Building a Robust Airline Reservation System Assignment
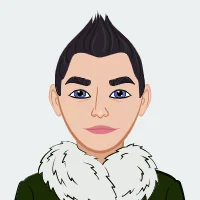
Creating a reservation system for an airline flight is an excellent way to practice and enhance your programming skills, especially if you’re seeking assistance from a Python assignment helper. Such a system involves managing complex data structures, handling various types of user input, and implementing logical processes that simulate real-world scenarios. By developing this system, you not only get to refine your coding abilities but also gain practical experience in problem-solving and system design. Whether you're working on the assignment yourself or using a Python assignment helper to guide you, this project will provide valuable insights into effective programming practices and system management. Here's a guide to help you develop a similar reservation system effectively and understand the key concepts involved.
Understanding the Core Components
Creating a reservation system involves understanding the system's main components and how they interact. This system should efficiently handle seat allocation, waiting lists, user interactions, and the overall workflow for adding and removing passengers. Let's delve into the essential elements of the system.
1. Structuring the Data
A well-structured data model is crucial for efficiently managing reservations and waiting lists. Here's how you can set up your data structures.
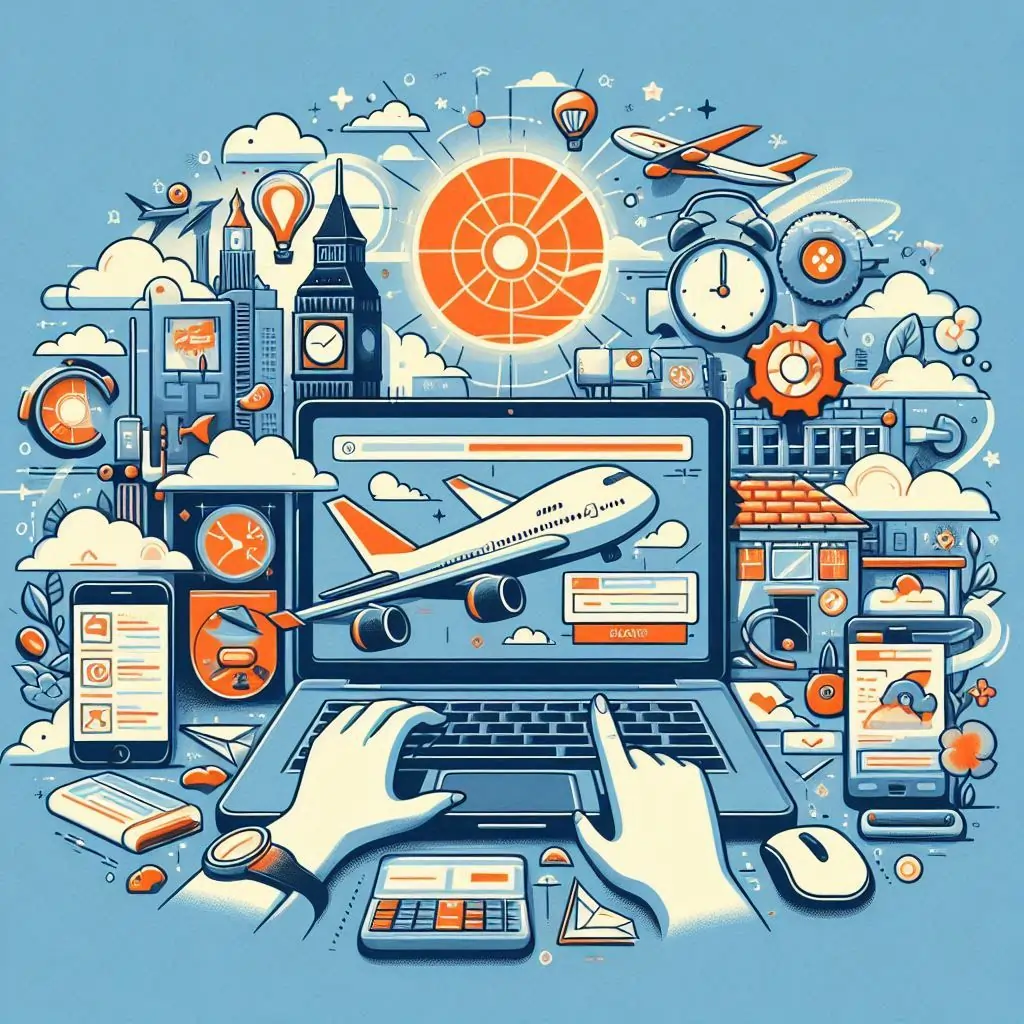
Defining the Seating Chart
The seating chart is a 2D array representing the airplane's seating arrangement. For this assignment, the airplane has 10 rows with 4 seats per row, resulting in a total of 40 seats. Each element in the array will store the passenger's name or indicate an available seat.
seating_chart = [["" for _ in range(4)] for _ in range(10)]
- Initialization: Start with all seats marked as empty. Use an empty string "" or a placeholder like "Available" to indicate open seats.
- Accessing Seats: Seats can be accessed using row and column indices, allowing easy retrieval and update of passenger information.
Managing the Waiting List
A waiting list is used to accommodate passengers when the flight is full. It should operate on a "first come, first served" basis, ensuring fair seat allocation as seats become available.
waiting_list = []
- Adding to the List: When a flight is full, add new passengers to the waiting list.
- Priority Handling: Ensure that passengers are given seats in the order they were added to the waiting list.
Passenger Information
Each passenger's information should include at least their name and their assigned seat (if applicable). This information can be stored in a dictionary or as part of the seating chart structure.
passenger_info = {
"name": "John Doe",
"seat": "1A"
}
2. Implementing Core Features
Once the data structures are set, you can begin implementing the core features of the reservation system. These features handle adding and removing passengers, displaying passenger lists, and managing the waiting list.
Adding a Passenger
The process of adding a passenger involves checking seat availability, assigning a seat, and updating the waiting list if necessary.
Checking Seat Availability
Before assigning a seat, check if any seats are available. This involves iterating through the seating chart and looking for empty seats.
def find_available_seat(seating_chart):
for row_index, row in enumerate(seating_chart):
for seat_index, seat in enumerate(row):
if seat == "":
return row_index, seat_index
return None
- Iteration: Loop through each seat in the seating chart to find an open spot.
- Return Value: Return the row and seat indices of the first available seat or None if no seats are available.
Seat Assignment Process
Once an available seat is identified, the passenger can choose a specific seat or be assigned the first available one.
def assign_seat(seating_chart, passenger_name, preferred_seat=None):
if preferred_seat:
row, seat = preferred_seat
if seating_chart[row][seat] == "":
seating_chart[row][seat] = passenger_name
return True
available_seat = find_available_seat(seating_chart)
if available_seat:
row, seat = available_seat
seating_chart[row][seat] = passenger_name
return True
return False
- Preferred Seat: If a passenger requests a specific seat, check its availability and assign it if possible.
- Automatic Assignment: If no preference is specified, assign the first available seat.
Handling the Waiting List
When no seats are available, add the passenger to the waiting list to maintain order and fairness.
def add_to_waiting_list(waiting_list, passenger_name):
waiting_list.append(passenger_name)
- Appending: Add the passenger’s name to the end of the waiting list, maintaining a queue structure.
Removing a Passenger
Removing a passenger requires identifying their seat, clearing it, and updating the waiting list.
Identifying and Removing the Passenger
Search the seating chart for the passenger's name, then remove them by clearing the seat assignment.
def remove_passenger(seating_chart, passenger_name):
for row_index, row in enumerate(seating_chart):
for seat_index, seat in enumerate(row):
if seat == passenger_name:
seating_chart[row][seat_index] = ""
return row_index, seat_index
return None
- Search: Iterate through the seating chart to find and clear the passenger's seat.
- Return Indices: Provide the cleared seat's indices for potential reassignment.
Updating the Waiting List
After a seat is vacated, check the waiting list for passengers to move to the seat.
def assign_seat_from_waiting_list(seating_chart, waiting_list):
if waiting_list:
passenger_name = waiting_list.pop(0)
assign_seat(seating_chart, passenger_name)
- Pop from List: Remove the first passenger from the waiting list and assign them the newly available seat.
- Maintain Order: Ensure the waiting list continues to operate on a first-come, first-served basis.
3. Displaying Passenger Information
Providing a clear display of passenger information enhances the user experience and simplifies management.
Displaying the Seating Chart
Create a function to visualize the seating chart, showing occupied and available seats.
def display_seating_chart(seating_chart):
for row_index, row in enumerate(seating_chart):
print(f"Row {row_index + 1}: ", end="")
for seat in row:
print(f"{seat if seat else 'Available'} ", end="")
print()
- Formatting: Ensure the seating chart is easy to read, with clear distinctions between occupied and available seats.
- Row Labels: Label each row for easy reference.
Listing Passengers and Waiting List
Provide an option to list all current passengers and those on the waiting list.
def list_passengers(seating_chart, waiting_list):
print("Passengers on Flight:")
for row_index, row in enumerate(seating_chart):
for seat_index, seat in enumerate(row):
if seat:
print(f"Seat {row_index + 1}{chr(seat_index + 65)}: {seat}")
print("\nWaiting List:")
for i, passenger in enumerate(waiting_list):
print(f"{i + 1}: {passenger}")
- Alphabetic Seat Labels: Use letters to label seats within rows (e.g., 1A, 1B).
- Clear Listing: Distinguish between passengers on the flight and those waiting.
Enhancing User Interaction
Ensure users can interact with the system through a straightforward interface.
def main_menu():
print("Welcome to the Airline Reservation System")
print("1. Add Passenger")
print("2. Remove Passenger")
print("3. List Passengers")
print("4. Reset Flight")
print("5. Exit")
- Menu Options: Provide users with a menu to navigate the system easily.
- Prompt for Input: Request input for each option, handling different commands appropriately.
Enhancing System Functionality
To make the reservation system more robust and user-friendly, consider additional features and improvements that can extend its capabilities.
1. Automating Passenger Generation
To test the system effectively, implement a feature that automatically generates passengers.
Command-Line Argument Handling
Use command-line arguments to specify the number of passengers to generate when the program starts.
import sys
def auto_generate_passengers(seating_chart, waiting_list, num_passengers):
for i in range(1, num_passengers + 1):
passenger_name = f"Pass{i}"
if not assign_seat(seating_chart, passenger_name):
add_to_waiting_list(waiting_list, passenger_name)
if __name__ == "__main__":
if len(sys.argv) > 1:
num_passengers = int(sys.argv[1])
auto_generate_passengers(seating_chart, waiting_list, num_passengers)
- Batch Files: Create a batch file to test the program with different numbers of passengers automatically.
- Efficiency: Ensure the system efficiently handles large numbers of passengers during testing.
Testing Scenarios
Use auto-generation to test edge cases, such as when the plane is full or nearly full, to ensure robustness.
# Batch File Example
@echo off
echo Close this cmd window after program starts.
cmd /c "python project5.py 40"
- Stress Testing: Simulate high passenger volumes to evaluate system performance under load.
- Boundary Testing: Examine how the system manages transitions between full and partially filled states.
2. Resetting the Flight
Implement a feature to clear all data and start a new flight, allowing the system to be reused efficiently.
Clearing Data Structures
Reset the seating chart and waiting list to their initial states.
def reset_flight(seating_chart, waiting_list):
for row in seating_chart:
for seat_index in range(len(row)):
row[seat_index] = ""
waiting_list.clear()
print("Flight has been reset.")
- Reinitialization: Ensure all seats are marked as available and the waiting list is empty.
- User Notification: Inform users that the flight has been reset to confirm the action.
User Interaction
Incorporate the reset feature into the main menu for easy access.
def main():
while True:
main_menu()
choice = input("Enter your choice: ")
if choice == "1":
# Add Passenger Logic
pass
elif choice == "2":
# Remove Passenger Logic
pass
elif choice == "3":
list_passengers(seating_chart, waiting_list)
elif choice == "4":
reset_flight(seating_chart, waiting_list)
elif choice == "5":
break
else:
print("Invalid choice. Please try again.")
- Integration: Ensure the reset feature is seamlessly integrated with other menu options.
- Exit Option: Allow users to exit the program gracefully.
3. Improving User Experience
Enhancing user experience is crucial for making the system intuitive and efficient.
Error Handling
Implement robust error handling to manage invalid inputs and unexpected scenarios.
def safe_input(prompt, valid_choices):
while True:
choice = input(prompt)
if choice in valid_choices:
return choice
print("Invalid choice. Please try again.")
- Input Validation: Ensure all user inputs are checked and validated before processing.
- Graceful Failure: Provide meaningful error messages and recovery options.
Advanced Display Options
Consider adding features like print preview, font change, or export options for the seating chart.
def print_seating_chart(seating_chart):
# Implement printing or exporting functionality
Pass
- Customization: Allow users to customize how the seating chart is displayed or printed.
- Advanced Features: Implement additional options for users who need more detailed output.
Conclusion
Creating an airline reservation system is a valuable exercise in programming, offering insights into data management, user interaction, and system design. By following this comprehensive guide, you can develop a robust system that not only meets the given requirements but also enhances your programming skills. Remember, the key to success lies in breaking down the problem, systematically addressing each component, and iterating through testing and improvement. With this approach, you'll be well-equipped to tackle similar programming assignment and real-world projects.