Building a Board Game Model in Java
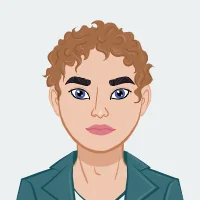
Creating a board game model for a programming assignment can be a rewarding yet challenging task. This guide provides a detailed, step-by-step approach to help you design and implement a board game model in Java. Although inspired by a specific board game development project, the principles and methods discussed here are applicable to a wide range of similar assignments. Whether you are looking for guidance to complete your Java assignment or need a comprehensive understanding of board game development, this guide covers all the essential steps.
Step 1: Understanding the Requirements
Before diving into the design and coding process, it's crucial to thoroughly understand the assignment's requirements. Breaking down the assignment into smaller, manageable tasks will make the process more manageable and structured.
Understanding the World Description
The world description is the foundation of your game model. It includes several key elements:
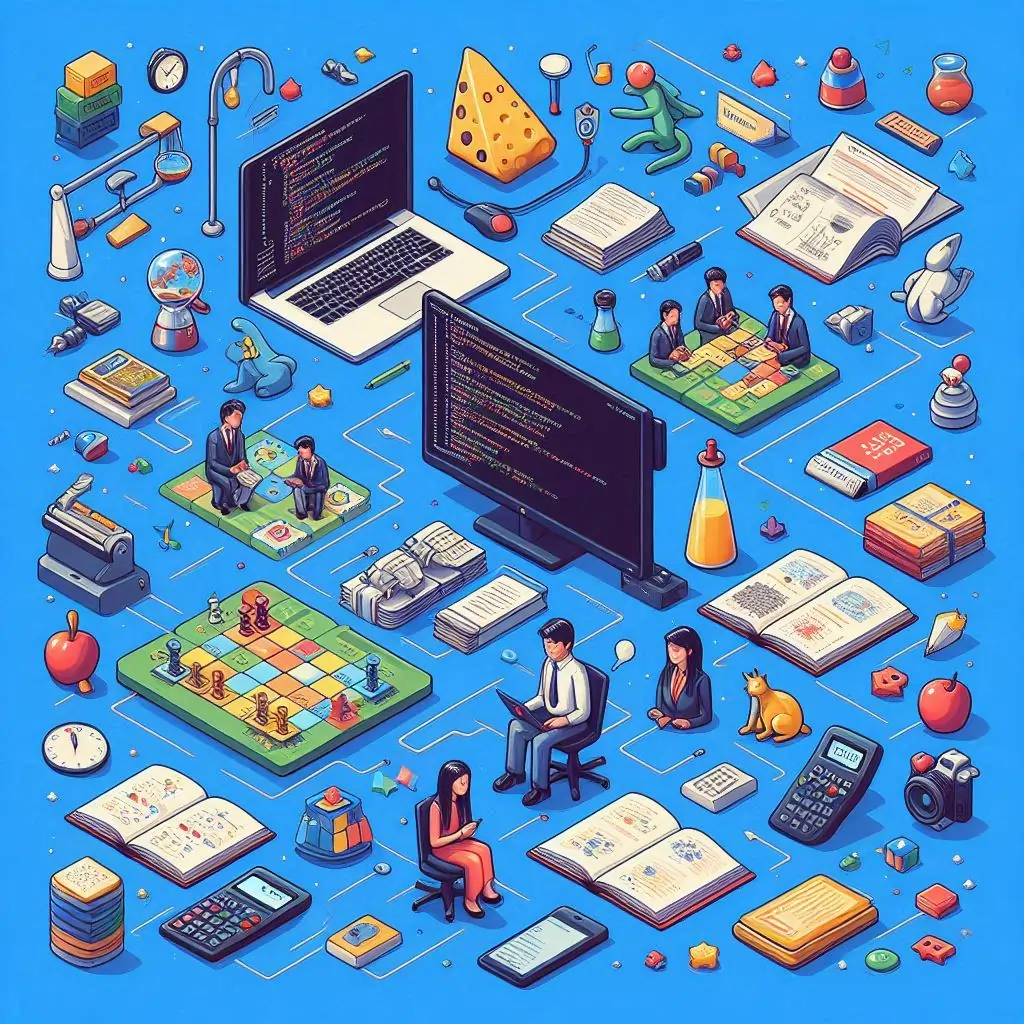
Understanding the World Description
The world description is the foundation of your game model. It includes several key elements:
- Size of the World: The number of rows and columns.
- World Name: A unique identifier for the world.
- Target Character: The character that players will interact with, including their health and name.
Spaces or Rooms
Spaces or rooms are essential components of the game world. They are laid out on a 2D grid and must be non-overlapping. Each space or room has specific attributes:
- Location: Defined by the coordinates of the upper left and lower right corners.
- Name: A unique identifier for each space.
Items
Items add an interactive element to the game. Each item has specific attributes and is associated with a space:
- Location: The index of the room where the item is found.
- Damage: The amount of damage the item can inflict if used to attack the target character.
- Name: A unique identifier for each item.
Step 2: Designing the Model
Designing the model involves defining the classes, their attributes, methods, and the relationships between them. This step ensures you have a clear blueprint before coding.
Classes and Interfaces
Identify and define the core classes and interfaces required for the game model:
- World: Represents the entire game world.
- Space: Represents a space or room in the world.
- Item: Represents an item found in a space.
- Character: Represents the target character.
Attributes and Methods
Specify the attributes and methods for each class to capture the required functionalities:
World Class
public class World {
private String name;
private int rows;
private int columns;
private List
spaces;
private List
-
items;
private Character targetCharacter;
public World(String name, int rows, int columns) {
this.name = name;
this.rows = rows;
this.columns = columns;
this.spaces = new ArrayList<>();
this.items = new ArrayList<>();
}
// Methods to add spaces, items, get neighbors, etc.
}
Space Class
public class Space {
private String name;
private Point upperLeft;
private Point lowerRight;
public Space(String name, Point upperLeft, Point lowerRight) {
this.name = name;
this.upperLeft = upperLeft;
this.lowerRight = lowerRight;
}
// Methods to check if space contains item, get neighbors, etc.
}
Item Class
public class Item {
private String name;
private int damage;
private int spaceIndex;
public Item(String name, int damage, int spaceIndex) {
this.name = name;
this.damage = damage;
this.spaceIndex = spaceIndex;
}
// Methods to get item details
}
Character Class
public class Character {
private String name;
private int health;
private Space currentSpace;
public Character(String name, int health, Space currentSpace) {
this.name = name;
this.health = health;
this.currentSpace = currentSpace;
}
// Methods to move character, reduce health, etc.
}
UML Diagram
A UML (Unified Modeling Language) diagram helps visualize the design. It should include all classes, attributes, methods, and relationships, ensuring you have a clear blueprint before coding.
Writing a Testing Plan
A thorough testing plan ensures your design works as expected. It should cover all scenarios, including unit tests, integration tests, and edge cases. Specify the conditions, example data, and expected results for each test.
Unit Tests
Test individual methods in isolation to ensure they function correctly.
Integration Tests
Test how methods work together to ensure the overall system functions correctly.
Edge Cases
Test unusual or extreme inputs to ensure the robustness of your design.
Step 3: Implementation
Once you have a clear design, you can start coding. Implement the classes and methods as per your design. Keep your code well-documented with comments explaining the purpose and usage of each class and method.
Implementing the World Class
The World class is the backbone of your game model. It should include methods to add spaces and items, get neighbors, move the target character, and display information about a space.
public class World {
private String name;
private int rows;
private int columns;
private List
spaces;
private List
-
items;
private Character targetCharacter;
public World(String name, int rows, int columns) {
this.name = name;
this.rows = rows;
this.columns = columns;
this.spaces = new ArrayList<>();
this.items = new ArrayList<>();
}
public void addSpace(Space space) {
spaces.add(space);
}
public void addItem(Item item) {
items.add(item);
}
public List
getNeighbors(Space space) {
// Logic to find neighboring spaces
}
public void moveCharacter(Space space) {
targetCharacter.setCurrentSpace(space);
}
public String getSpaceInfo(Space space) {
// Logic to display information about a space
}
}
Implementing the Space Class
The Space class represents a space or room in the game world. It should include methods to check if the space contains an item and get neighboring spaces.
public class Space {
private String name;
private Point upperLeft;
private Point lowerRight;
public Space(String name, Point upperLeft, Point lowerRight) {
this.name = name;
this.upperLeft = upperLeft;
this.lowerRight = lowerRight;
}
public boolean containsItem(Item item) {
// Logic to check if the space contains the item
}
public List
getNeighborSpaces() {
// Logic to find neighboring spaces
}
}
Implementing the Item Class
The Item class represents an item found in a space. It should include methods to get item details such as damage and the space index.
public class Item {
private String name;
private int damage;
private int spaceIndex;
public Item(String name, int damage, int spaceIndex) {
this.name = name;
this.damage = damage;
this.spaceIndex = spaceIndex;
}
public int getDamage() {
return damage;
}
public int getSpaceIndex() {
return spaceIndex;
}
}
Implementing the Character Class
The Character class represents the target character in the game. It should include methods to move the character to a new space and reduce health.
public class Character {
private String name;
private int health;
private Space currentSpace;
public Character(String name, int health, Space currentSpace) {
this.name = name;
this.health = health;
this.currentSpace = currentSpace;
}
public void moveToSpace(Space space) {
this.currentSpace = space;
}
public void reduceHealth(int amount) {
this.health -= amount;
}
}
Step 4: Thorough Testing
Testing is a crucial step to ensure your design works as expected. Use your testing plan to write tests for each class and method. Tools like JUnit can help automate this process.
Unit Testing with JUnit
Unit tests verify the functionality of individual methods. Here’s an example of a unit test for the addSpace method in the World class.
@Test
public void testAddSpace() {
World world = new World("ExampleWorld", 10, 10);
Space space = new Space("Room1", new Point(0, 0), new Point(2, 2));
world.addSpace(space);
assertEquals(1, world.getSpaces().size());
}
Integration Testing
Integration tests verify that different parts of the system work together correctly. Here’s an example of an integration test to check if a character can move between spaces.
@Test
public void testCharacterMovement() {
World world = new World("ExampleWorld", 10, 10);
Space space1 = new Space("Room1", new Point(0, 0), new Point(2, 2));
Space space2 = new Space("Room2", new Point(3, 3), new Point(5, 5));
world.addSpace(space1);
world.addSpace(space2);
Character character = new Character("Target", 100, space1);
world.setTargetCharacter(character);
world.moveCharacter(space2);
assertEquals(space2, character.getCurrentSpace());
}
Edge Case Testing
Testing edge cases ensures your design handles unusual or extreme inputs robustly. For example, test what happens when a character tries to move to a non-existent space or when an item is added to a non-existent room.
@Test
public void testInvalidSpaceMovement() {
World world = new World("ExampleWorld", 10, 10);
Space space1 = new Space("Room1", new Point(0, 0), new Point(2, 2));
world.addSpace(space1);
Character character = new Character("Target", 100, space1);
world.setTargetCharacter(character);
Space invalidSpace = new Space("InvalidRoom", new Point(10, 10), new Point(12, 12));
world.moveCharacter(invalidSpace);
assertEquals(space1, character.getCurrentSpace()); // Character should not move
}
Step 5: Iterative Refinement
Design is an iterative process. As you implement your design, you may discover areas for improvement. Continuously refine your design and code based on feedback and new insights.
Incorporating Feedback
As you progress, seek feedback from peers, instructors, or mentors. Incorporate their suggestions to enhance your design and implementation.
Updating the Design Document
Keep an up-to-date design document reflecting any changes made during the implementation phase. This ensures that your design remains aligned with your code and provides a clear reference for future modifications.
Enhancing the Model
As you refine your model, consider adding additional features or improving existing ones. For example, you could enhance the getNeighbors method to handle more complex neighbor relationships or improve the getSpaceInfo method to provide more detailed information.
Conclusion
Building a board game model in Java involves understanding the requirements, designing the model, implementing the classes and methods, thoroughly testing the design, and iteratively refining the solution. By following this structured approach, you can manage the project effectively and develop a robust, flexible solution.