Effective Strategies for Implementing a Queue and Simulating Hot Potato
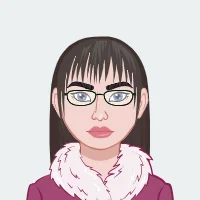
Building a Queue and Playing Hot Potato Programming assignments often pose challenges but approaching them methodically enhances your ability to succeed. This guide provides a step-by-step approach to mastering tasks such as constructing a queue with a linked list and simulating the "Hot Potato" game. While referencing a sample assignment, the principles discussed here are universally applicable, empowering you to navigate similar programming challenges confidently. Understanding the fundamentals of data structures like queues and mastering algorithms for simulations not only fulfills assignment requirements but also builds foundational skills crucial for broader programming endeavors. This guide will walk you through solving assignments that involve building a queue using a linked list and simulating the "Hot Potato" game. While we’ll refer to a specific sample Data Structure assignment, the principles and steps outlined here will equip you to handle similar tasks.
Implementing the Queue with a Linked List
A queue is a fundamental data structure following the First-In-First-Out (FIFO) principle. In this section, we’ll focus on implementing a queue using a linked list.
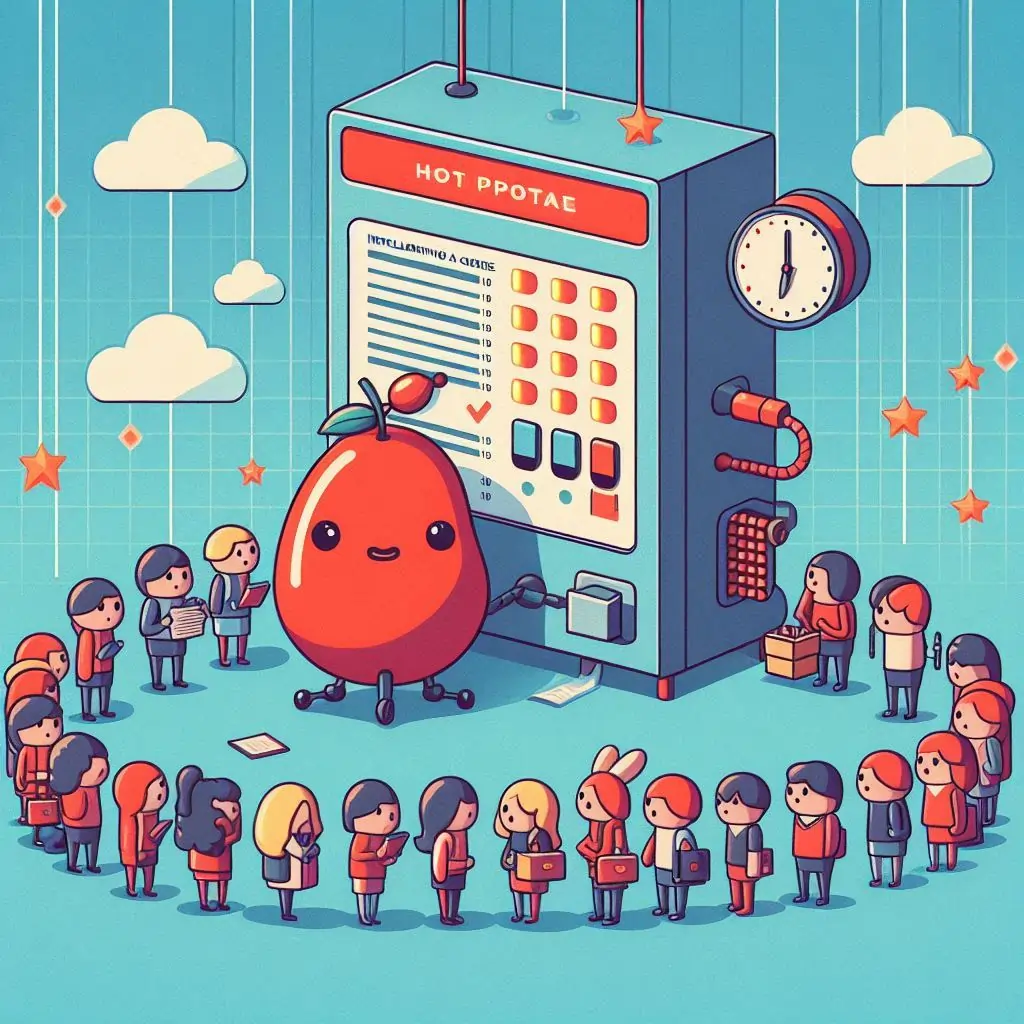
Designing the Queue
A queue allows adding elements to the end (enqueue) and removing elements from the front (dequeue). Here’s how to design it using a linked list.
Node Structure
First, define a node structure. Each node will contain the data (a string in this case) and a pointer to the next node.
class Node {
public:
std::string data;
Node* next;
Node(std::string val) : data(val), next(nullptr) {}
};
Queue Class
Next, create a class for the queue. It will have pointers to the front and rear of the linked list and functions to manage the queue operations.
class Queue {
private:
Node* front;
Node* rear;
public:
Queue() : front(nullptr), rear(nullptr) {}
~Queue();
Queue(const Queue& other);
Queue& operator=(const Queue& other);
void enqueue(const std::string& str);
void dequeue();
std::string front() const;
bool isEmpty() const;
};
Implementing Queue Operations
With the basic structure in place, implement the operations: constructor, destructor, copy constructor, assignment operator, enqueue, dequeue, front, and isEmpty.
Constructor and Destructor
The constructor initializes an empty queue, while the destructor ensures proper memory management by deallocating all nodes.
Queue::Queue() : front(nullptr), rear(nullptr) {}
Queue::~Queue() {
while (front != nullptr) {
Node* temp = front;
front = front->next;
delete temp;
}
}
Enqueue Operation
The enqueue function adds a new node to the rear of the queue.
void Queue::enqueue(const std::string& str) {
Node* newNode = new Node(str);
if (rear != nullptr) {
rear->next = newNode;
}
rear = newNode;
if (front == nullptr) {
front = rear;
}
}
Dequeue Operation
The dequeue function removes the front node. If the queue is empty, it throws an exception.
void Queue::dequeue() {
if (isEmpty()) {
throw std::runtime_error("QueueEmptyException");
}
Node* temp = front;
front = front->next;
if (front == nullptr) {
rear = nullptr;
}
delete temp;
}
Front Operation
The front function returns the data of the front node. It throws an exception if the queue is empty.
std::string Queue::front() const {
if (isEmpty()) {
throw std::runtime_error("QueueEmptyException");
}
return front->data;
}
Copy Constructor and Assignment Operator
Ensure deep copying to handle copying queues properly.
Queue::Queue(const Queue& other) : front(nullptr), rear(nullptr) {
Node* current = other.front;
while (current != nullptr) {
enqueue(current->data);
current = current->next;
}
}
Queue& Queue::operator=(const Queue& other) {
if (this == &other) return *this;
while (!isEmpty()) {
dequeue();
}
Node* current = other.front;
while (current != nullptr) {
enqueue(current->data);
current = current->next;
}
return *this;
}
bool Queue::isEmpty() const {
return front == nullptr;
}
Testing Your Queue Implementation
Before moving on to the next part of the assignment, test your queue implementation thoroughly. Create test cases to verify each function, ensuring they handle edge cases correctly.
Simulating the Hot Potato Game
The "Hot Potato" game involves a group of participants passing an object (the potato) until one person remains. This part of the assignment requires simulating the game using your queue implementation.
Setting Up the Simulation
Start by setting up the simulation environment.
Reading Input
Read the names of participants from a file into the queue. Each line in the file represents a participant.
void readParticipants(std::istream& in, Queue& players) {
std::string name;
while (std::getline(in, name)) {
players.enqueue(name);
}
}
Handling the Game Logic
The game progresses in rounds. Each round, the potato is passed a specified number of times, and the player left holding the potato is eliminated.
std::string playHotPotato(std::istream& in, const std::vector
& numOfPasses) {
Queue players;
readParticipants(in, players);
unsigned passIndex = 0;
while (players.size() > 1) {
unsigned passes = numOfPasses[passIndex];
for (unsigned i = 0; i < passes; ++i) {
players.enqueue(players.front());
players.dequeue();
}
players.dequeue();
passIndex = (passIndex + 1) % numOfPasses.size();
}
return players.front();
}
Breaking Down the Simulation
Initializing Participants
Use a queue to store the participants in the order they are read from the file.
Queue players;
readParticipants(in, players);
Simulating Rounds
Use a loop to simulate each round of the game. In each round, pass the potato the specified number of times and then eliminate the player holding the potato.
unsigned passIndex = 0;
while (players.size() > 1) {
unsigned passes = numOfPasses[passIndex];
for (unsigned i = 0; i < passes; ++i) {
players.enqueue(players.front());
players.dequeue();
}
players.dequeue();
passIndex = (passIndex + 1) % numOfPasses.size();
}
Determining the Winner
The last player remaining in the queue is the winner of the game.
return players.front();
Testing the Simulation
Create test cases to ensure your simulation works correctly. Test with different numbers of participants and various pass patterns to verify the logic.
General Tips for Solving Programming Assignments
Successfully completing programming assignments requires more than just coding skills. Here are some tips to help you approach and solve assignments effectively.
Plan Before Coding
Planning is Key: Before you write any code, plan your approach. Understand the problem, design your solution, and break it down into smaller, manageable parts.
Sketch Out Your Solution
Draw diagrams or write pseudocode to outline your solution. This helps you visualize the data flow and logic before implementing it in code.
Understand the Requirements
Make sure you understand every requirement of the assignment. Clarify any ambiguities before starting to code.
Write Modular Code
Modularity: Write your code in small, reusable functions. This makes it easier to debug, test, and maintain.
Functions and Classes
Divide your code into functions and classes, each responsible for a specific part of the solution. This modular approach enhances readability and reusability.
Code Reusability
Look for opportunities to reuse code. For instance, if you have written file I/O code in previous assignments, consider reusing it here with necessary modifications.
Test Thoroughly
Testing: Thoroughly test your code to ensure it works correctly in all scenarios.
Create Test Cases
Write test cases for each function and test them individually. Ensure your tests cover edge cases and typical usage scenarios.
Debugging
Use debugging tools and techniques to identify and fix issues. Step through your code to understand its behavior and identify any logical errors.
Handle Errors Gracefully
Error Handling: Implement proper error handling to make your code robust and user-friendly.
Exception Handling
Use exceptions to handle errors gracefully. Ensure your code does not crash unexpectedly and provides meaningful error messages.
Input Validation
Validate inputs to your functions to prevent invalid data from causing errors. This helps in maintaining the integrity of your program.
Comment Your Code
Documentation: Comment your code to explain its purpose and logic. Good comments make your code easier to understand and maintain.
Explain Your Logic
Use comments to explain complex logic and the purpose of specific code segments. This is helpful for both you and others who may read your code later.
Keep Comments Updated
Ensure your comments remain accurate as your code evolves. Outdated comments can be misleading and counterproductive.
By following these steps and tips, you’ll be well-equipped to tackle programming assignments involving data structures, algorithms, and simulations. Whether you're building a queue or simulating a game, a structured approach will help you solve the problem efficiently and effectively.