Simplifying Complex Assignments: Data Structures and File Management
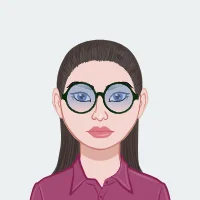
Programming assignments, particularly those involving data structures and file handling, can be daunting. These tasks often require an understanding of complex concepts and the ability to implement them effectively. This guide aims to help students approach and solve programming assignments by breaking down the process into manageable steps. Whether you're working on a linked list, hash table, or handling data on disk, these techniques will provide a solid foundation. By the end of this blog, you should be equipped with the knowledge to handle similar data structure assignments confidently, improving your coding skills and understanding of key concepts essential for mastering any data structure assignment.
Understanding the Problem
Programming assignments dealing with two-dimensional data (x, y) often require you to store, retrieve, and manage these points efficiently. This section will help you understand the key aspects of such assignments, which include:
- Data Entry: How to insert data points into the structure.
- Data Search: How to efficiently search for a specific data point.
- Memory Management: How to handle data in central memory.
- Disk Management: How to handle data stored on disk, ensuring efficient access and modifications.
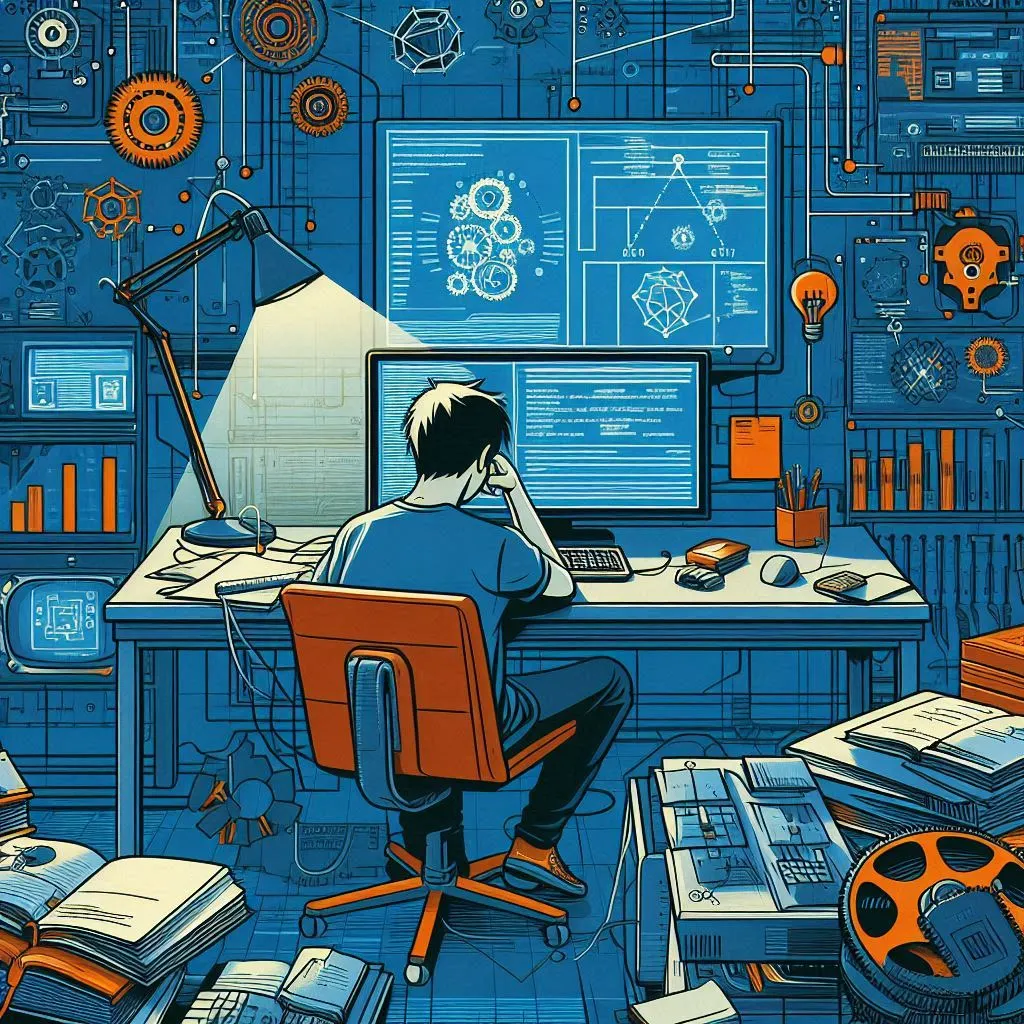
By understanding these core components, you can break down the assignment into smaller, more manageable parts and tackle each one systematically.
A. Central Memory Processing
Central memory processing involves handling data structures that reside in the main memory. In this section, we'll explore linked lists and the fragmentation method, two common data structures used in such assignments.
1. Linked Lists
A linked list is a fundamental data structure that stores elements sequentially. Each element, or node, contains data and a reference (or link) to the next node in the sequence. Here's how you can implement linked lists for this type of assignment:
Data Structure
Each node in the linked list will store an (x, y) pair. You'll need a class to represent a node and another to represent the linked list.
class Node:
def __init__(self, x, y):
self.data = (x, y)
self.next = None
class LinkedList:
def __init__(self):
self.head = None
self.tail = None
Insertion
To insert a new (x, y) pair into the linked list, you'll create a method that adds the new node to the end of the list. Keeping track of both the head and the tail of the list optimizes insertion time.
def insert(self, x, y):
new_node = Node(x, y)
if self.tail:
self.tail.next = new_node
else:
self.head = new_node
self.tail = new_node
Search
Implementing a search method involves traversing the linked list and comparing each node's data with the target (x, y) pair. You'll also keep track of the number of comparisons made during the search.
def search(self, x, y):
current = self.head
comparisons = 0
while current:
comparisons += 1
if current.data == (x, y):
return True, comparisons
current = current.next
return False, comparisons
2. Fragmentation Method
The fragmentation method combines a hash table with linked lists to manage data more efficiently. This approach involves calculating the position in the hash table using a hash function and storing linked lists at each position.
Hash Function
Calculate the position in the hash table using the formula H(x, y) = (x * N + y) % M. This function distributes the data points evenly across the table.
Data Structure
Use an array (hash table) where each entry points to a linked list.
class HashTable:
def __init__(self, M, N):
self.table = [LinkedList() for _ in range(M)]
self.M = M
self.N = N
Insertion
Insert the (x, y) pair into the linked list at the appropriate index determined by the hash function.
def hash_function(self, x, y):
return (x * self.N + y) % self.M
def insert(self, x, y):
index = self.hash_function(x, y)
self.table[index].insert(x, y)
Search
Traverse the linked list at the calculated index to find the (x, y) pair.
def search(self, x, y):
index = self.hash_function(x, y)
return self.table[index].search(x, y)
B. Disk Processing
Handling data structures on disk involves managing data storage in binary format and using disk pages. This section covers linked lists and the fragmentation method on disk, including methods for insertion and search.
1. Linked Lists on Disk
Handling linked lists on disk requires managing data storage in binary format and using disk pages.
Disk Pages
Define the size of a disk page (e.g., 256 bytes) and manage data using buffers. This approach helps in efficiently reading and writing data to disk.
Insertion
To insert a new (x, y) pair, read the last page from disk, insert the (x, y) pair, and write back to disk. If the page is full, create a new page.
def insert_to_disk(file, x, y):
def insert_to_disk(file, x, y):
# Implementing disk operations here
Pass
Search
Sequentially read pages from disk into memory, searching for the (x, y) pair. This involves reading pages one by one until the target is found.
def search_on_disk(file, x, y):
# Implementing disk search operations here
Pass
2. Fragmentation Method on Disk
The fragmentation method on disk extends the in-memory fragmentation method to disk storage. This involves managing page chains and handling overflow pages.
Data Structure
Maintain a table in memory pointing to pages on disk. Each table entry points to the first and last page of the chain for that position.
class DiskHashTable:
def __init__(self, M, N):
self.table = [None] * M
self.M = M
self.N = N
Insertion
Read the last page of the chain from disk, insert the (x, y) pair, and manage overflow pages if necessary.
def insert_to_disk_table(self, file, x, y):
# Implementing disk insertion operations here
Pass
Search
Sequentially read pages in the chain from disk into memory, searching for the (x, y) pair. This involves reading each page until the target is found.
def search_on_disk_table(self, file, x, y):
# Implementing disk search operations here
Pass
C. Performance Comparison
To evaluate the performance of these methods, you need to conduct experiments by inserting varying amounts of data and measuring the average number of comparisons or disk accesses required for searches. Plotting these results will help visualize the efficiency of each method under different conditions.
Methodology
- Data Sets: Use data sets of varying sizes (e.g., 1,000, 10,000, 30,000, 50,000, 70,000, 100,000).
- Search Operations: Perform 100 searches for data points that are known to exist in the structure.
- Comparisons and Disk Accesses: Track the number of comparisons for in-memory structures and the number of disk accesses for disk-based structures.
In-Memory Structures
For in-memory structures (linked lists and fragmentation method), measure the average number of comparisons required for successful and unsuccessful searches.
def evaluate_disk_based_structures():
# Implementing evaluation logic for disk-based structures
Pass
Disk-Based Structures
For disk-based structures (linked lists and fragmentation method on disk), measure the average number of disk accesses required for successful and unsuccessful searches.
def evaluate_disk_based_structures():
# Implementing evaluation logic for disk-based structures
Pass
Results and Analysis
Plot the results to compare the performance of each method. Create graphs showing the average number of comparisons or disk accesses as a function of the data set size (M) for both successful and unsuccessful searches.
def plot_results():
# Implementing plotting logic
Pass
D: Conclusion
Understanding and implementing various data structures and file handling techniques is crucial for solving programming assignments involving complex data management. By breaking down the problem and systematically addressing each part, you can develop efficient solutions and improve your programming skills.
Key Takeaways
- Linked Lists: Suitable for simple sequential data storage and retrieval.
- Fragmentation Method: Combines hash tables and linked lists for more efficient data management.
- Disk Management: Essential for handling large data sets that cannot fit entirely in memory.
Conclusion
Document your process and results to highlight the performance of different methods. This practice not only helps in understanding the efficiency of each approach but also serves as a valuable reference for future assignments.