: Checks if your site is mobile-friendly and provides recommendations for improvement.
- BrowserStack: Allows you to test your site across a wide range of browsers and devices.
- Responsinator: Quickly previews how your site looks on popular devices.
Common Challenges and How to Overcome Them
Building responsive websites can present several challenges. Understanding these challenges and knowing how to address them is crucial for successful web development.
Handling Complex Layouts
Complex layouts can be difficult to manage with responsive design. CSS Grid is particularly useful for creating intricate layouts that adjust well to different screen sizes.
Tips:
- Plan Your Layout: Sketch your layout on paper before coding to visualize how it will adapt to different screens.
- Use Grid Areas: Define grid areas in your CSS to simplify the placement of elements.
Example:
.grid-container {
display: grid;
grid-template-areas:
'header header header'
'sidebar main main'
'footer footer footer';
gap: 10px;
}
.header {
grid-area: header;
}
.sidebar {
grid-area: sidebar;
}
.main {
grid-area: main;
}
.footer {
grid-area: footer;
}
Ensuring Cross-Browser Compatibility
Different browsers may render your site differently, causing inconsistencies in layout and functionality. Testing across multiple browsers is essential to ensure a consistent user experience.
Tips:
- Use CSS Resets: Apply a CSS reset to normalize styles across browsers.
- Test Regularly: Regularly test your site in all major browsers during development.
Optimizing Performance
Responsive websites can sometimes suffer from performance issues, especially on mobile devices. Optimizing your site's performance ensures a smooth user experience.
Tips:
- Minimize HTTP Requests: Reduce the number of HTTP requests by combining CSS and JavaScript files.
- Optimize Images: Compress images and use responsive image techniques to reduce load times.
- Enable Caching: Leverage browser caching to speed up subsequent visits to your site.
Conclusion
Building and testing responsive web pages involves careful planning and attention to detail. By following this comprehensive guide, you can create a website that not only meets the assignment requirements but also provides a great user experience across various devices. Keep practicing these skills, and you’ll be well-prepared for similar assignments in the future.