Effortless 3D Graphics in Java: JOGL Hacks for Flawless Assignments
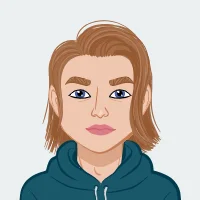
Creating 3D graphics scenes in Java using OpenGL can be an exciting and rewarding experience. Whether you are working on a class project or exploring the field of computer graphics, understanding the fundamentals and techniques involved is essential. This comprehensive guide will help you to complete your programming assignments by breaking down the process into manageable steps, ensuring you have the skills and knowledge to tackle any 3D graphics task using Java and OpenGL. If you need help with java assignment, this guide will provide you with the foundational knowledge and practical tips to succeed.
Setting Up Your Development Environment
Before diving into the coding aspect, it's crucial to set up a robust development environment. This ensures that you have all the necessary tools and libraries to create and test your 3D graphics scenes effectively.
Installing the IDE
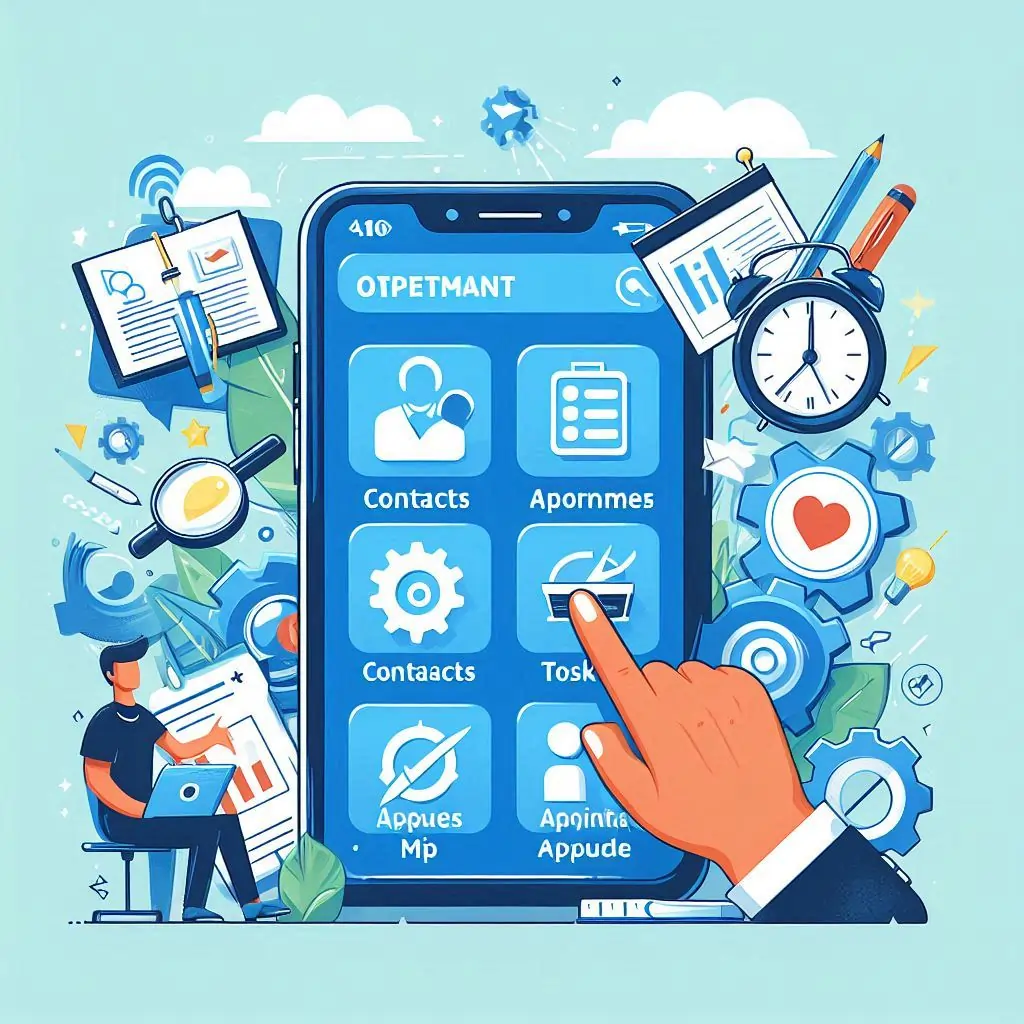
The first step is to choose and install an Integrated Development Environment (IDE) that supports Java development. Two popular options are NetBeans and Eclipse.
NetBeans
NetBeans is a versatile and user-friendly IDE that supports various programming languages, including Java. Here’s how to get started with NetBeans:
- Download NetBeans: Visit the official NetBeans website and download the latest version suitable for your operating system.
- Install NetBeans: Follow the installation instructions provided on the website. The installation process is straightforward and should take only a few minutes.
- Set Up Your Project: Once installed, open NetBeans and create a new Java project. This will be the foundation for your JOGL application.
Eclipse
Eclipse is another powerful IDE widely used for Java development. Here’s how to set up Eclipse:
- Download Eclipse: Go to the Eclipse official website and download the Eclipse IDE for Java Developers.
- Install Eclipse: Run the installer and follow the on-screen instructions to complete the installation.
- Create a New Project: Launch Eclipse and create a new Java project. This will serve as the starting point for your 3D graphics application.
Configuring JOGL
JOGL (Java Binding for the OpenGL API) is essential for using OpenGL in Java applications. To configure JOGL in your IDE, follow these steps:
- Download JOGL: Obtain the JOGL library from the official JOGL website. Ensure you download the correct version for your operating system.
- Add JOGL to Your Project: In your IDE, add the JOGL JAR files to your project’s build path. This process may vary slightly between NetBeans and Eclipse, but the goal is to include the necessary JOGL libraries in your project.
- Verify Configuration: To ensure JOGL is correctly configured, create a simple OpenGL application that initializes a GLCanvas and renders a basic shape. If the shape renders successfully, your setup is complete.
Understanding the Requirements
Assignments involving 3D graphics typically come with specific requirements and deliverables. Understanding these requirements is crucial for successful project completion.
Scene Specifications
The 3D scene you create must meet certain specifications, such as resolution, shapes, and transformation methods.
Resolution
The scene should have a minimum resolution of 640x480 pixels. This ensures that the rendered graphics are of adequate size and clarity.
Shapes
Include at least six different shapes in your scene. Common shapes to consider are cubes, spheres, pyramids, cones, cylinders, and toruses. These shapes will demonstrate your ability to work with various geometric forms.
Transformation Methods
Use at least six different transformation methods to manipulate the shapes. Transformations include translation, rotation, scaling, shearing, reflection, and perspective projection. Applying these transformations effectively showcases your understanding of 3D space manipulation.
Programming Language and Libraries
You are required to use Java and JOGL for implementing OpenGL. This combination leverages Java’s object-oriented capabilities and JOGL’s powerful graphics rendering functions.
Code Style
Adhering to a coding style guide, such as the Google Java Style Guide, ensures your code is consistent, readable, and maintainable. Key aspects of this guide include proper indentation, naming conventions, and commenting practices.
Documentation and Testing
Creating thorough documentation and a comprehensive test plan is essential for verifying your application’s functionality and quality.
Test Plan
A test plan outlines the methods you will test, the testing procedures, and the expected results. This helps ensure that all components of your application work as intended.
Documentation
Documenting your project involves capturing screenshots of your 3D scene, providing detailed descriptions, and organizing the information in a clear and professional manner. Include a title page, captions for screenshots, and references formatted in APA style.
Developing the 3D Scene
Now that your environment is set up and you understand the requirements, it’s time to develop your 3D scene.
Creating Shapes
Start by defining the shapes you will include in your scene. This involves writing Java code to specify the vertices and faces of each shape.
Defining a Cube
A cube is a basic 3D shape with six faces, each a square. Here’s how to define a cube in JOGL:
import com.jogamp.opengl.GL2;
public class Shapes {
public void drawCube(GL2 gl) {
gl.glBegin(GL2.GL_QUADS);
// Define vertices for a cube
gl.glVertex3f(1.0f, 1.0f, -1.0f);
gl.glVertex3f(-1.0f, 1.0f, -1.0f);
gl.glVertex3f(-1.0f, -1.0f, -1.0f);
gl.glVertex3f(1.0f, -1.0f, -1.0f);
gl.glVertex3f(1.0f, -1.0f, 1.0f);
gl.glVertex3f(-1.0f, -1.0f, 1.0f);
gl.glVertex3f(-1.0f, 1.0f, 1.0f);
gl.glVertex3f(1.0f, 1.0f, 1.0f);
gl.glEnd();
}
// Add methods for other shapes (e.g., drawSphere, drawPyramid)
}
Defining a Sphere
A sphere is more complex due to its curved surface. You can approximate a sphere by defining multiple triangles:
public void drawSphere(GL2 gl) {
GLUT glut = new GLUT();
glut.glutSolidSphere(1.0, 36, 18);
}
Defining a Pyramid
A pyramid has a polygonal base and triangular faces converging to a point:
public void drawPyramid(GL2 gl) {
gl.glBegin(GL2.GL_TRIANGLES);
// Define vertices for a pyramid
gl.glVertex3f(0.0f, 1.0f, 0.0f);
gl.glVertex3f(-1.0f, -1.0f, 1.0f);
gl.glVertex3f(1.0f, -1.0f, 1.0f);
// ... Add the rest of the faces
gl.glEnd();
}
Applying Transformations
Transformation methods manipulate the position, orientation, and size of shapes within the 3D space.
Translation
Translation moves a shape from one location to another. Here’s how to apply translation in JOGL:
gl.glTranslatef(1.0f, 0.0f, -6.0f); // Move the shape along the x, y, and z axes
Rotation
Rotation changes the orientation of a shape around a specified axis:
gl.glRotatef(45.0f, 1.0f, 1.0f, 0.0f); // Rotate 45 degrees around the x, y, and z axes
Scaling
Scaling alters the size of a shape:
gl.glScalef(0.5f, 0.5f, 0.5f); // Reduce the size of the shape to half
Implementing Additional Transformations
To meet the requirement of using at least six different transformation methods, consider adding the following transformations:
Shearing
Shearing skews the shape along the x or y axis:
gl.glMatrixMode(GL2.GL_MODELVIEW);
gl.glLoadIdentity();
gl.glMultMatrixf(new float[]{
1, 0.5f, 0, 0,
0, 1, 0, 0,
0, 0, 1, 0,
0, 0, 0, 1
}, 0);
Reflection
Reflection flips the shape across a plane, such as the x-y plane:
gl.glScalef(1.0f, -1.0f, 1.0f); // Reflect the shape across the x-y plane
Perspective Projection
Adjusting the perspective projection matrix can change how the scene is viewed, creating a sense of depth:
gl.glMatrixMode(GL2.GL_PROJECTION);
gl.glLoadIdentity();
glu.gluPerspective(45.0, aspect, 1.0, 20.0);
Adhering to the Style Guide
Following a coding style guide ensures that your code is clean, readable, and maintainable. The Google Java Style Guide is a popular choice, and here’s how you can apply its principles to your project.
Proper Indentation
Indentation makes your code visually clear and indicates the structure and hierarchy of your code blocks.
public void drawCube(GL2 gl) {
gl.glBegin(GL2.GL_QUADS);
// Correct indentation for nested code blocks
gl.glVertex3f(1.0f, 1.0f, -1.0f);
// Additional vertices with proper indentation
gl.glEnd();
}
Naming Conventions
Use descriptive and consistent names for variables, methods, and classes.
public class ShapeRenderer {
private GL2 gl;
public ShapeRenderer(GL2 gl) {
this.gl = gl;
}
public void renderCube() {
gl.glBegin(GL2.GL_QUADS);
// Naming variables appropriately
float vertex1 = 1.0f;
// Additional code
gl.glEnd();
}
}
Commenting
Effective comments explain the purpose and functionality of your code, making it easier to understand and maintain.
/**
* Draws a 3D cube using OpenGL.
*
* @param gl the GL2 object for rendering
*/
public void drawCube(GL2 gl) {
gl.glBegin(GL2.GL_QUADS);
// Define vertices for the front face of the cube
gl.glVertex3f(1.0f, 1.0f, -1.0f);
// Additional vertices and comments
gl.glEnd();
}
Testing and Documentation
A comprehensive test plan and thorough documentation are essential for ensuring your application works as expected and is easy to understand.
Preparing a Test Plan
A test plan outlines the methods to be tested, the testing procedures, and the expected results. This helps ensure that all components of your application function correctly.
Test Matrix
A test matrix is a table that lists each method tested, the testing procedure, and the results.
Method | TestDescription | ExpectedResult | ActualResult |
---|---|---|---|
drawCube() | Render a cube on the screen | Cube is rendered | Pass |
applyTransformations() | Apply transformations and render | Transformed shapes are rendered | Pass |
Capturing and Documenting Output
Documenting your project involves capturing screenshots of your 3D scene, providing detailed descriptions, and organizing the information in a clear and professional manner.
Taking Screen Captures
Use screen capture tools to take clear and labeled screenshots of your 3D scene at various stages. Ensure the images are high quality and adequately demonstrate the features and transformations applied to the shapes.
Writing Descriptions
Accompany each screenshot with a well-written description that explains what the image shows and how it relates to the overall project. Include details such as the shapes rendered, the transformations applied, and any challenges encountered during development.
Finalizing and Submitting Your Project
Once you have developed your 3D scene, tested your application, and documented your work, it’s time to finalize and submit your project.
Compiling Java Source Code
Ensure all Java source code is compiled and free of errors. Adhere to the Google Java Style Guide to maintain consistency and readability. Double-check that all methods and transformations are correctly implemented and that the code is well-commented.
Reviewing Documentation
Review your documentation to ensure it is complete, accurate, and well-organized. Make sure all screenshots are clear and appropriately labeled, and that descriptions provide sufficient detail to understand the project.
Preparing Deliverables
Prepare the final deliverables, which typically include:
- Java Source Code: All Java source code files used in the project, adhering to the Google Java Style Guide.
- Documentation: A Word or PDF file that includes the title page, introduction, setup and configuration details, implementation descriptions, test plan, screen captures, and references.
Submitting the Project
Submit the compiled Java source code and the documentation file as per the assignment instructions. Ensure that all files are correctly named and organized, making it easy for the instructor or evaluator to review your work. By following this structured approach, you can effectively tackle any 3D graphics assignment using Java and OpenGL. This guide provides a comprehensive framework that ensures you understand the requirements, develop the necessary skills, and produce high-quality work. Happy coding and best of luck with your 3D graphics projects!