Comprehensive Strategies for Tackling Hash Table Assignments
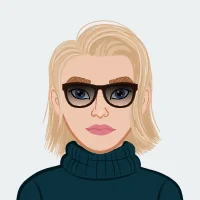
Hash tables are a cornerstone in the field of computer science, widely used to implement associative arrays or mappings of key-value pairs. These data structures offer efficient methods for data retrieval, which makes them essential for various applications. For students tackling programming assignments, mastering hash tables is crucial. This comprehensive guide will walk you through the process of creating a hash table, helping you understand the underlying principles and techniques that you can apply to any similar assignment. This blog will provide a step-by-step guide to help students approach and solve data structure assignments effectively.
Understanding Hash Tables
Before diving into the implementation, it’s important to grasp what a hash table is and how it functions. This understanding forms the foundation for effectively solving assignments related to hash tables.
What is a Hash Table?
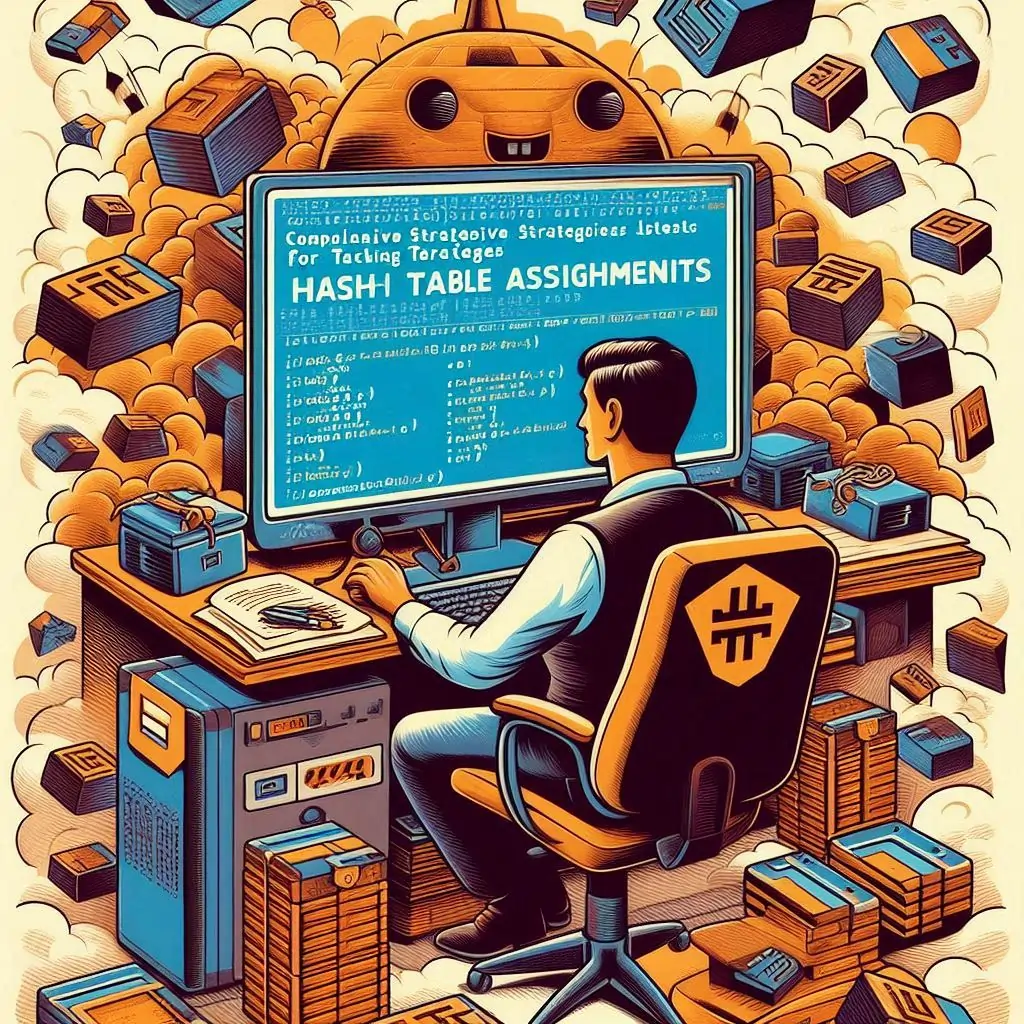
A hash table is a data structure that stores data in an array-like format. Each data value is associated with a unique key, and the position of this key-value pair in the array is determined by a hash function. This function takes the key as input and produces an index within the array, where the corresponding value will be stored. The efficiency of a hash table lies in its ability to provide quick data retrieval, insertion, and deletion operations, typically in constant time, O(1).
Hash Function
A hash function is crucial for the operation of a hash table. It converts a key into an array index, ensuring that the data is distributed uniformly across the array. A good hash function minimizes collisions, which occur when two keys hash to the same index. For string keys, a common hash function is based on the formula:
s0⋅31(n−1)+s1⋅31(n−2)+...+sn−1s0 \cdot 31^{(n-1)} + s1 \cdot 31^{(n-2)} + ... + sn-1s0⋅31(n−1)+s1⋅31(n−2)+...+sn−1
where sisisi is the ith character of the input, and nnn is the length of the input string.
Collision Handling
Even with a good hash function, collisions are inevitable. There are several strategies to handle collisions, with chaining being one of the most common methods. In chaining, each array index points to a list of key-value pairs. If multiple keys hash to the same index, they are stored in the same list. This approach ensures that collisions do not degrade the performance of the hash table significantly.
Creating a Hash Table Class
Implementing a hash table involves several steps, from defining the class to implementing the methods for various operations. Let’s break down this process step by step.
Defining the Hash Table Class
Start by defining a class for the hash table. This class will encapsulate all the methods required to manipulate the hash table, such as adding, removing, and retrieving items. Additionally, it will have methods to clear the table and get the size and number of values in the table.
class HashTable:
def __init__(self, size):
self.size = size
self.table = [[] for _ in range(size)]
self.num_items = 0
self.collisions = 0
In this class definition, the __init__ method initializes the hash table with a specified size. It creates an array of empty lists (to handle collisions using chaining), initializes the number of items to 0, and sets the collision count to 0.
Implementing the Hash Function
The hash function is responsible for converting keys into array indices. For strings, the hash function discussed earlier can be implemented as follows:
def hash_code(self, key):
hash_value = 0
for i, char in enumerate(key):
hash_value += ord(char) * (31 ** (len(key) - 1 - i))
return hash_value % self.size
This method calculates the hash value for a given key by iterating over each character, computing its contribution to the hash value, and then taking the modulus with the table size to ensure the index is within bounds.
Adding Items to the Hash Table
The add method inserts a key-value pair into the hash table. It first computes the index using the hash function and then checks if the key already exists at that index. If the key is a duplicate, it returns False. Otherwise, it adds the key-value pair to the list at the computed index, updates the number of items, and increments the collision count if necessary.
def add(self, key, value):
index = self.hash_code(key)
for kv in self.table[index]:
if kv[0] == key:
return False
self.table[index].append((key, value))
self.num_items += 1
if len(self.table[index]) > 1:
self.collisions += 1
return True
Removing and Retrieving Items
The remove and retrieve methods handle the removal and retrieval of key-value pairs, respectively. They traverse the list at the computed index to find the key and perform the necessary operation.
def remove(self, key):
index = self.hash_code(key)
for i, kv in enumerate(self.table[index]):
if kv[0] == key:
self.table[index].pop(i)
self.num_items -= 1
return kv[1]
return None
def retrieve(self, key):
index = self.hash_code(key)
for kv in self.table[index]:
if kv[0] == key:
return kv[1]
return None
Additional Methods
Implement methods to clear the table, get the number of collisions, get the size of the table, and get the number of items.
def clear(self):
self.table = [[] for _ in range(self.size)]
self.num_items = 0
self.collisions = 0
def get_collisions(self):
return self.collisions
def get_size(self):
return self.size
def get_num_items(self):
return self.num_items
Testing Your Hash Table
Testing is a critical part of the development process. Create a test suite in your main class to insert randomly generated string values into your hash table and display the number of collisions and the size of the table.
import random
import string
def random_string(length):
letters = string.ascii_letters
return ''.join(random.choice(letters) for i in range(length))
def main():
ht = HashTable(10000)
for _ in range(20000):
key = random_string(random.randint(4, 20))
ht.add(key, random_string(10))
print("Number of collisions:", ht.get_collisions())
print("Size of hash table:", ht.get_size())
if __name__ == "__main__":
main()
Advanced Techniques for Optimizing Hash Tables
Once you have the basic hash table implementation, you can explore advanced techniques to optimize its performance and functionality.
Dynamic Resizing
A static hash table size may lead to inefficiencies as the number of items grows. Implementing dynamic resizing, where the hash table size is increased when the load factor (number of items divided by table size) exceeds a certain threshold, can maintain efficient operations.
Load Balancing
Load balancing involves redistributing items in the hash table to ensure even distribution across the array. This can be achieved by rehashing, which involves recalculating the hash values of all items when the table size changes.
Alternative Collision Handling
While chaining is a common method, other techniques like open addressing (e.g., linear probing, quadratic probing) can also be effective. These methods store all elements within the array itself, eliminating the need for additional data structures like lists.
Real-World Applications of Hash Tables
Hash tables are not just academic exercises; they have numerous real-world applications that highlight their importance.
Databases
Hash tables are used in database indexing to quickly locate records. They help implement hash indexes, which can significantly speed up query performance by reducing the number of disk accesses required.
Caches
Caches use hash tables to store frequently accessed data, allowing for rapid retrieval. This application is crucial in web browsers, operating systems, and other systems that rely on quick data access.
Symbol Tables
Compilers and interpreters use hash tables to implement symbol tables, which store information about variable names, function names, and other identifiers. This allows for efficient symbol lookup during code compilation or interpretation.
Conclusion
Creating a hash table involves understanding hash functions, collision handling, and implementing key operations. By breaking down the task into manageable steps and focusing on each part of the process, students can effectively complete their programming assignments. Practice and familiarity with these concepts will lead to greater confidence and proficiency in handling data structures in programming.
Remember, the key to mastering hash tables lies in consistent practice and a clear understanding of the underlying principles.